In SQL, we use the INSERT INTO
statement to insert new row(s) into a database table.
Example
-- insert a row in the Customers table
INSERT INTO Customers(customer_id, first_name, last_name, age, country)
VALUES
(7, 'Ron', 'Weasley', 31, 'UK');
Here, the SQL command inserts a new row into the Customers table with the given values.
INSERT INTO Syntax
INSERT INTO table_name(column1, column2, column3, ...)
VALUES
(value1, value2, value3, ...);
Here,
table_name
is the table where the new row(s) are insertedcolumn1, column2, column3, ...
are the columns where the values are to be insertedvalue1, value2, value3, ...
are the values to be inserted
Example: Insert Row Into a Table
In SQL, the INSERT INTO
statement is used to insert new row(s) into a database table.
-- insert a row in the Customers table
INSERT INTO Customers(customer_id, first_name, last_name, age, country)
VALUES
(5, 'Harry', 'Potter', 31, 'USA');
Here, the SQL command inserts a new row into the Customers table with the given values.
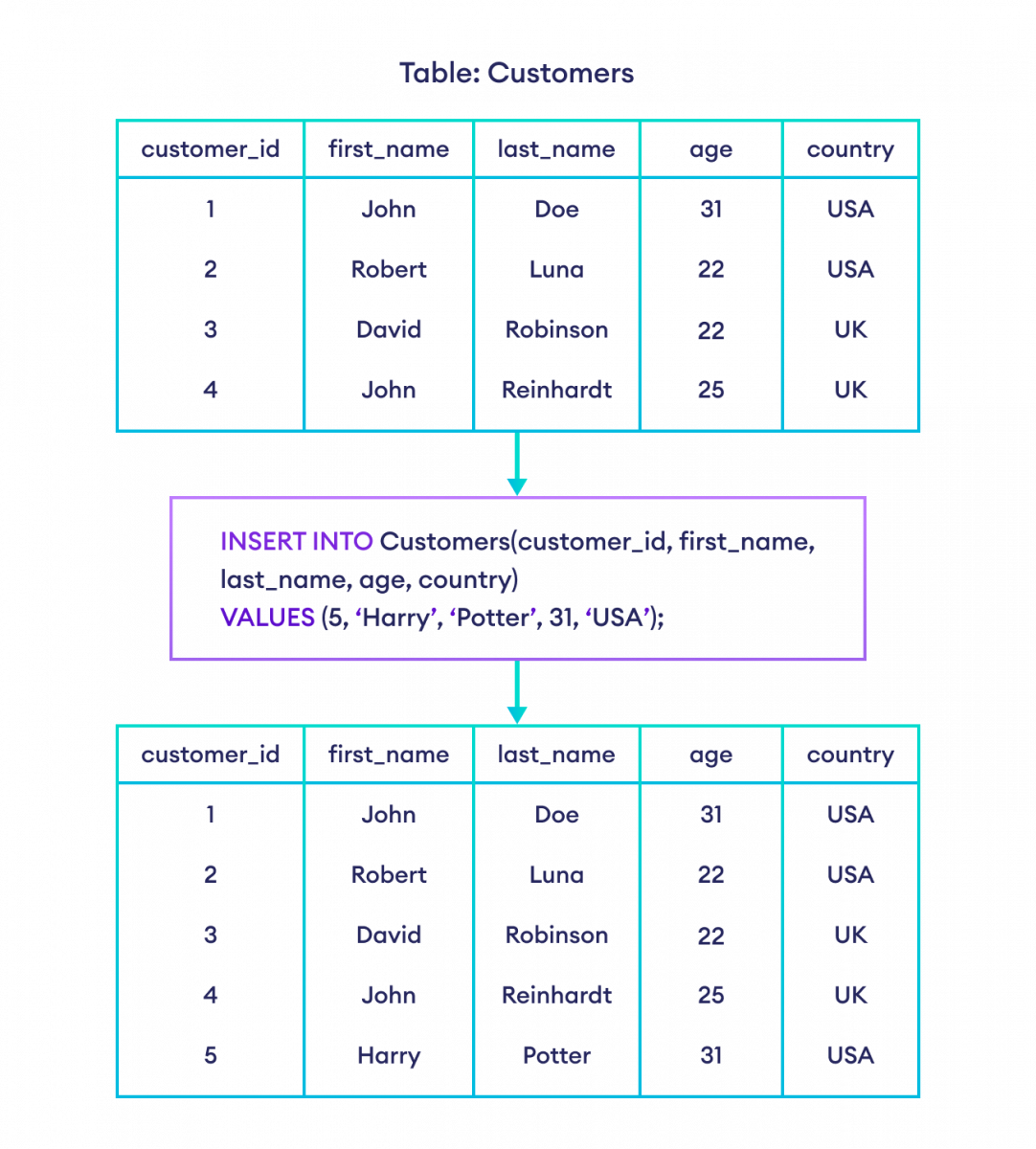
Note: If you want to insert rows from any other existing table, you can use the SQL INSERT INTO SELECT statement.
It is also possible to insert values in a row without specifying columns. For example,
INSERT INTO Customers
VALUES
(5,'Harry', 'Potter', 31, 'USA');
Here, the SQL command inserts the new row serially in each column.
