CSS grid container is a HTML element that serves as a parent element of the grid items.
The following diagram shows a grid container (purple box) with three grid items (orange box).
The CSS grid layout model needs a grid container before using any grid-based properties.
The display
property with the grid
value sets an element as a grid container. For example,
/* A div element (grid container) having
three paragraphs (grid items) */
<div>
<p>First Item</p>
<p>Second Item</p>
<p>Third Item</p>
</div>
/* CSS */
div {
display: grid;
}
Here, the display: grid
sets the div
element as a grid container. All the direct elements within the div
element are referred to as grid items.
The grid container properties define the overall grid structure, such as the number of columns and rows and the size and spacing of the columns and rows.
CSS Grid Container Properties
The grid container has the following set of properties:
Setting up the Grid:
display: grid
- Turns an HTML element into a grid container.
Specifying the Grid Rows and Columns:
grid-template-columns
- Defines the size and number of columns.
grid-template-rows
- Defines the size and number of rows.
grid-template-areas
- Defines named grid areas where the grid items can be placed.
grid-template
- A shorthand for defining one property's rows, columns, and areas.
Specifying the Grid Row and Column Gap:
grid-column-gap
- Defines the gap between columns.
grid-row-gap
- Defines the gap between rows.
grid-gap
- A shorthand for defining both row and column gaps.
Aligning the Grid Items:
justify-items
- Aligns items horizontally within their grid cell.
align-items
- Aligns items vertically within their grid cell.
place-items
- A shorthand for aligning items both horizontally and vertically.
Aligning the Grid:
justify-content
- Aligns the grid along the horizontal axis.
align-content
- Aligns the grid along the vertical axis.
place-content
- A shorthand for aligning content both horizontally and vertically.
Setting Automatic Grid Tracks:
grid-auto-columns
- Defines the size of automatically generated columns.
grid-auto-rows
- Defines the size of automatically generated rows.
grid-auto-flow
- Defines the placement of auto-generated grid items.
Shorthand Grid Property:
grid
- A shorthand property for defining multiple grid properties in a single declaration.
Let's look at each of these properties in detail.
CSS display Property
The grid
value of the display
property sets the element as a grid container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
Browser Output
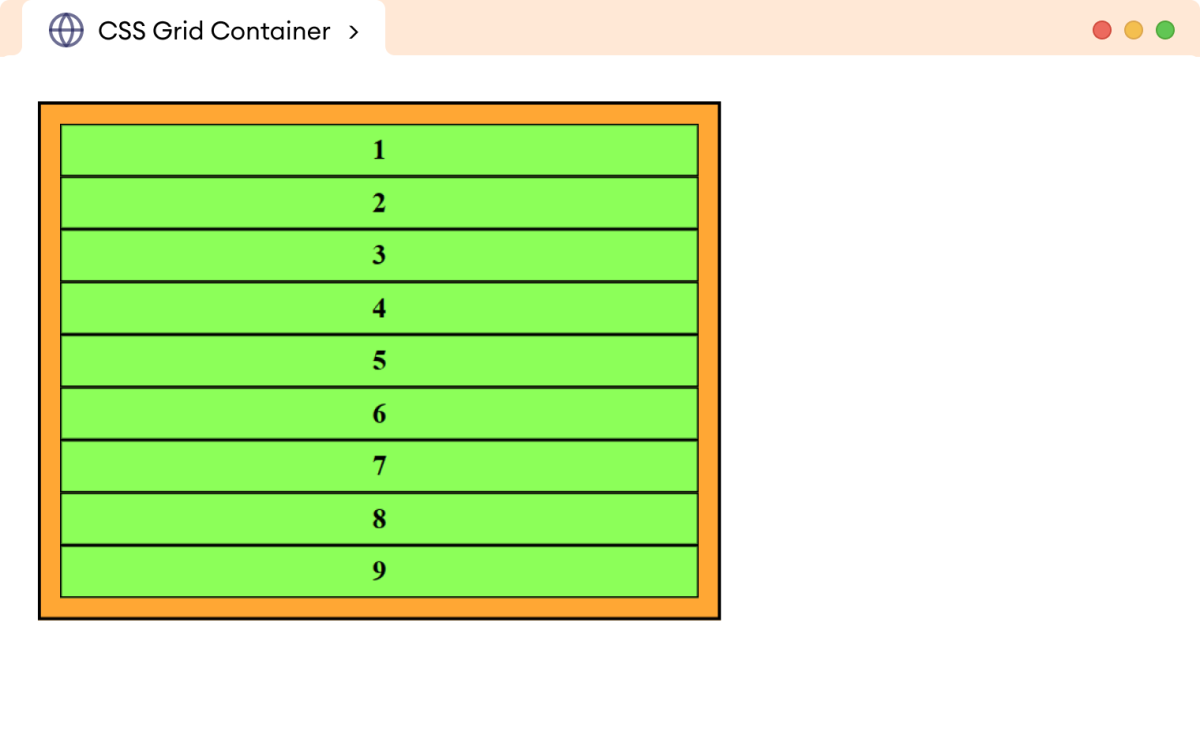
In the above example, display: grid
sets the outer div
element as a grid container.
However, no effects are still visible in the layout, and the grid items, i.e., div
elements, flow normally within the grid container.
CSS grid-template-columns
The grid-template-columns
property defines the size and the number of columns within the grid container.
The syntax of the grid-template-columns
property is:
grid-template-columns: column1-size column2-size column3-size ..
Here, the grid-template-columns
property takes different length values (px, em, percentage, etc.) as the size of the column.
The number of column size values determines the number of columns.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* creates a three columns of size 100px, 200px, 100px respectively */
grid-template-columns: 100px 200px 100px;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
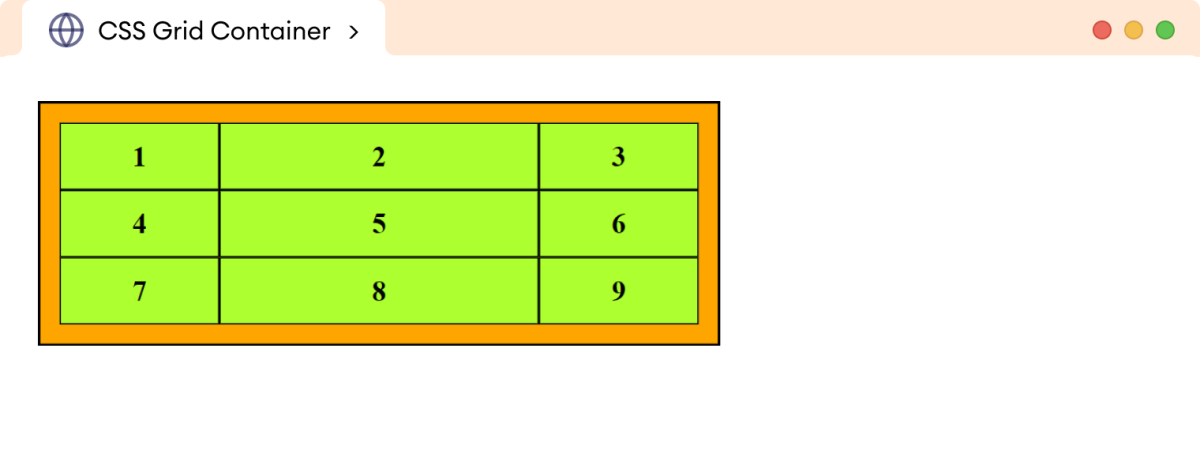
In the above example,
grid-template-columns: 100px 200px 100px;
creates a grid of three columns of size 100px
, 200px
, and 100px
, respectively.
The column size values can also be mixed together as required. For example,
grid-template-columns: 100px 25% 3em;
Browser Output
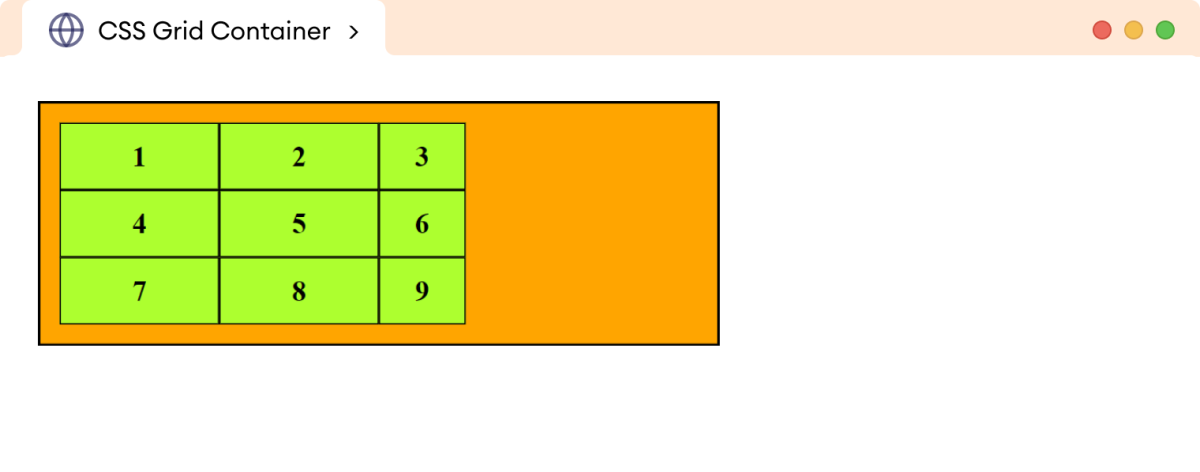
Here,
grid-template-columns: 100px 25% 3em;
creates a grid of three columns of 100px,
25%
of the container's total width, and 3em
, respectively.
CSS grid-template-rows
The grid-template-rows
property defines the size and number of rows in a grid container.
The syntax of the grid-template-rows
property is:
grid-template-rows: row1-size row2-size row3-size ..
Here, the grid-template-rows
take different length values (px, em, percentage, etc.) as the size of the row.
The number of row size values determines the number of rows.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
grid-template-columns: 100px 200px 100px;
/* sets three rows of size 100px, 60px, and 80px respectively */
grid-template-rows: 100px 60px 80px;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
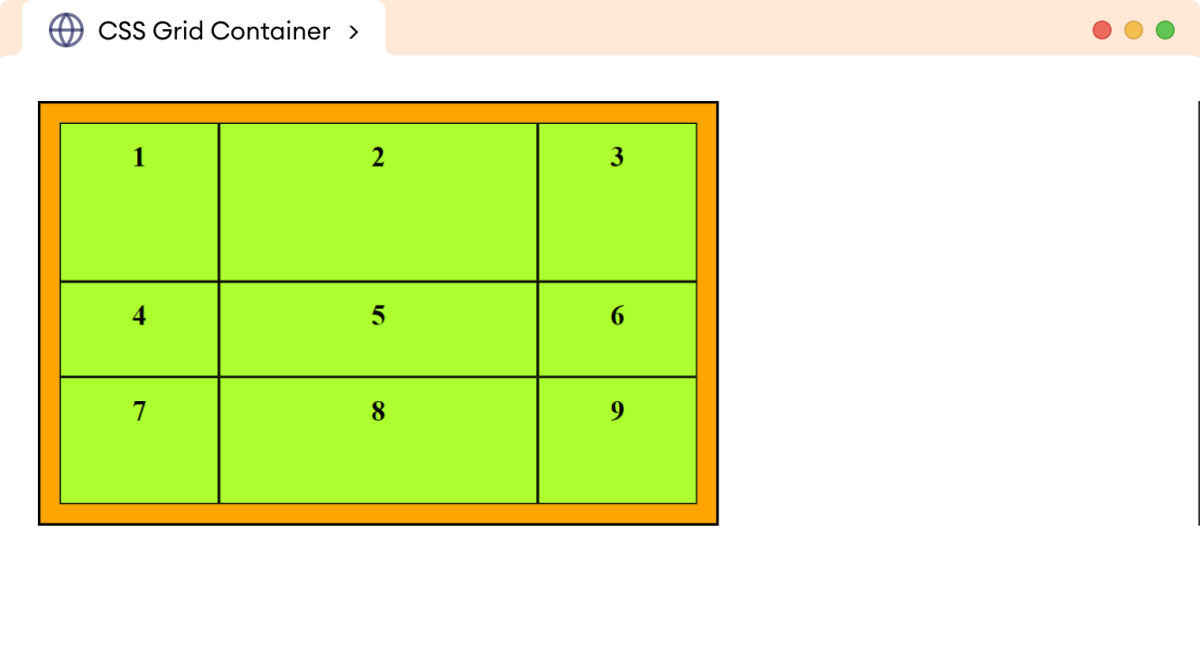
In the above example,
grid-template-rows: 100px 60px 80px;
creates a grid of three rows with the size of 100px
, 60px
, and 80px
, respectively.
Note: The row or column is also referred to as a grid track. A grid track is an adjacent space between two grid lines.
CSS Fractional (Fr) Unit
The fractional unit (fr) divides the available space in the grid container into fractions. It distributes available space within the grid container among columns or rows in a flexible and dynamic way.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* column-1, column-2, column-3 */
grid-template-columns: 1fr 2fr 1fr;
/* row-1, row-2, row-3 */
grid-template-rows: 1fr 1fr 2fr;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
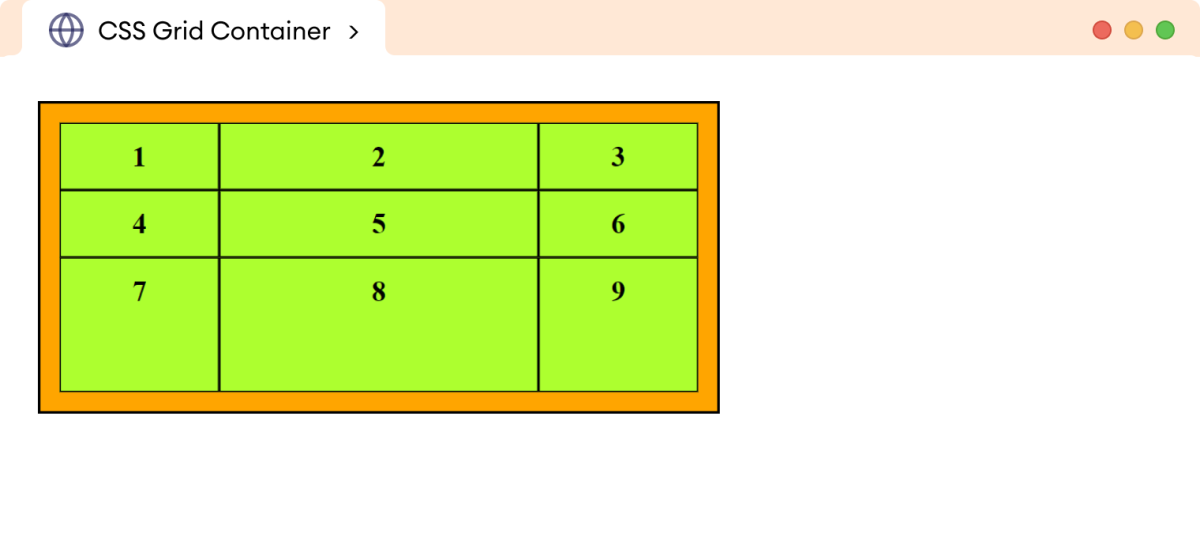
In the above example,
grid-template-columns: 1fr 2fr 1fr;
divides the available horizontal space into four equal parts: the first column occupies one part, the second column occupies two parts, and the third column occupies one part.
Similarly,
grid-template-rows: 1fr 1fr 2fr;
divides the available vertical space into four equal parts: the first and second row occupies one part, while the third row occupies two parts.
Minimum and Maximum Grid Track Sizes
The minmax()
function defines the minimum and maximum size for the grid track i.e. for a row or column size.
The syntax of the minmax()
function is:
minmax(min-size, max-size)
The minmax()
function accepts 2 arguments:
min-size
: the first is the minimum size of the trackmax-size
: the second is the maximum size.
For example,
grid-template-columns: minmax(100px, 300px) 200px;
creates two rows, where
- the first column can dynamically vary in width, ranging from a minimum of
100px
to a maximum of300px
, and - the second column maintains a fixed width of
200px
.
Alongside length (px, em, percentage, etc.) values, the minmax()
function also accepts an auto
value, which allows the track to grow/stretch based on the size of the content.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">
The first column track has a minimum size of auto. This means
that the minimum size it will take is to fit the content. Its
maximum size of 50% will prevent it from getting wider than 50%
of the grid container width.
</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* column-1, column-2, column-3 */
grid-template-columns: minmax(auto, 80%) 1fr 3em;
/* row-1, row-2, row-3 */
grid-template-rows: 1fr 1fr 3fr;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
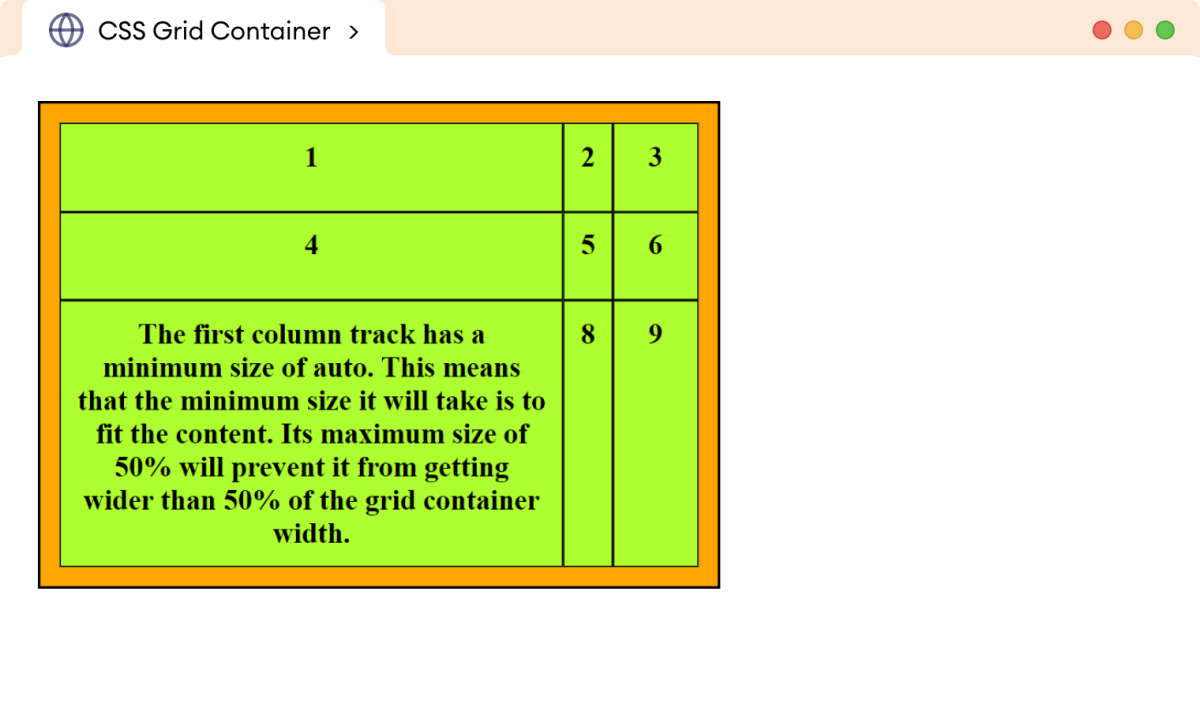
In the above example, the first column has the value of
minmax(auto, 80%)
which causes the column to automatically adjust its width to fit its content, but it won't exceed 80%
of the available space in the grid container.
Repeating Grid Tracks
The repeat()
function defines the repeating grid tracks. This is useful for grids with items of equal sizes.
The syntax of the repeat()
function is:
repeat(no of tracks, track size)
The repeat()
notation accepts two arguments:
no of tracks
: the first represents the number of times the defined tracks should repeattrack size
: the second is the track size.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* creates 3 columns of 100px each */
grid-template-columns: repeat(3, 100px);
/* creates 3 rows of equal fraction */
grid-template-rows: repeat(3, 1fr);
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
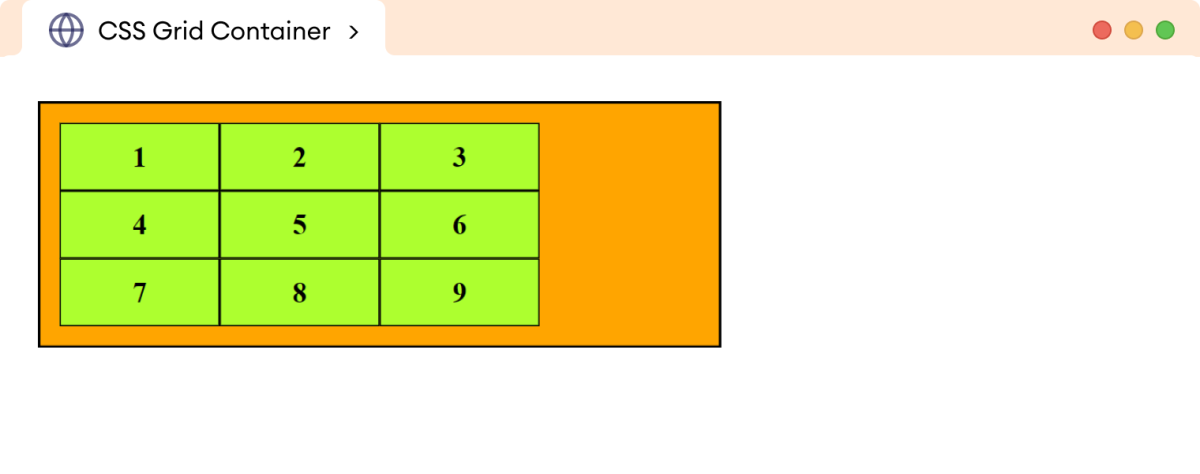
In the above example,
grid-template-columns: repeat(3, 100px)
creates three columns, each with a width of 100px
size.
And,
grid-template-rows: repeat(3, 1fr)
creates three rows occupying an equal fraction of the available vertical space.
CSS grid-template-areas
The grid-template-areas
property defines the layout of the grid container using named grid areas.
The syntax of the grid-template-areas
property is:
grid-template-areas:
"area-name1 area-name2 area-name3"
"area-name4 area-name5 area-name6"
/* ... additional rows ... */;
Here,
- each row within the double quotation marks represents a row in the grid
- each area name corresponds to a named grid area, and they are separated by spaces
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<header>HEADER</header>
<aside class="left">SIDEBAR 1</aside>
<aside class="right">SIDEBAR 2</aside>
<main>MAIN SECTION</main>
<footer>FOOTER</footer>
</div>
</body>
</html>
div.container {
display: grid;
/* defines the columns */
grid-template-columns: 1fr 3fr 1fr;
/* defines the rows */
grid-template-rows: 40px 100px 40px;
/* defines the grid areas placing the grid items */
grid-template-areas:
"header header header"
"sidebar1 main sidebar2"
"footer footer footer";
border: 2px solid black;
text-align: center;
}
header {
/* places the header within the header grid area */
grid-area: header;
background-color: skyblue;
}
aside.left {
/* places left sidebar within the sidebar1 grid area */
grid-area: sidebar1;
}
aside.right {
/* places right sidebar within the sidebar2 grid area */
grid-area: sidebar2;
}
main {
/* places the main content within the main grid area */
grid-area: main;
background-color: greenyellow;
}
footer {
/*places the footer in the footer grid area*/
grid-area: footer;
background-color: skyblue;
}
Browser Output
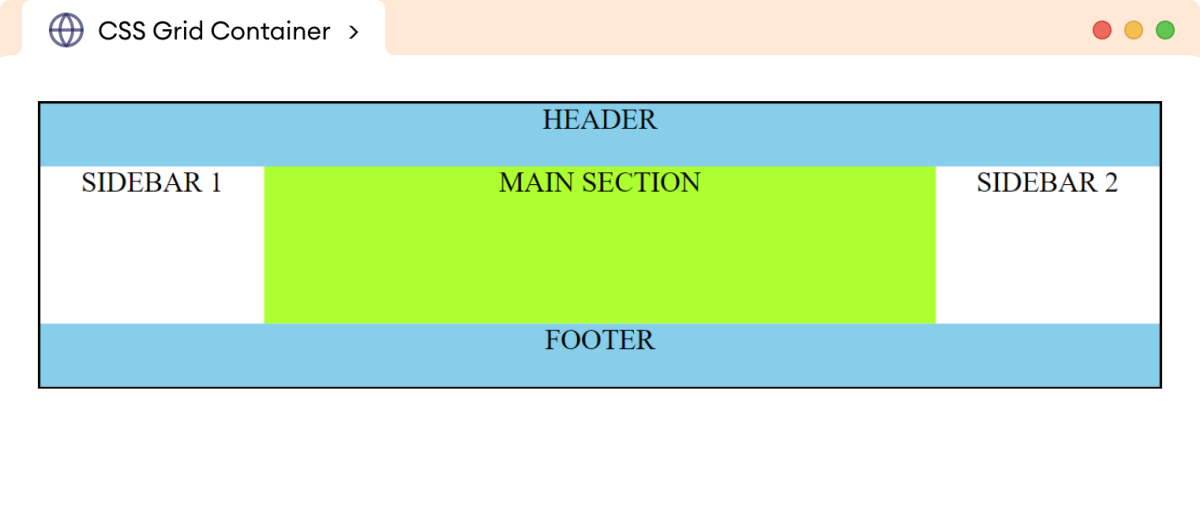
In the above example,
grid-template-areas:
"header header header"
"sidebar1 main sidebar2"
"footer footer footer";
defines a grid layout with distinct areas for five elements: the header, two sidebars, main content, and footer sections.
The grid-area
property allows the placement of grid items in the respective grid address. For example,
header {
grid-area: header;
}
allows the header
element to completely occupy the first row of the grid container.
The elements get placed in the respective grid area mentioned by the grid-template-areas
property.
CSS grid-template Property
The grid-template
is a shorthand property to specify grid-template-rows
, grid-template-columns
, and grid-template-areas
in a single declaration.
The syntax of the grid-template
property is:
grid-template: none | grid-template-rows / grid-template-columns
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
</div>
</body>
</html>
div.container {
display: grid;
/* grid-template-rows / grid-template-columns */
grid-template: 50px 150px 50px / 1fr 1fr 1fr;
width: 400px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 10px;
font-weight: bold;
}
Browser Output
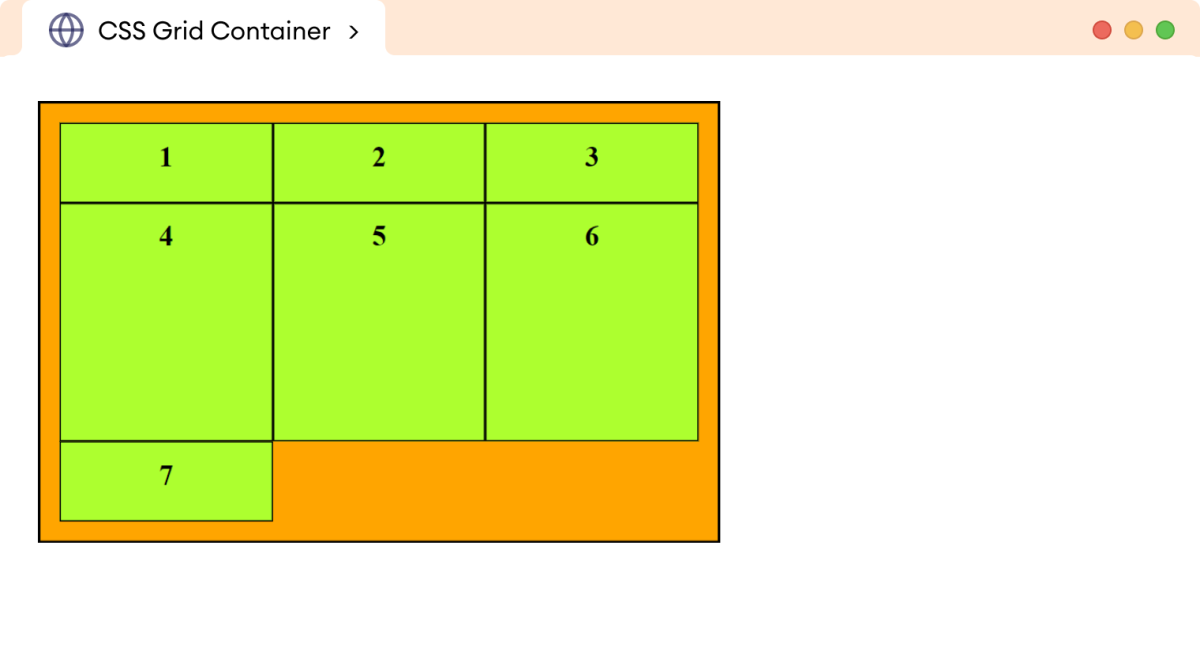
In the above example,
grid-template: 50px 150px 50px / 1fr 1fr 1fr;
creates a grid container of three rows of 50px
, 150px
, and 50px
respectively and three columns having equal fractions of grid container width.
CSS grid-column-gap Property
The grid-column-gap
property defines the spacing between columns in a grid container.
The syntax of the grid-column-gap
property is:
gird-column-gap: value
The value can be any valid length unit, such as pixels, percentages, ems, rems, etc.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines 3 rows of 80px each */
grid-template-rows: repeat(3, 80px);
/* defines 3 columns of equal fraction */
grid-template-columns: repeat(3, 1fr);
/* creates a column gap of 20px */
grid-column-gap: 20px;
width: 400px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 30px;
font-weight: bold;
}
Browser Output
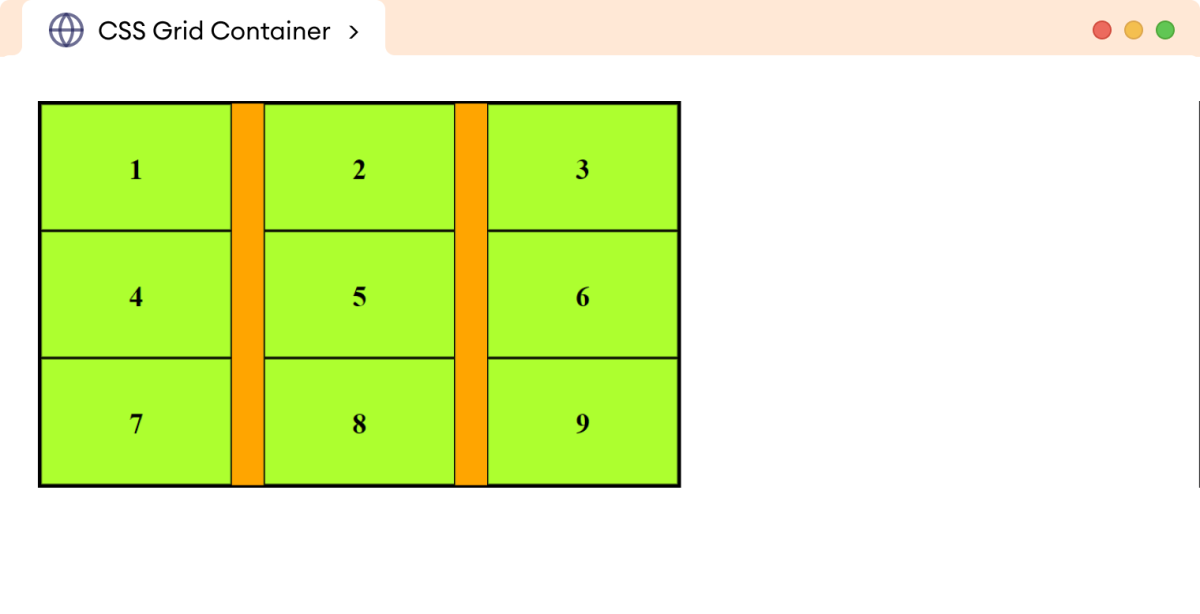
In the above example,
grid-column-gap: 20px
defines a 20px
gap between the adjacent columns of the grid container.
CSS grid-row-gap Property
The grid-row-gap
property defines the spacing between the rows of the grid container.
The syntax of the grid-row-gap
property is:
gird-row-gap: value
The value can be any valid length unit, such as pixels, percentages, ems, rems, etc.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines three rows of 80px each */
grid-template-rows: repeat(3, 80px);
/* defines three columns of equal fraction */
grid-template-columns: repeat(3, 1fr);
/* creates a row gap of 20px */
grid-row-gap: 20px;
width: 400px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 30px;
font-weight: bold;
}
Browser Output
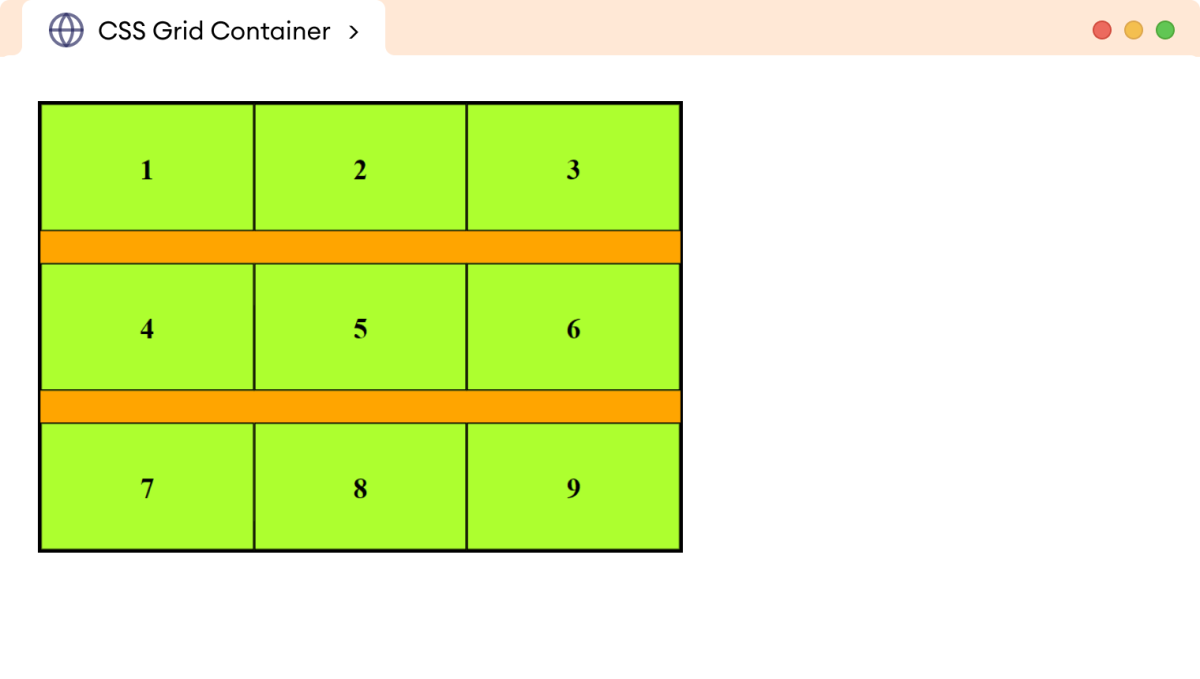
In the above example,
grid-row-gap: 20px
defines a 20px
gap between the adjacent rows of the grid container.
CSS grid-gap property
The grid-gap
is a shorthand property to specify the grid-column-gap
and grid-row-gap
simultaneously. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines three rows of 80px each */
grid-template-rows: repeat(3, 80px);
/* defines three columns of equal fraction */
grid-template-columns: repeat(3, 1fr);
/* creates a row and column gap of 20px */
grid-gap: 20px;
width: 400px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 30px;
font-weight: bold;
}
Browser Output
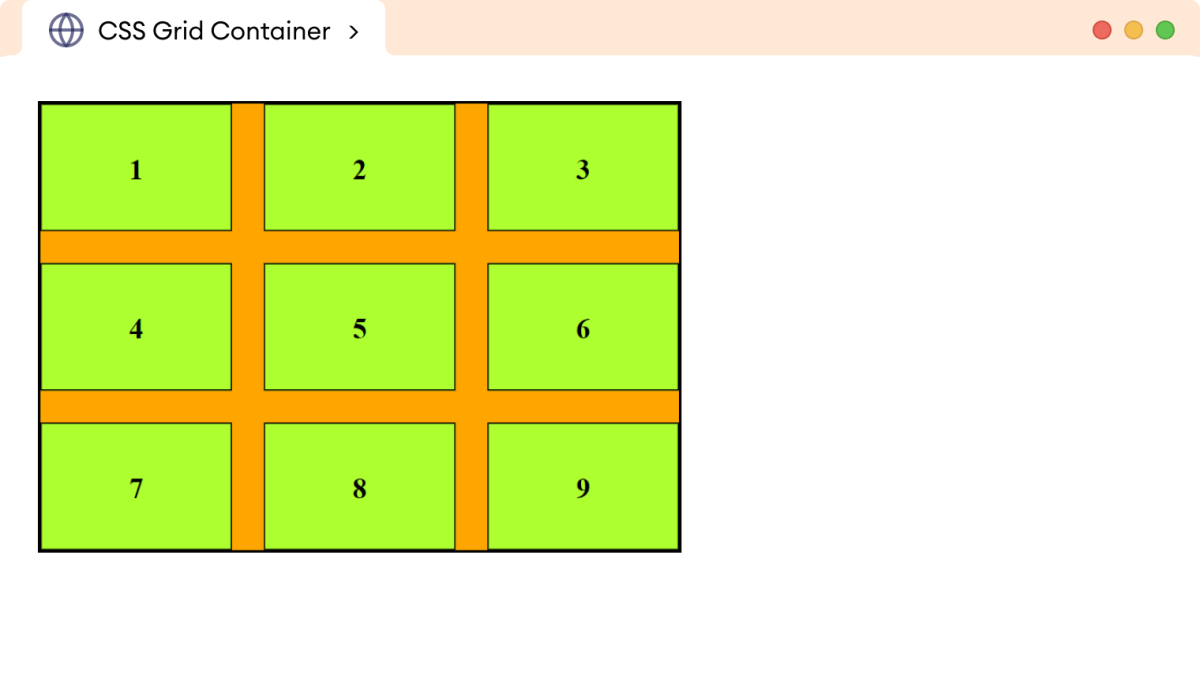
In the above example,
grid-gap: 20px
defines a gap of 20px
between the adjacent columns and rows of the grid container.
CSS justify-content Property
The justify-content
property controls the horizontal alignment of the grid within the grid container.
The possible values of the justify-content
property are start
, end
, center
, space-around
, space-between
, and space-evenly
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* aligns the entire grid to center in the grid container*/
justify-content: center;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
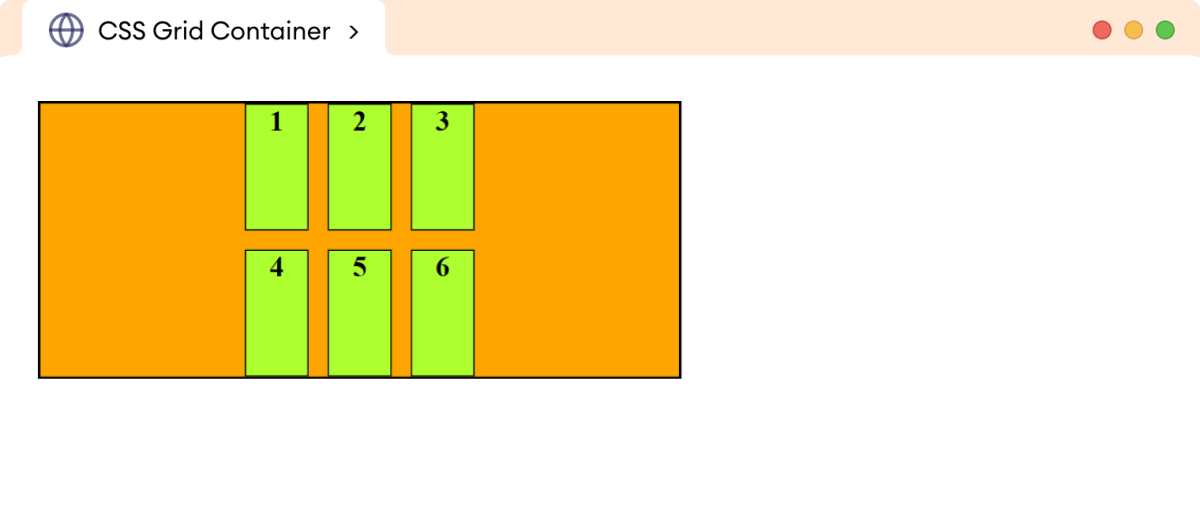
In the above example,
justify-content: center
aligns the grid to the center of the grid container.
Note: The effect of justify-content is only possible when the size of the grid formed is less than the size of the grid container.
Values of justify-content Property
The start
value of justify-content
aligns the grid to the starting edge of the grid container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* aligns the grid to start of the grid container*/
justify-content: start;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
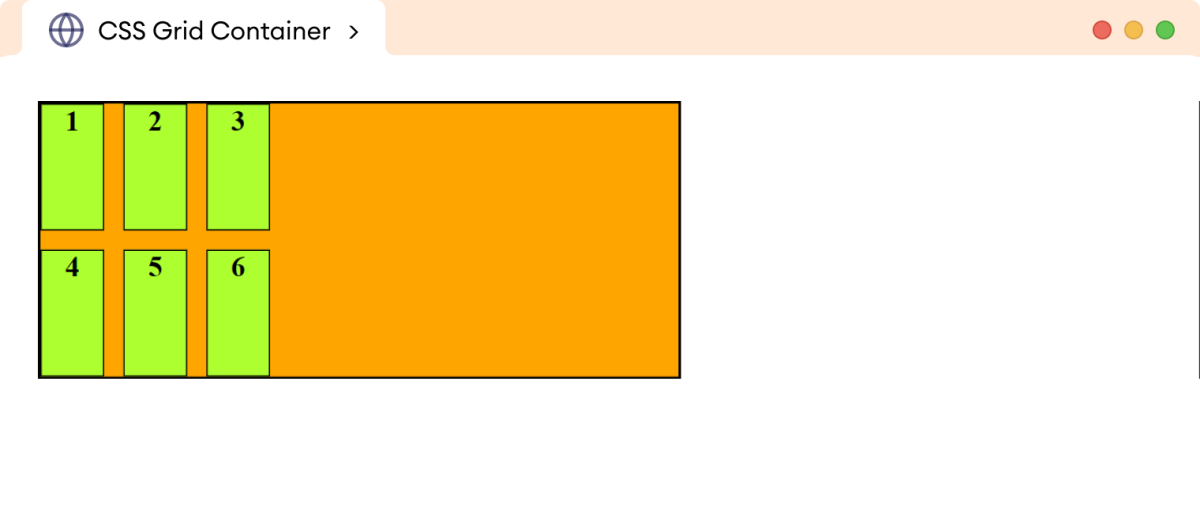
The end
value of justify-content
aligns the grid to the ending edge of the grid container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* aligns the grid to end of the grid container*/
justify-content: end;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
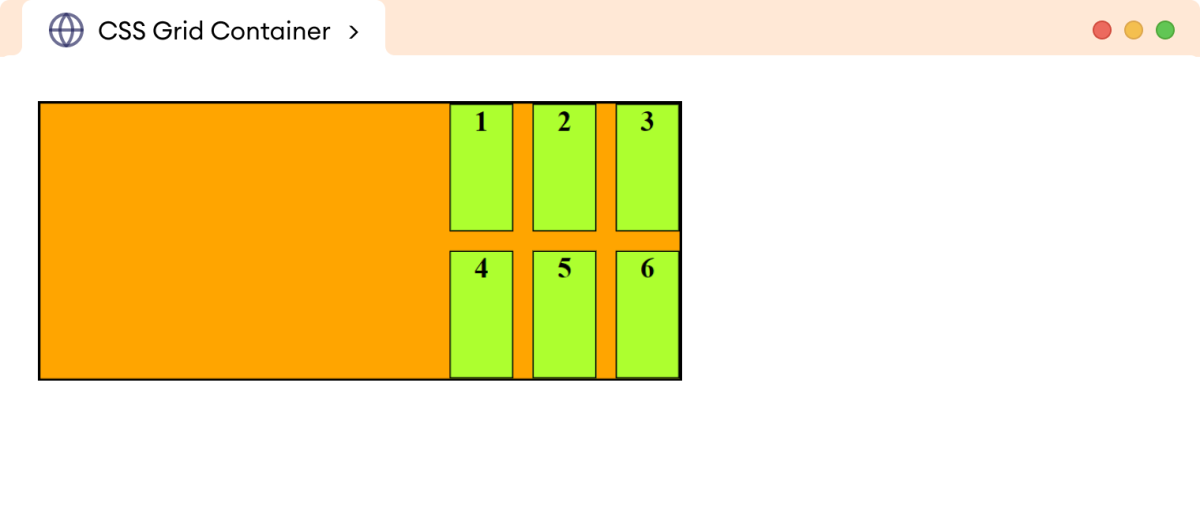
The space-around
value of justify-content
evenly distributes space between grid items horizontally, while also maintaining half the space at the far ends. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and column */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* adds the space around the grid items */
justify-content: space-around;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
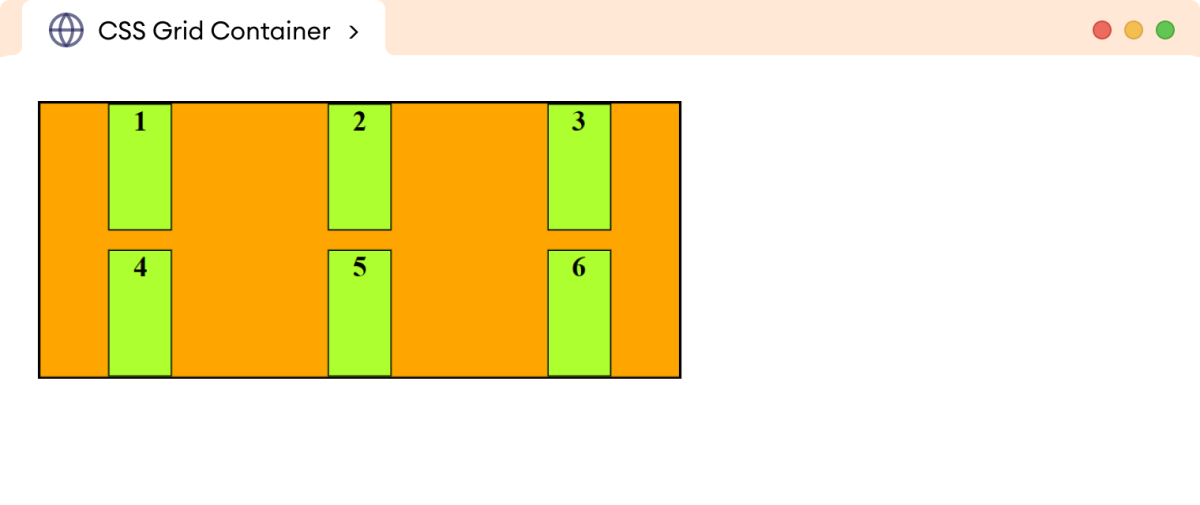
The space-between
value of justify-content
only distributes the space evenly between the grid items. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* adds the space only between the gird items */
justify-content: space-between;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
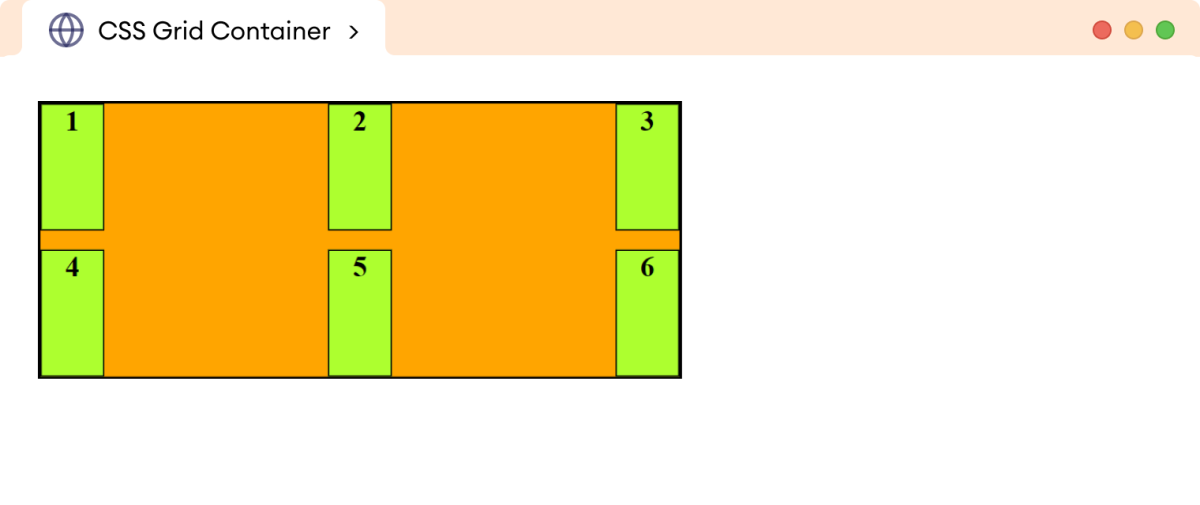
The space-evenly
value of the justify-content
distributes the space evenly between the grid items, including the first and last grid item. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 40px);
/* distributes the space evenly between the items */
justify-content: space-evenly;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
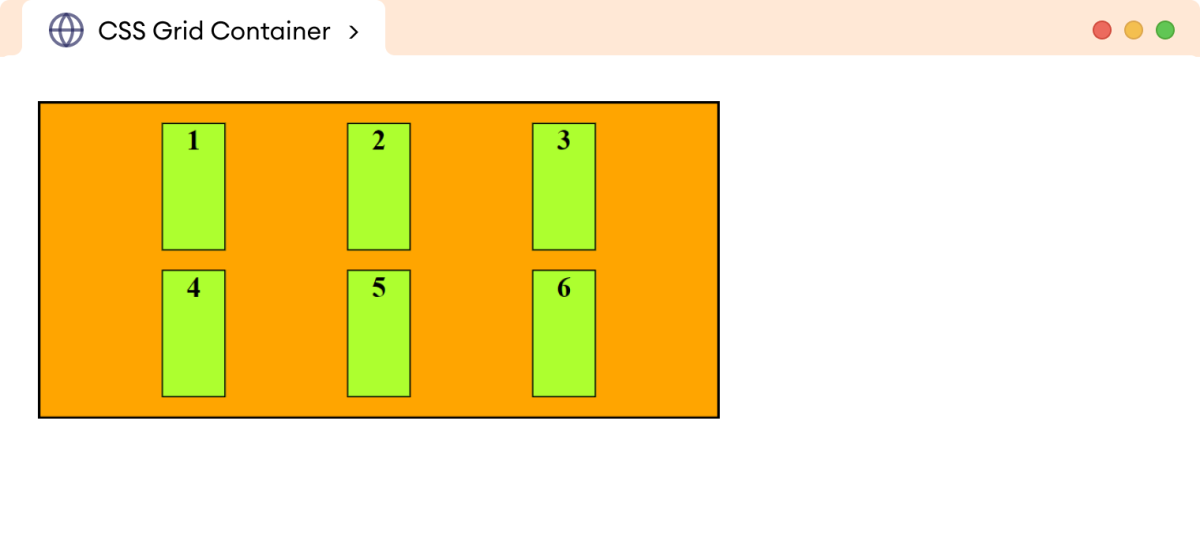
CSS align-content Property
The align-content
property controls the alignment of the grid within the grid container along the horizontal direction.
The possible values of the align-content
property are start
, end
, center
, space-around
, space-between
, and space-evenly
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* aligns the grid vertically to center of grid container */
align-content: center;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
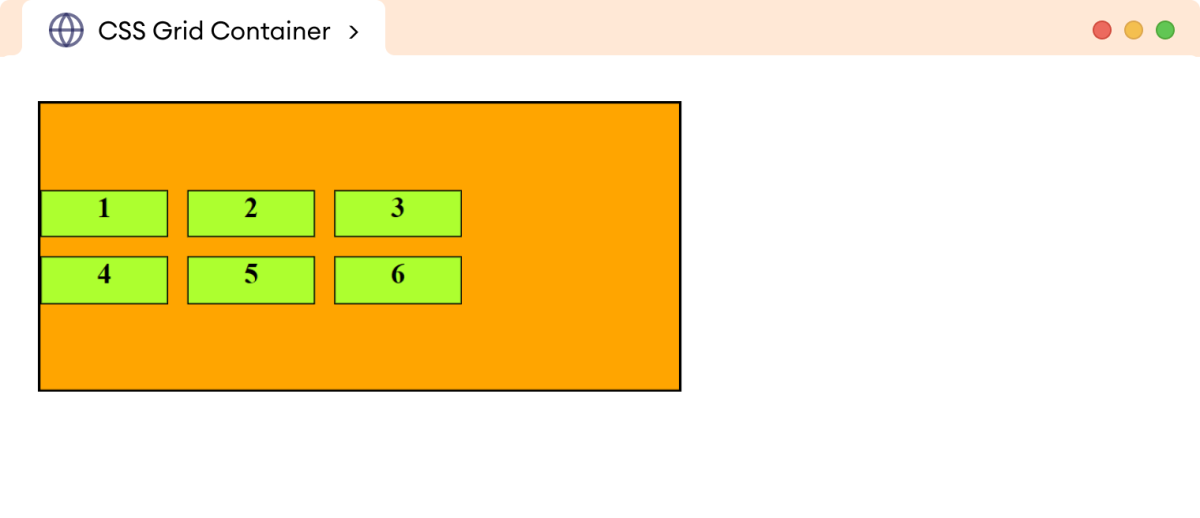
Here,
align-content: center
aligns the grid in the center of the grid container vertically.
Note: The align-content
will have only effect when the grid container is larger than the grid itself.
Values of align-content Property
The start
value of the align-content
property aligns the grid to the top edge of the grid container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines the rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* aligns the grid to the top of grid container*/
align-content: top;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
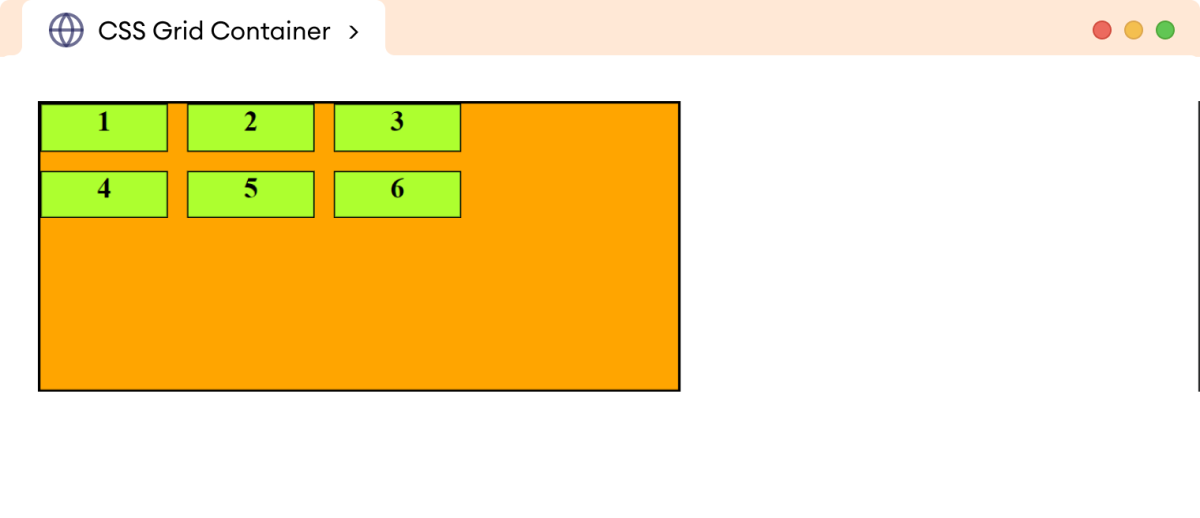
The end
value of the align-content
aligns the grid to the bottom edge of the grid container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* aligns the grid to bottom of grid container*/
align-content: end;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
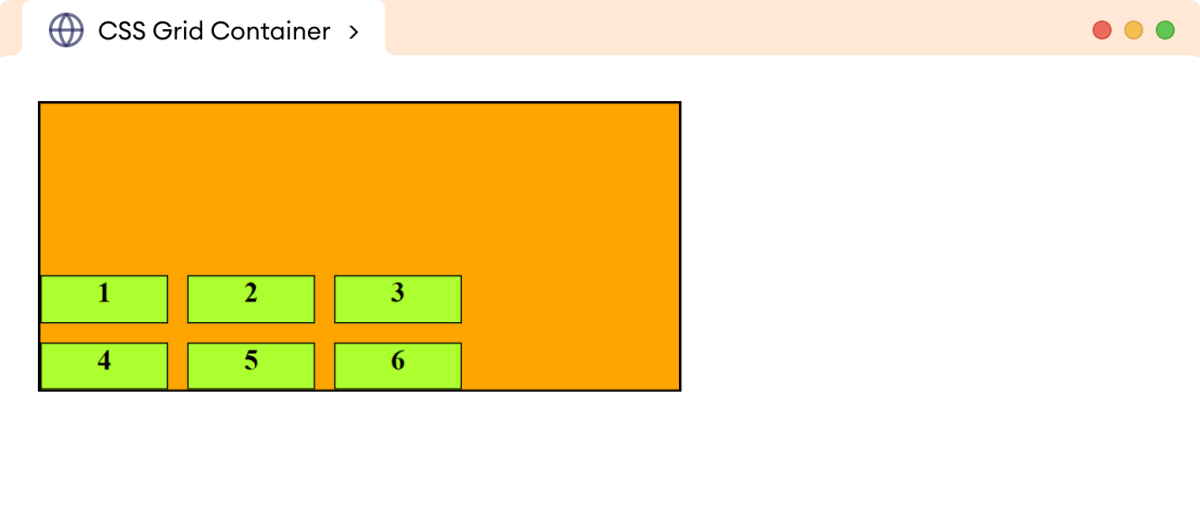
The space-around
value of align-content
evenly distributes space between grid items vertically, while also maintaining half the space at the far ends. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* vertically aligns the space */
align-content: space-around;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
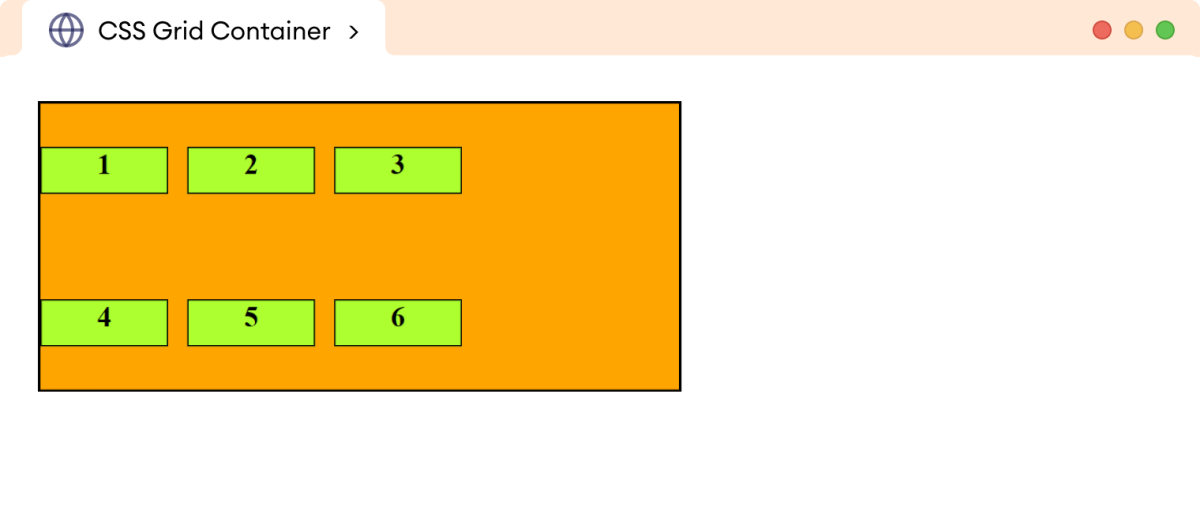
The space-between
value of align-content
only distributes the space evenly between the grid items vertically. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* distributes the space between the items vertically */
align-content: space-between;
width: 400px;
column-gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
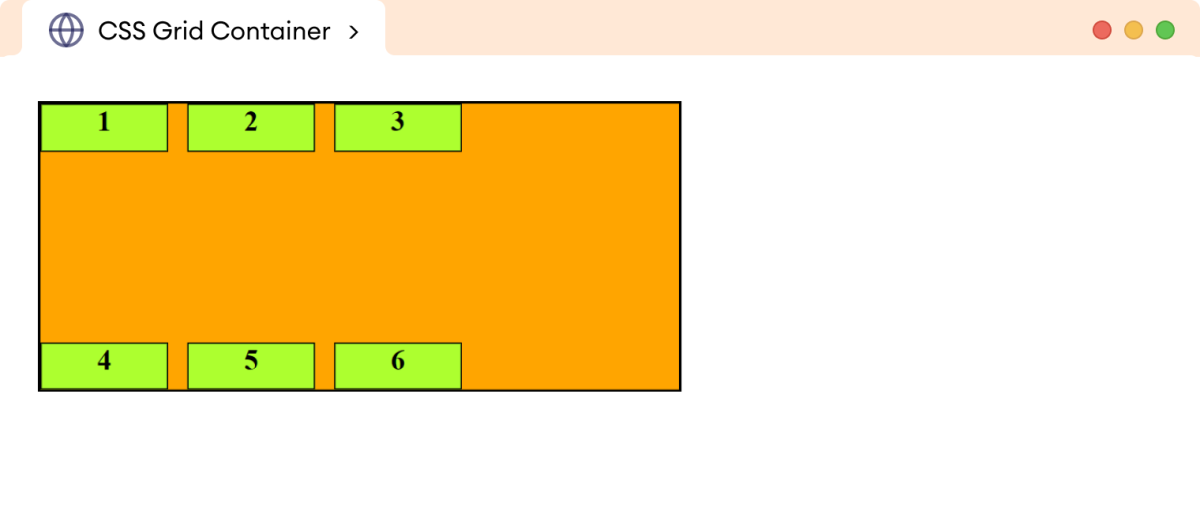
The space-evenly
value of the justify-content
distributes the space evenly between the grid items, including the first and last grid item vertically. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* distributes the space evenly in vertical direction */
align-content: space-evenly;
width: 400px;
column-gap: 12px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
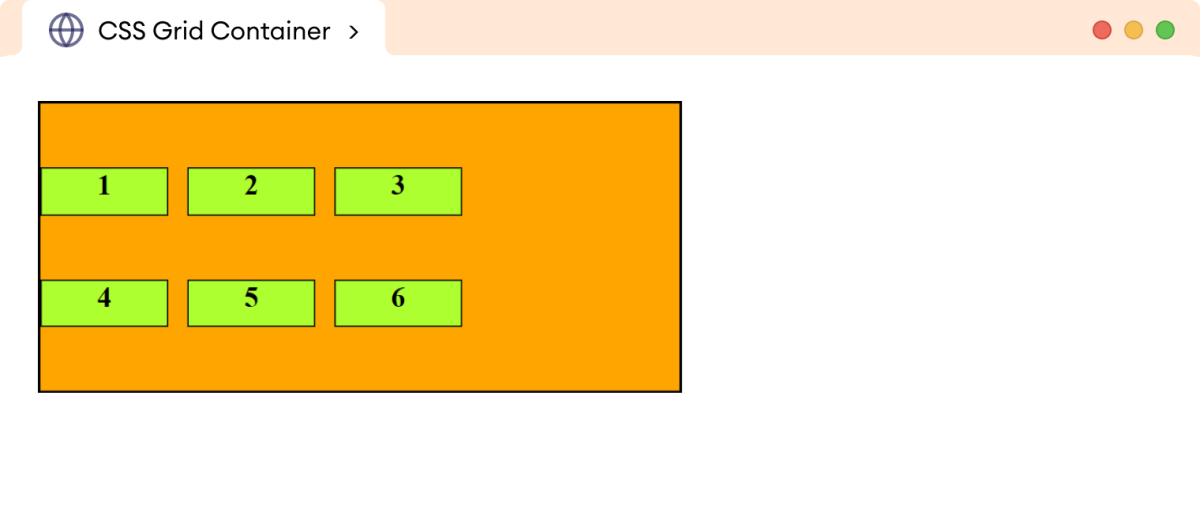
CSS place-content Property
The place-content
property is a shorthand property to specify the justify-content
and align-content
in a single declaration.
The syntax of the place-content-property
is:
place-content: align-content place-content
Here, the first value sets align-content,
and the second value sets justify-content
. If the second value is omitted, the first value is assigned to both properties.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
width: 400px;
height: 180px;
/* defines rows and columns */
grid-template-rows: repeat(2, 30px);
grid-template-columns: repeat(3, 80px);
/* sets align-content to center and justify-content to end of grid container*/
place-content: center end;
column-gap: 20px;
border: 2px solid black;
background-color: orange;
}
/* styles all grid items */
div.item {
text-align: center;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
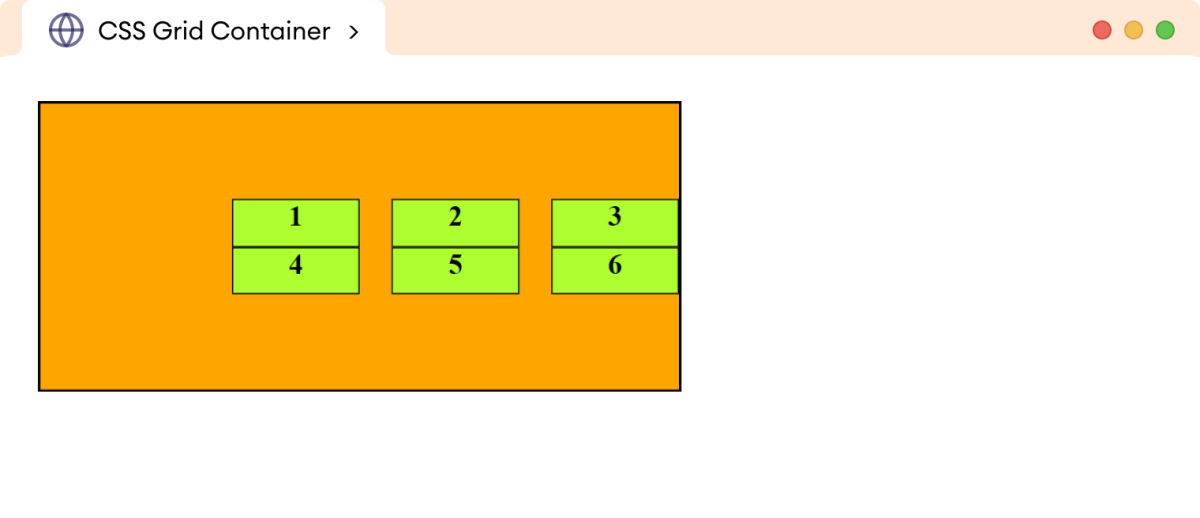
In the above example,
place-content: center end
sets the align-content
property to center
and justify-content
to end.
As a result, the grid is aligned in the center vertically and to the right end horizontally.
CSS justify-items Property
The justify-items
property controls the horizontal alignment of the grid items within the respective grid cells.
The common values for the justify-items
property are start
, end
, center
, and stretch
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the end of grid cells */
justify-items: end;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
width: 20px;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
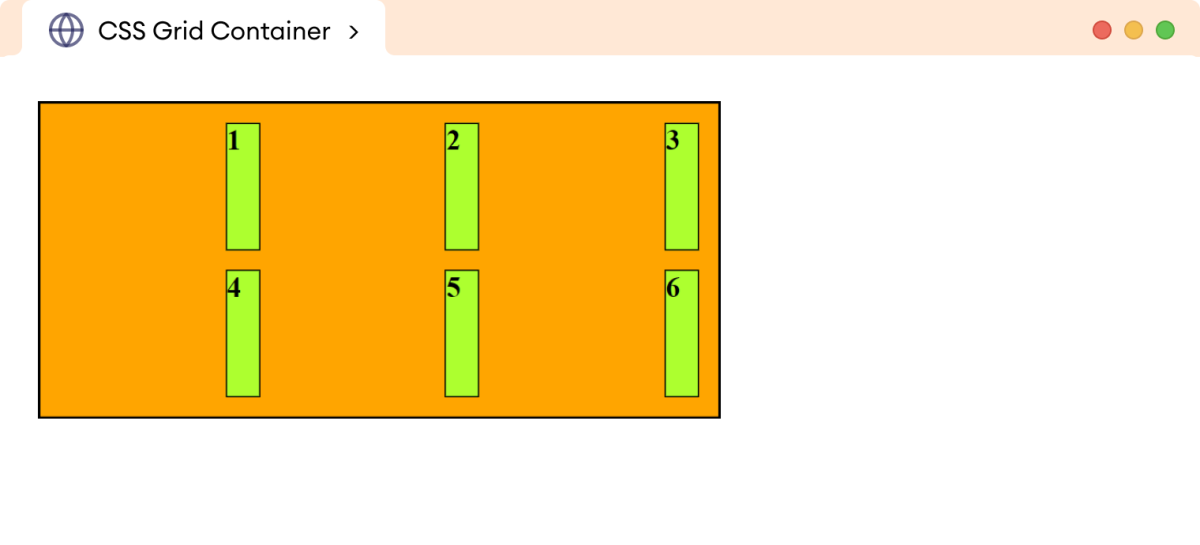
Here, the end
value of the justify-items
property aligns the grid item at the end of the grid cell.
Note: The individual items can also be self-aligned using the justify-self
property. This property should be specified on the grid item.
Values of justify-items Property
The start
value of the justify-items
aligns the grid item at the start of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the start of grid cells */
justify-items: start;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
width: 20px;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
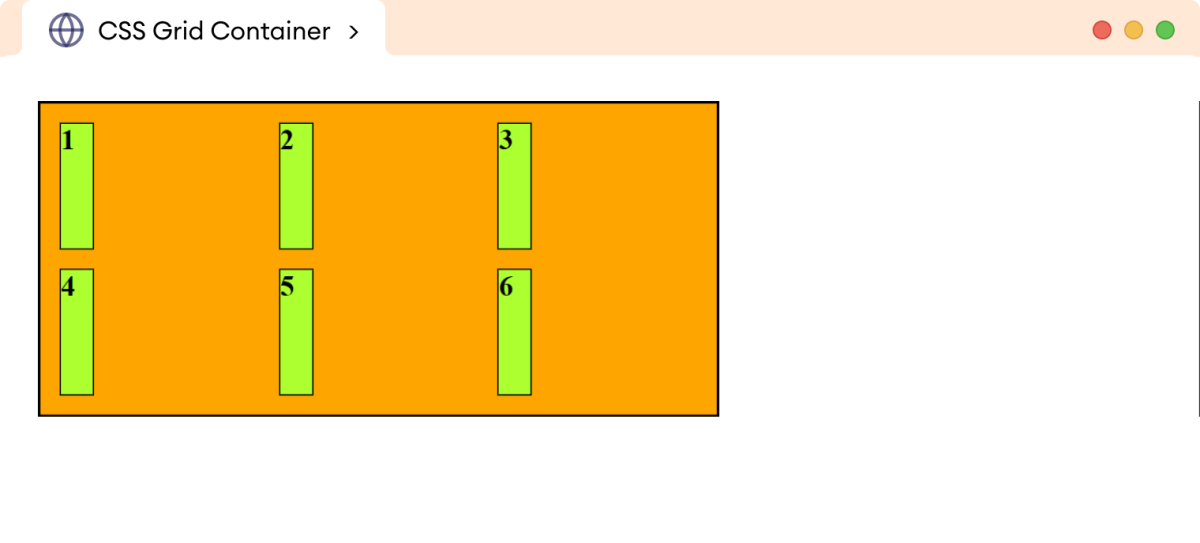
Here, the justify-items: start
aligns all the grid items at the start of the grid cells.
The center
value of the justify-items
aligns the grid items at the center of the grid cells. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the center of grid cells */
justify-items: center;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
width: 20px;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
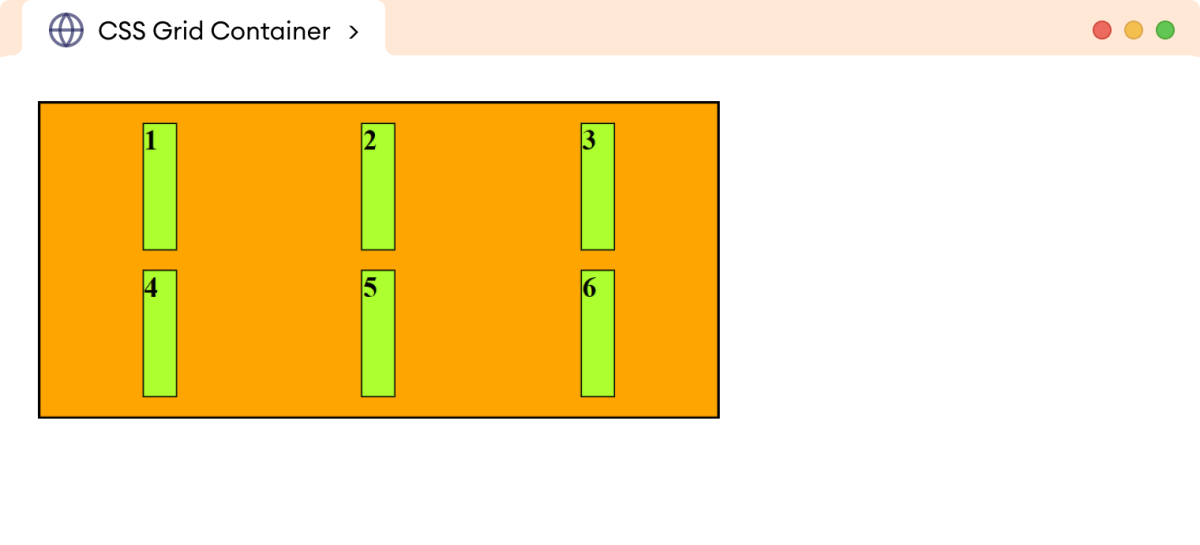
Here, justify-items: center
aligns all the grid items at the center of grid cells.
The stretch
value of justify-items
stretches the grid items to fill the entire grid cells. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* stretches the grid items to fill the grid cells */
justify-items: stretch;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
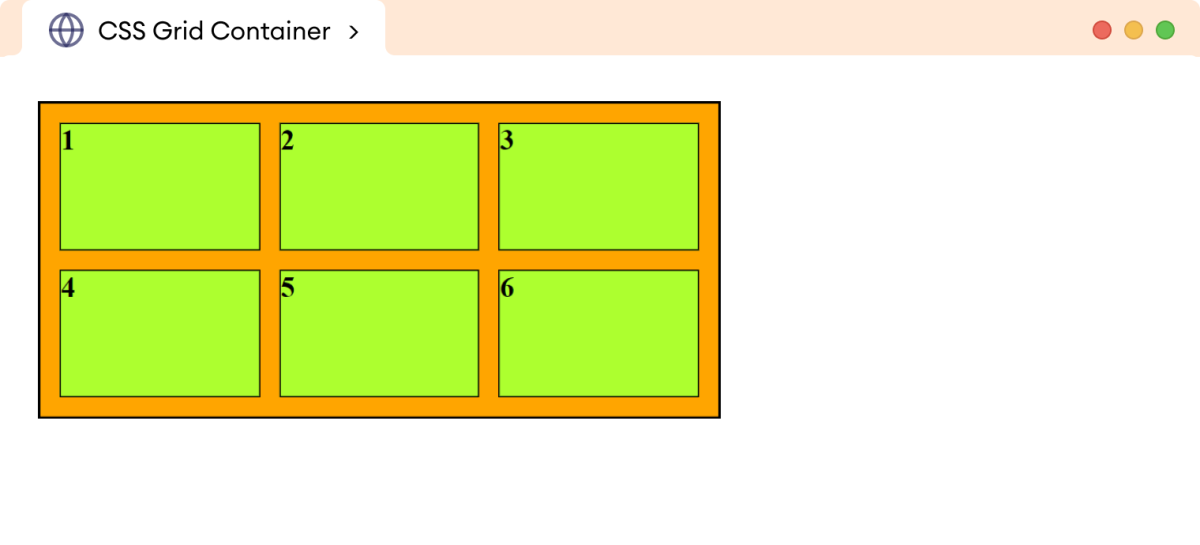
Here, all the grid items are stretched to fill the entire grid cell.
CSS align-items Property
The align-items
property controls the vertical alignment of the grid items within the respective grid cells.
The common values for the align-items
property are start
, end
, center
, and stretch
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the bottom of grid cells */
align-items: end;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
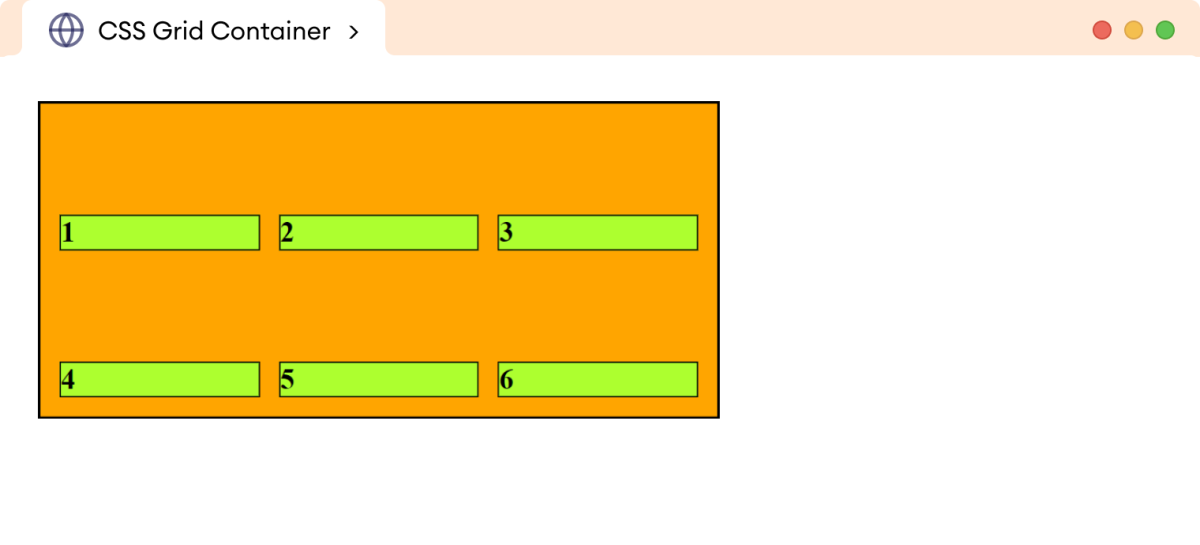
Here, the end
value of the align-items
property aligns the grid item at the bottom of the grid cell.
Note: The individual items can also be self-aligned using the align-self
property. This property should be specified on the grid item.
Values of align-items Property
The start
value of the align-items
aligns the grid item at the top of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the top of grid cells */
align-items: start;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
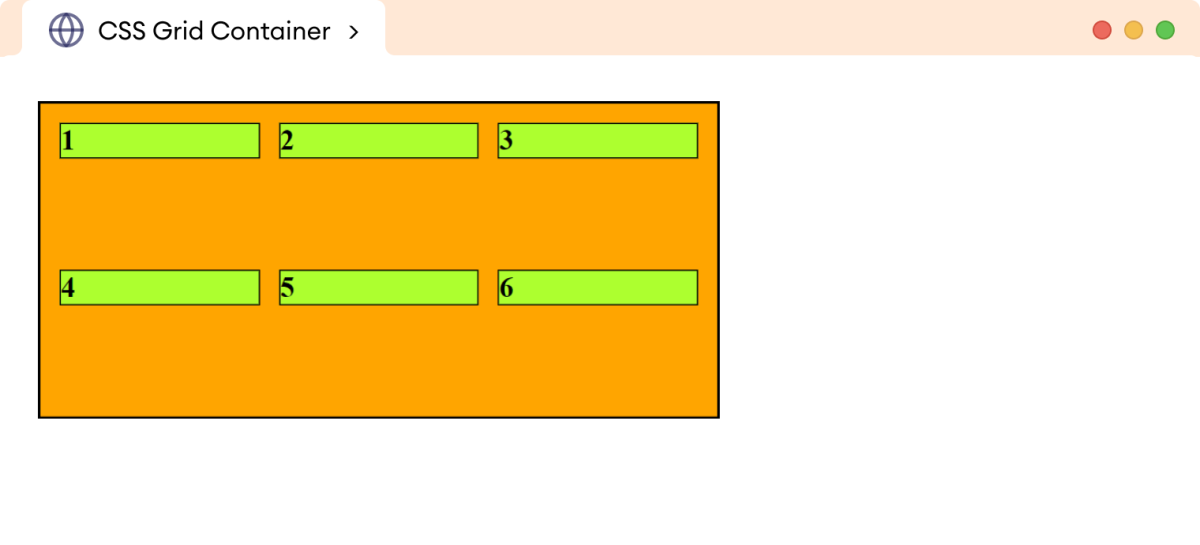
Here, the align-items: start
aligns all the grid items at the top of the grid cells.
The center
value of the align-items
aligns the grid items at the center of the grid cells. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the center of grid cells */
align-items: center;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
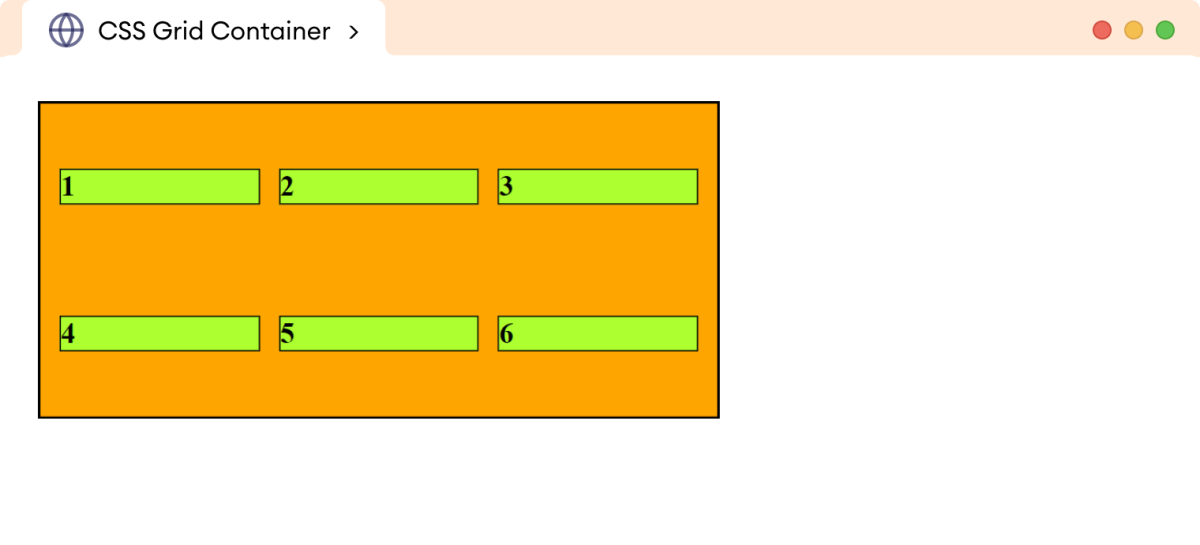
Here, align-items: center
aligns all the grid items at the center of grid cells.
The stretch
value of align-items
stretches the grid items to fill the entire grid cells. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* aligns the grid items at the top of grid cells */
align-items: stretch;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
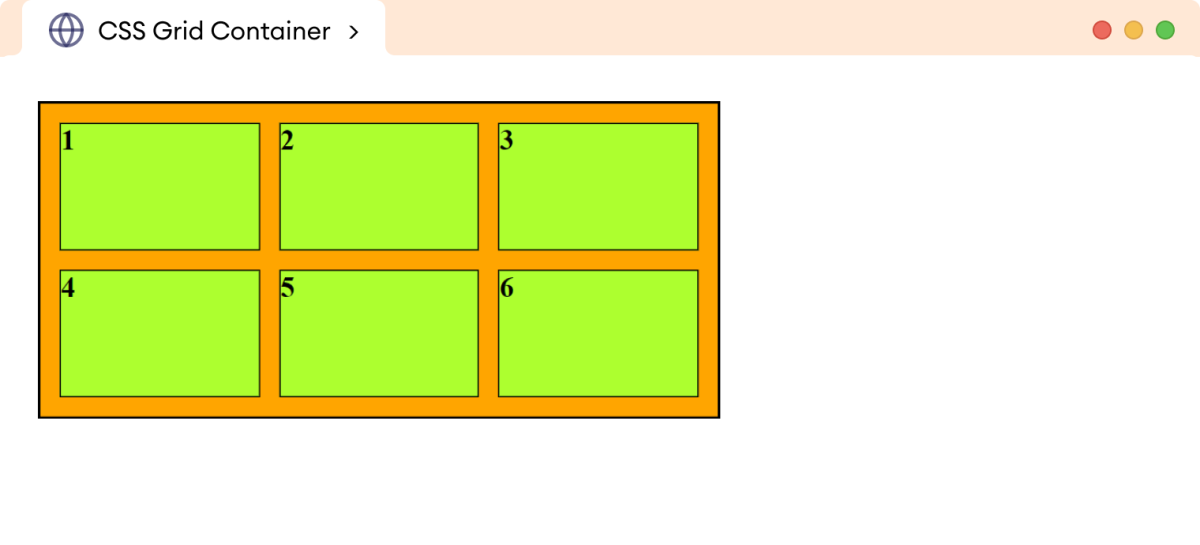
Here, all the grid items are stretched to fill the entire grid cell.
CSS place-items
The place-items
is a shorthand property to specify the align-items
and justify-items
property in a single declaration.
The syntax of place-items
is as follows,
place-items: align-items justify-items
Here, the first value sets the align-items
, and the second value sets the justify-items
property. If the second value is omitted, the first value is set for both properties.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* defines rows and columns */
grid-template-rows: repeat(2, 80px);
grid-template-columns: repeat(3, 1fr);
/* sets align-items and justify-items to the center of grid cell */
place-items: center;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
width: 40px;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
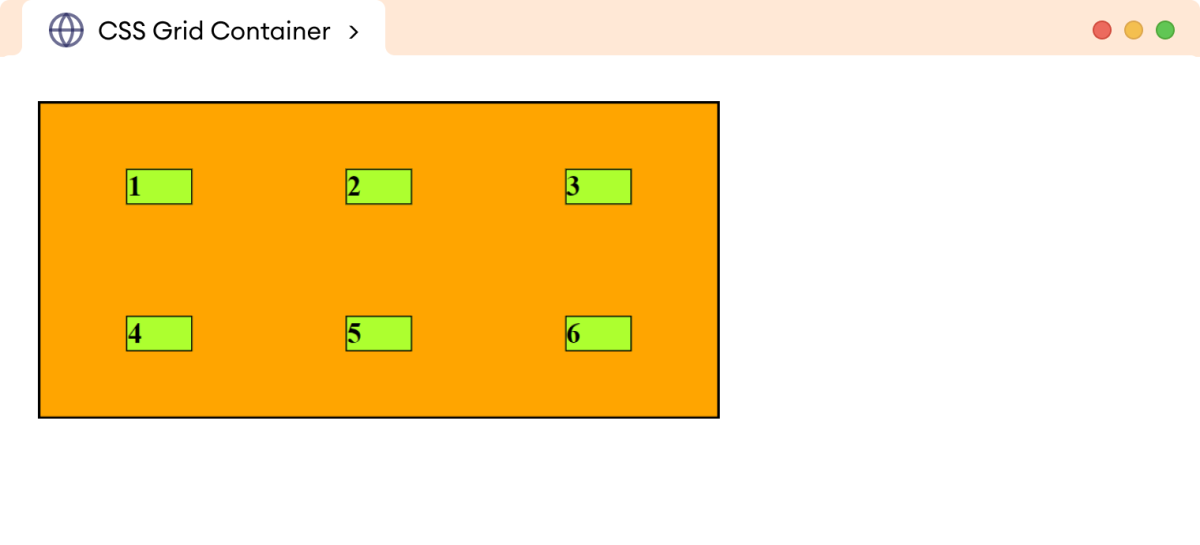
Here, the place-items
property sets both align-items
and justify-items
properties to the center.
As a result, the grid content is aligned at the center vertically and horizontally within the grid cell.
CSS grid Property
The grid
is a shorthand property to specify grid-template-rows
and grid-template-columns
properties in a single declaration.
The syntax of the grid
property is:
grid: none | grid-template-rows / grid-template-columns
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Container</title>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
</div>
</body>
</html>
div.container {
display: grid;
/* grid-template-rows / grid-template-columns */
grid: 50px 100px / 1fr 1fr 2fr;
width: 400px;
gap: 12px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
width: 100%;
border: 1px solid black;
background-color: greenyellow;
font-weight: bold;
}
Browser Output
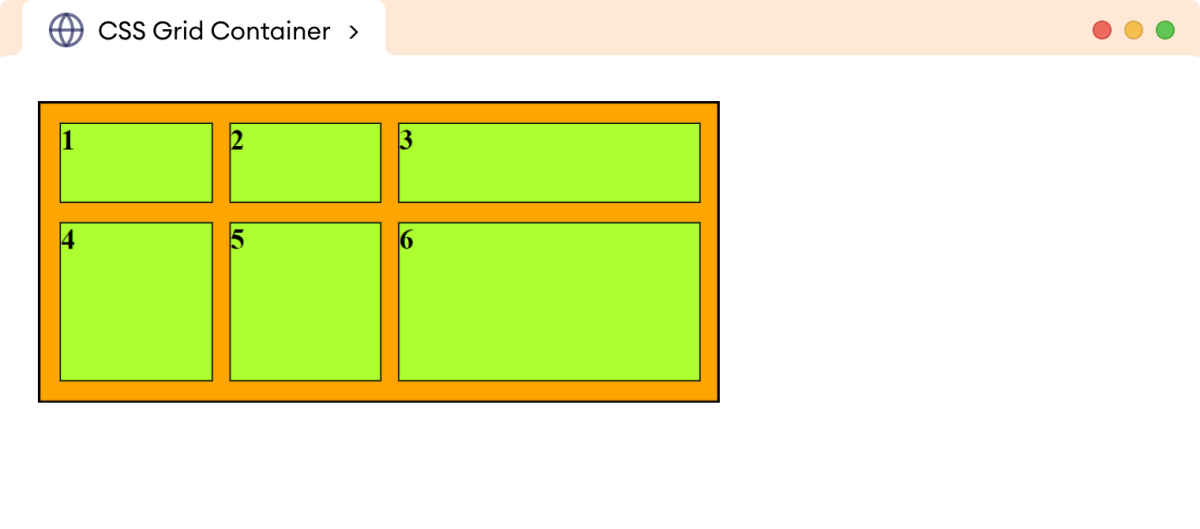
In the above example,
grid: 50px 100px / 1fr 1fr 2fr;
is equivalent to,
grid-template-rows: 50px 100px;
grid-template-columns: 1fr 1fr 2fr;