An image is visual content that is displayed on a web page using specified URL. For example,
Styled Image
The above example shows a styled image using CSS.
Images are crucial in enhancing the visual appeal of a website, conveying information, and engaging users.
Add Image in HTML
An image is added in HTML using <img>
element.
<img src="house.png" alt="A building" />
Browser Output
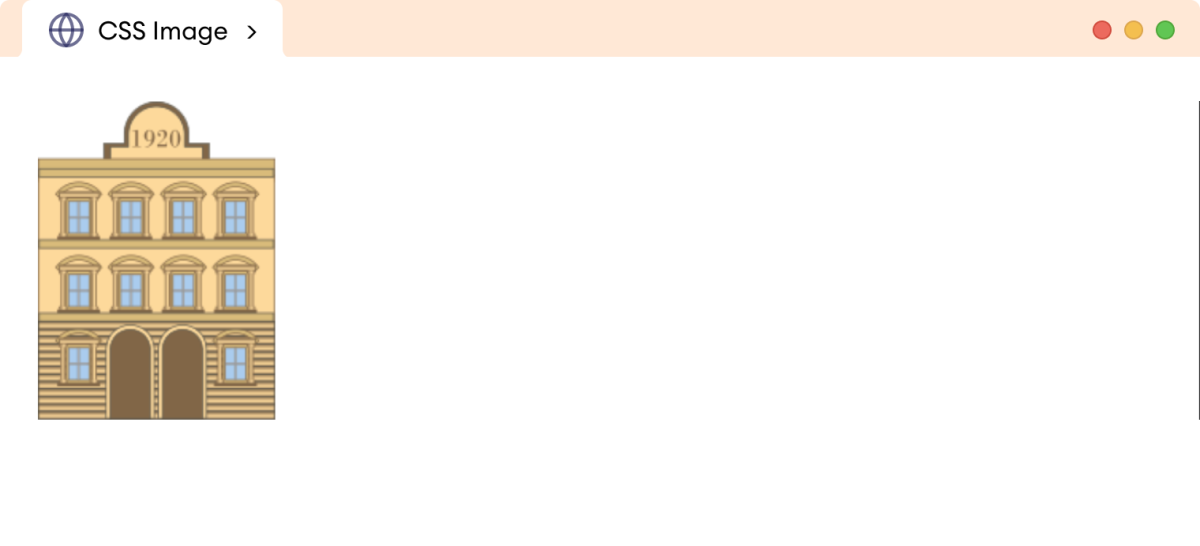
The above example shows the default image in HTML without any CSS styles.
Note: By default, images take their default width and height.
Style Your Image
We can use CSS to style our image in the following ways,
- Changing the image size
- Adding a rounded corner to the image
- Centering image
- Creating responsive image
- Creating thumbnail image
- Creating transparent image
- Adding a background image
- Creating image card
- Creating image text overlay
- Object-fit property
- Applying image filters
- Adding hover to the image
- Adding a flipping effect to the image
Let's look at each of them in detail.
Changing the Image Size
The width
and height
properties are used to resize
the image. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Size</title>
</head>
<body>
<h2>Original Image</h2>
<img src="https://www.programiz.com/blog/content/images/2020/11/intro-c-banner-1-1.png" alt="Avatar of a standing girl" />
<div class="styled-image">
<h2>After setting up width and height properties</h2>
<img src="https://www.programiz.com/blog/content/images/2020/11/intro-c-banner-1-1.png" alt="Avatar of a standing girl" />
</div>
</body>
</html>
.styled-image img {
width: 280px;
height: 200px;
}
Browser Output
Original Image
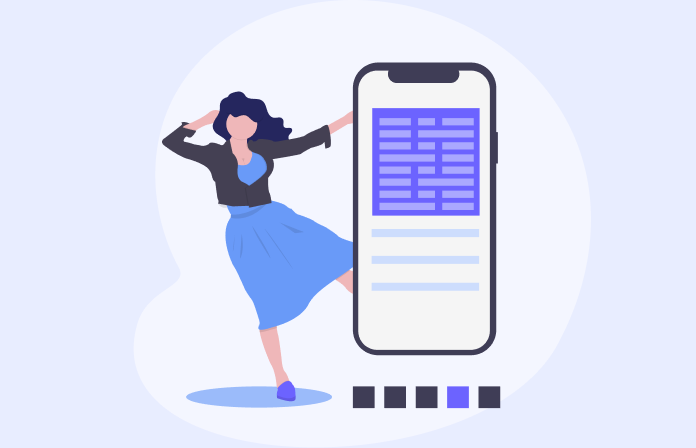
After setting up width and height properties
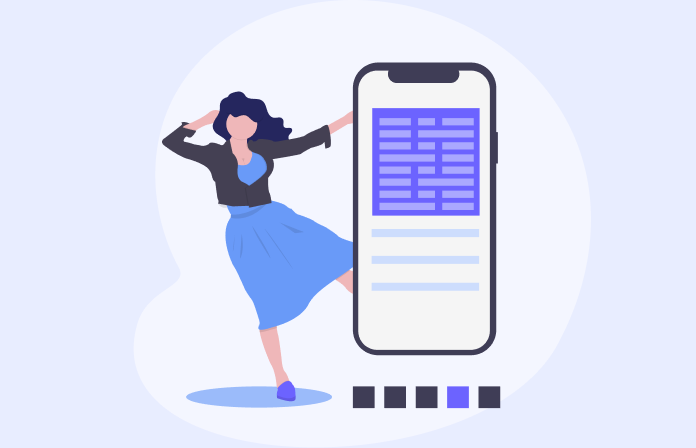
The above example shows the default image and the image with a fixed width
and height of 280px
and 200px
, respectively.
Note: Adjusting the width
and
height
properties of an image can change the proportion of
its width to height. This is known as distorting the aspect ratio.
To maintain the original proportions of an image, use the max-width property to set an upper limit on width while allowing the height to adjust automatically.
Adding Rounded Corner to Image
The border-radius
property is used to add the rounded corners
around the image. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Styling</title>
</head>
<body>
<img src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="Building" />
</div>
</body>
</html>
img {
width: 200px;
height: 200px;
border-radius: 10px;
}
Browser Output
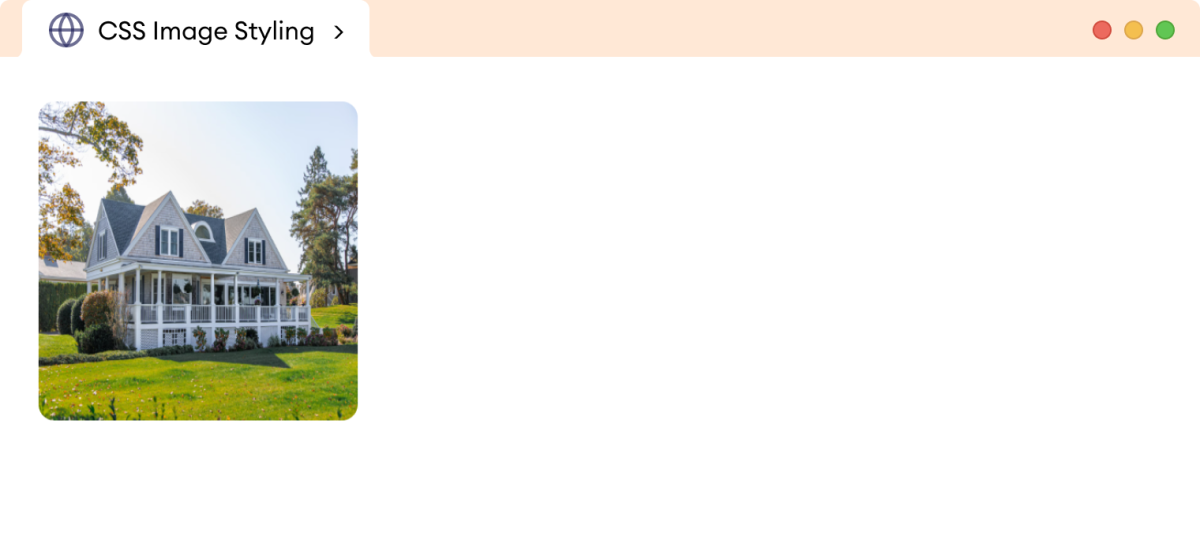
In the above example, the border-radius
property rounds the
corners of the image with a 10px
of border-radius.
We can make a circular image by adding:
border-radius: 50%;
Browser Output
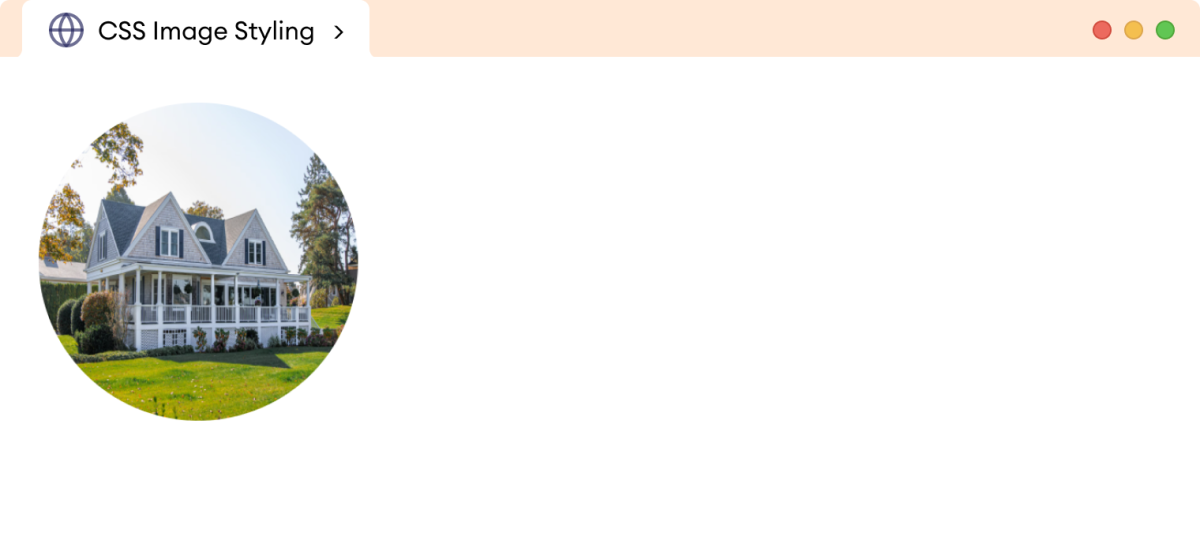
Centering Image
We can center an image in a container with the help of
display
and margin
properties. For example,
img {
width: 150px;
display: block;
margin-left: auto;
margin-right: auto;
}
Browser Output
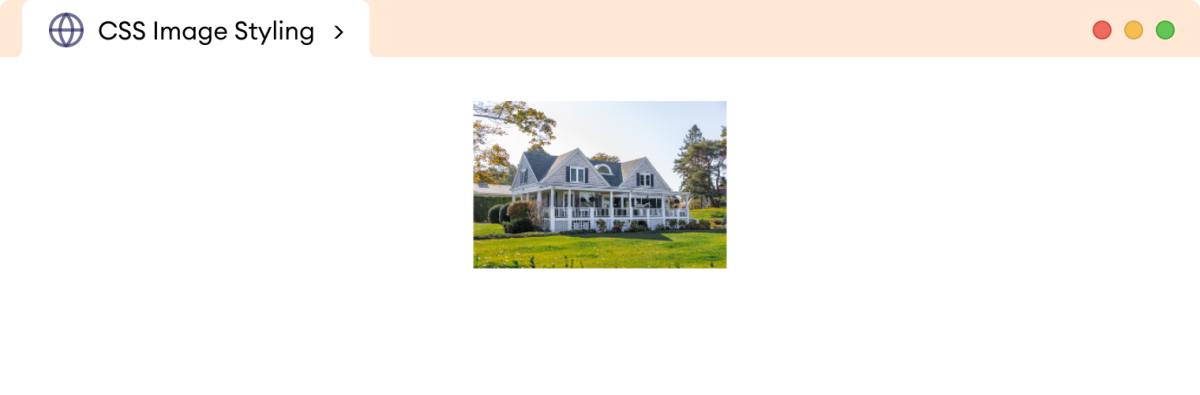
In the above example,
-
display: block
converts the image into a block-level element -
margin-left: auto
andmargin-right: auto
center an image horizontally by creating an equal space around it
Note: Block-level elements begin on a new line and take the full available width of the parent element.
Creating Responsive Image
The max-width
property is used to make the image responsive.
For example,
img {
max-width: 500px;
height: auto;
}
Browser Output
Here,
-
max-width: 500px
sets the maximum width of500px
to the image height: auto
maintains the image's aspect ratio
Note: It is important to note that the
max-width
property will only scale down an image. If the image
is smaller than its container, it will not scale up to fill the container.
Creating Thumbnail Image
The border
property is used to create a thumbnail image. For
example,
img {
max-width: 150px;
border: 1px solid black;
padding: 10px;
}
Browser Output
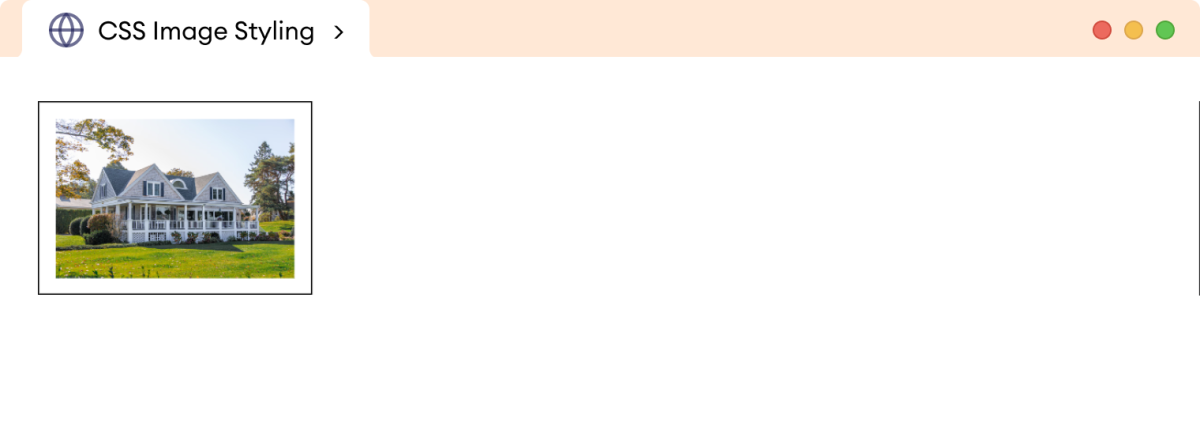
Here, the border
property adds a solid
black
border of 1px
around the image, creating an
appearance like a thumbnail.
Creating Transparent Image
The opacity
property adds transparency to an image.
The value of the opacity
property ranges from
0.0
to 1.0
. The lower value represents the more
transparent image.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Transparent Image</title>
</head>
<body>
<div>
<p>Opacity-0.1</p>
<img
class="first-image"
src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="Building" />
</div>
<div>
<p>Opacity-0.5</p>
<img
class="second-image"
src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="Building" />
</div>
<div>
<p>Opacity-1</p>
<img
class="third-image"
src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="Building" />
</div>
</body>
</html>
img {
max-width: 150px;
}
.first-image {
opacity: 0.1;
}
.second-image {
opacity: 0.5;
}
.third-image {
opacity: 1;
}
div {
display: inline-block;
margin-right: 20px;
}
Browser Output
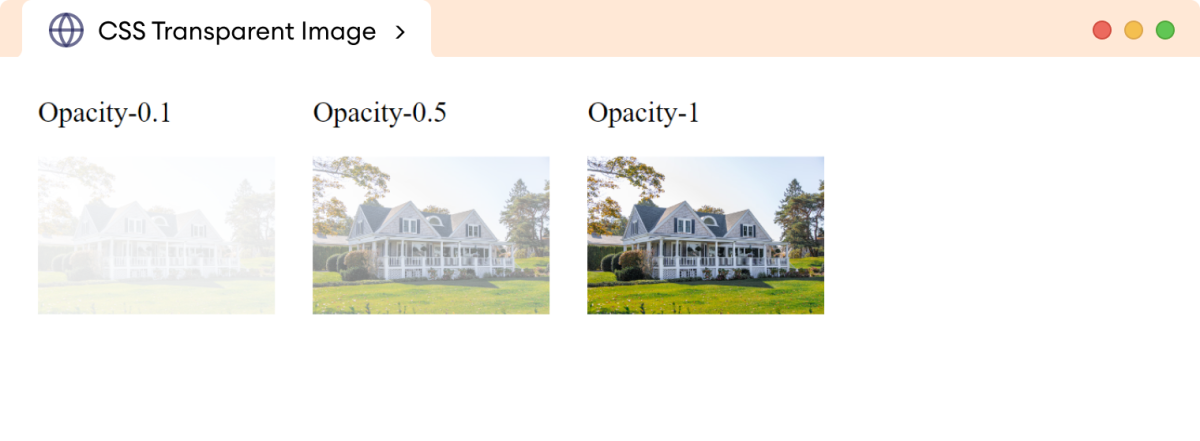
The above example shows images with different opacity values.
Adding a Background Image
We can add an image to use as a background of an element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS image</title>
</head>
<body>
<h2>Adding a background image</h2>
</body>
</html>
body {
background-image: url("https://images.unsplash.com/photo-1621091211034-53136cc1eb32?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1974&q=80");
}
Browser Output
Adding a background image
In the above example, the background-image
property adds the
background image to the body of the webpage.
Creating Image Card
Let's create an image card using different CSS properties.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Card</title>
</head>
<body>
<div class="image-card">
<img
src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="Image Description" />
<div class="card-content">
<p>Image Card</p>
</div>
</div>
</body>
</html>
.image-card {
max-width: 200px;
box-shadow: 0px 0px 20px rgba(0, 0, 0, 0.3);
border-radius: 8px;
overflow: hidden;
}
.image-card img {
width: 100%;
height: auto;
}
.card-content {
text-align: center;
}
Browser Output
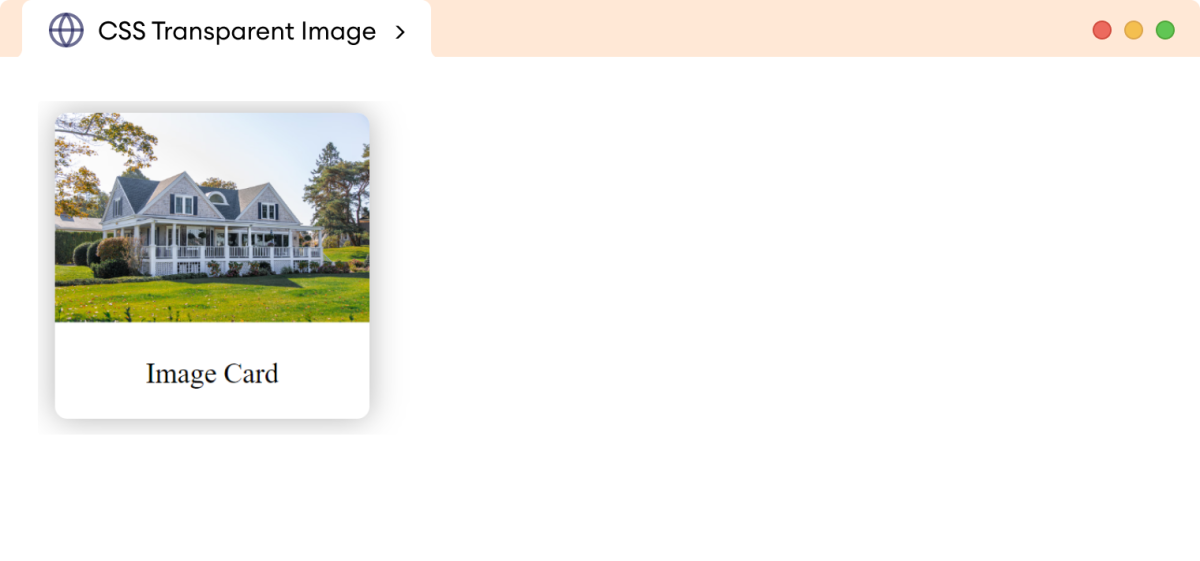
The above example creates a simple image card.
Creating Image Text Overlay
We can place and position text in an image using different CSS properties. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Text</title>
</head>
<body>
<div class="container">
<img src="https://images.unsplash.com/photo-1444080748397-f442aa95c3e5?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1932&q=80"
alt="Building" />
<p class="top-left">Top Left</p>
<p class="top-right">Top Right</p>
<p class="bottom-left">Bottom Left</p>
<p class="bottom-right">Bottom Right</p>
<p class="center">Center</p>
</div>
</body>
</html>
.container {
position: relative;
}
.top-left {
position: absolute;
top: 5px;
left: 10px;
color: white;
}
.top-right {
position: absolute;
top: 5px;
right: 10px;
color: white;
}
.bottom-left {
position: absolute;
bottom: 5px;
left: 10px;
color: white;
}
.bottom-right {
position: absolute;
bottom: 5px;
right: 10px;
color: white;
}
.center {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
color: white;
}
img {
width: 100%;
height: 200px;
}
Browser Output
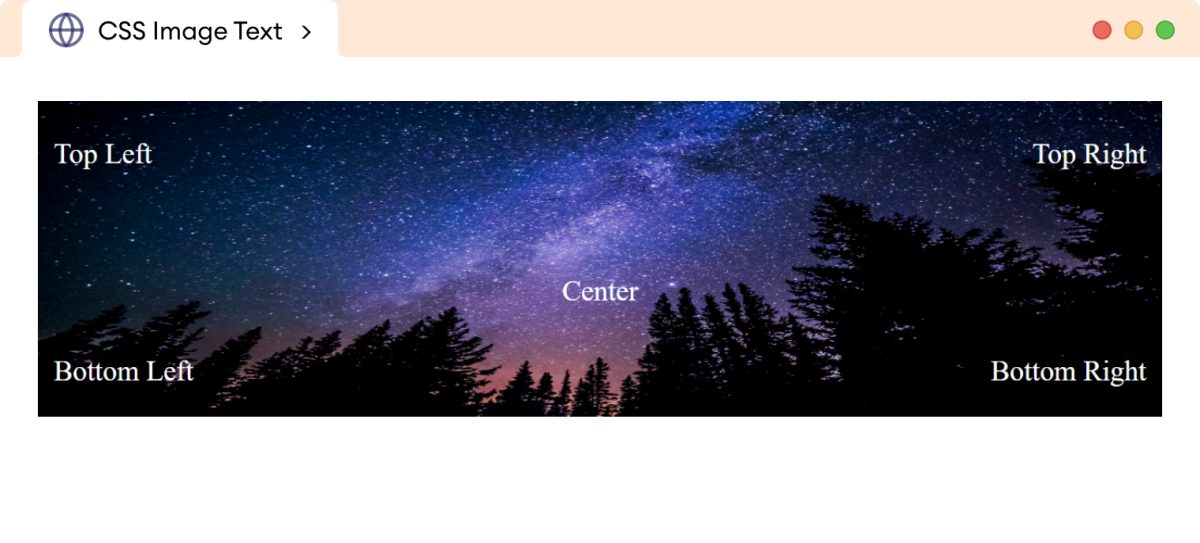
The above example shows different positions of the text on the image.
Note: To absolutely position any inner child element within a container, it is important to set the position of the container to relative.
This is because absolutely positioned elements are positioned relative to their nearest positioned ancestor.
Object Fit
The object-fit
property specifies how an image should fit
inside the container without affecting its aspect ratio.
The object-fit
property can take several values as:
fill
: stretches the image to fill the entire container-
contain
: scales image to fit within the container maintaining its aspect ratio cover
: scales image to cover the entire container-
scale-down
: scales down the image to its smallest version none
: does not resize the image
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Object-Fit Example</title>
</head>
<body>
<h2>Object-Fit: Fill</h2>
<div>
<img
class="fill" src="https://images.unsplash.com/photo-1444080748397-f442aa95c3e5?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1932&q=80"
alt="Image" />
</div>
<h2>Object-Fit: Cover</h2>
<div>
<img
class="cover" src="https://images.unsplash.com/photo-1444080748397-f442aa95c3e5?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1932&q=80"
alt="Image" />
</div>
<h2>Object-Fit: Scale Down</h2>
<div>
<img
class="scale-down" src="https://images.unsplash.com/photo-1444080748397-f442aa95c3e5?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1932&q=80"
alt="Image" />
</div>
<h2>Object-Fit: Contain</h2>
<div>
<img
class="contain" src="https://images.unsplash.com/photo-1444080748397-f442aa95c3e5?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=1932&q=80"
alt="Image" />
</div>
</body>
</html>
img {
width: 200px;
height: 200px;
border: 2px solid #333;
margin: 10px;
}
img.fill {
object-fit: fill;
}
img.cover {
object-fit: cover;
}
img.scale-down {
object-fit: scale-down;
}
img.contain {
object-fit: contain;
}
Browser Output
Object-Fit: Fill
Object-Fit: Cover
Object-Fit: Scale Down
Object-Fit: Contain
The above example shows different values of the
object-fit
property.
Image Filters
The filter
property is used to create various visual effects on
an element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Filter</title>
</head>
<body>
<div>
<p>filter: blur(3px);</p>
<img class="blur" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="buiilding" style="max-width: 200px; filter: blur(3px)"
/>
</div>
<div>
<p>filter: brightness(150%);</p>
<img class="brightness" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: contrast(200%);</p>
<img class="contrast" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: sepia(100%)</p>
<img class="sepia" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: greyscale(100%)</p>
<img class="grayscale" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: hue-rotate(90deg)</p>
<img class="huerotate" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: invert(150%)</p>
<img class="invert" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
<div>
<p>filter: saturate(5)</p>
<img class="saturate" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80" alt="building" />
</div>
</body>
</html>
div {
display: inline-block;
margin: 12px;
}
img {
max-width: 200px;
}
img.blur {
filter: blur(3px);
}
img.brightness {
filter: brightness(150%);
}
img.contrast {
filter: contrast(200%);
}
img.sepia {
filter: sepia(100%);
}
img.greyscale {
filter: greyscale(100%);
}
img.huerotate {
filter: hue-rotate(90deg);
}
img.invert {
filter: invert(150%);
}
img.saturate {
filter: saturate(5);
}
Browser Output
filter: blur(3px);
filter: brightness(150%);
filter: contrast(200%);
filter: sepia(100%)
filter: greyscale(100%)
filter: hue-rotate(90deg)
filter: invert(150%)
filter: saturate(5)
The above example shows different image filters.
Image Hover Overlay
The :hover
pseudo class selector is used to change the overlay
effect on hovering the image. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Overlay</title>
</head>
<body>
<div class="container">
<img src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
<div class="overlay overlay-left">
<p class="overlay-text">Slide in (left)</p>
</div>
</div>
<div class="container">
<img src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
<div class="overlay overlay-right">
<p class="overlay-text">Slide in (right)</p>
</div>
</div>
<div class="container">
<img src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
<div class="overlay overlay-top">
<p class="overlay-text">Slide in (top)</p>
</div>
</div>
<div class="container">
<img src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
<div class="overlay overlay-bottom">
<p class="overlay-text">Slide in (bottom)</p>
</div>
</div>
</body>
</html>
.container {
max-width: 200px;
border: 1px solid black;
position: relative;
overflow: hidden;
margin-bottom: 12px;
}
.overlay {
width: 100%;
height: 100%;
background-color: purple;
position: absolute;
transition: all 0.7s ease;
}
.overlay-text {
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
color: white;
font-weight: bold;
}
img {
width: 100%;
height: 100%;
object-fit: cover;
}
/* creating the left overlay */
.overlay-left {
left: -100%;
top: 0;
}
.container:hover .overlay-left {
left: 0;
}
/* creating the right overlay */
.overlay-right {
right: -100%;
top: 0;
}
.container:hover .overlay-right {
right: 0;
}
/* creating the bottom overlay */
.overlay-bottom {
bottom: -100%;
left: 0;
}
.container:hover .overlay-bottom {
bottom: 0;
}
/* creating the top overlay */
.overlay-top {
top: -100%;
left: 0;
}
.container:hover .overlay-top {
top: 0;
}
Browser Output
The above example shows different overlay effects on hovering the images.
Flip an Image
Let's see how we can flip an image.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Image Flips</title>
</head>
<body>
<div>
<p>Horizontal Flip</p>
<img
class="horizontal-flip"
src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
</div>
<div>
<p>Vertical Flip</p>
<img
class="vertical-flip" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
</div>
<div>
<p>Diagonal Flip</p>
<img
class="diagonal-flip" src="https://images.unsplash.com/photo-1570129477492-45c003edd2be?ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D&auto=format&fit=crop&w=2070&q=80"
alt="buiilding" />
</div>
</body>
</html>
div {
display: inline-block;
margin: 12px;
}
img {
width: 200px;
}
img.horizontal-flip:hover {
transform: scaleX(-1);
}
img.vertical-flip:hover {
transform: scaleY(-1);
}
img.diagonal-flip:hover {
transform: scaleX(-1) scaleY(-1);
}
Browser Output
Horizontal Flip
Vertical Flip
Diagonal Flip
The above example shows different ways of flipping an image.