Note: If we don't specify column names, the order of columns in the database table must match the order of values in the SQL query. We also need to provide the value(s) for the auto-incremented field.
Insert Data Only in Specified Column
If we skip column names during row insertion, the values of those columns will be NULL.
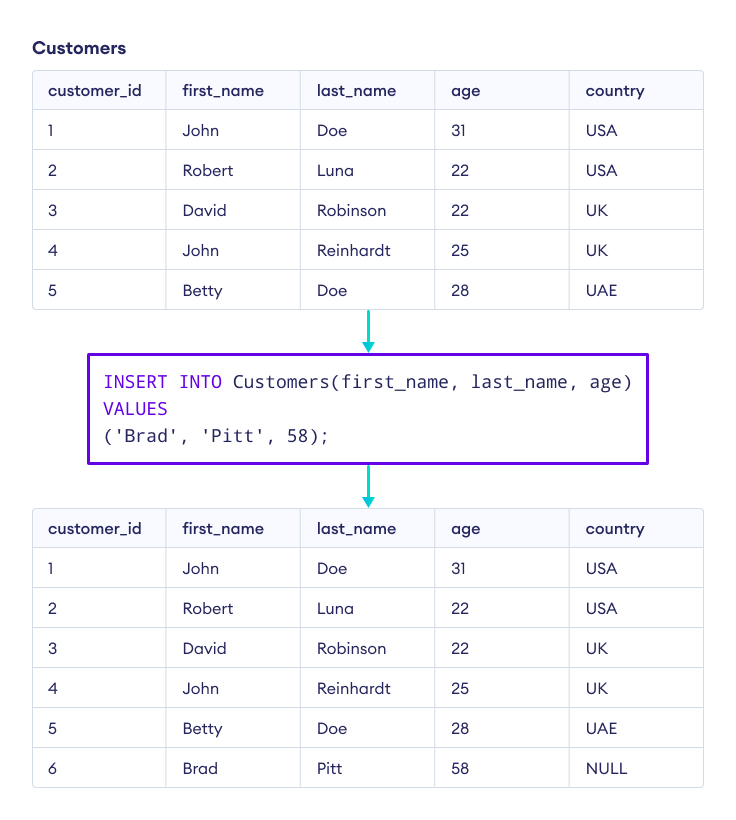
Here, the SQL command sets the country
column's value to NULL. However, the customer_id
column is auto-incremented because of the auto-increment constraint.
Note: If NULL
values are not allowed for a column, the SQL query results in an error. To learn more, visit NOT NULL Constraint.
Example: Insert Multiple Rows at Once in SQL
It's also possible to insert multiple rows into a database table at once. For example,
INSERT INTO Customers(first_name, last_name, age, country)
VALUES
('Harry', 'Potter', 31, 'USA'),
('Chris', 'Hemsworth', 43, 'USA'),
('Tom', 'Holland', 26, 'UK');
Here is the result of the code.
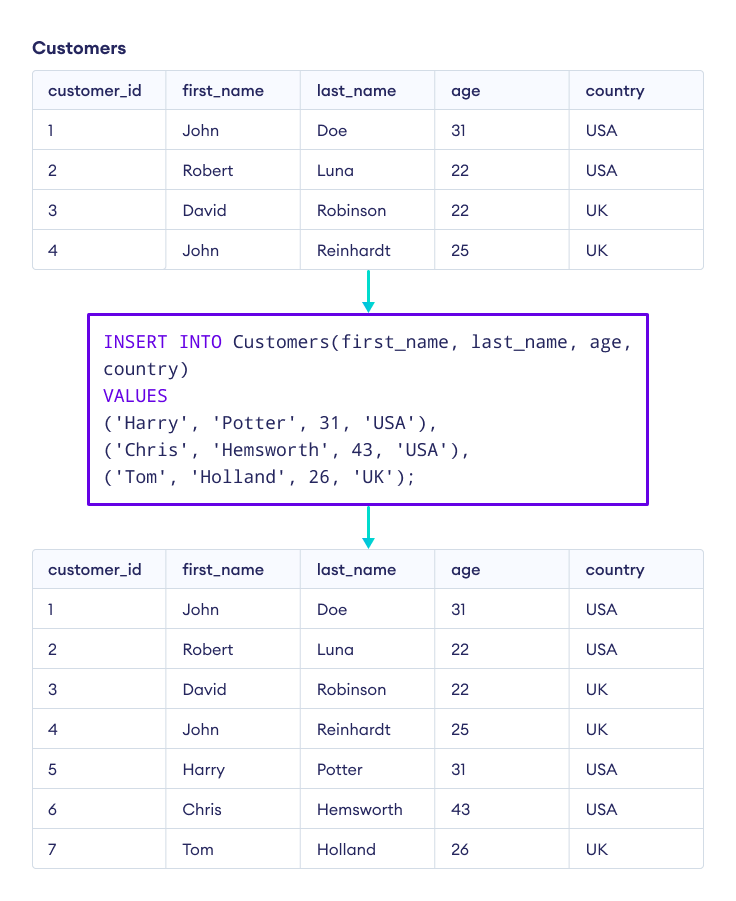
Here, the SQL command inserts three rows to the Customers table.
Also Read: