Slice is a collection of similar types of data, just like arrays.
However, unlike arrays, slice doesn't have a fixed size. We can add or remove elements from the array.
Create slice in Golang
Here's how we create a slice in Golang:
numbers := []int{1, 2, 3, 4, 5}
Here,
numbers
- the name of the sliceint
- represents that the slice only includes integer numbers{1, 2, 3, 4, 5}
- elements of the slice
You might have noticed that we have left the []
notation empty. This is because we can add elements to a slice that will change its size.
Note: If we provide size inside the []
notation, it becomes an array. For example,
// this is an array
numbers := [5]int{1, 2, 3, 4, 5}
// this is a slice
numbers := []int{1, 2, 3, 4, 5}
Example Golang Slice
// Program to create a slice and print its elements
package main
import "fmt"
func main() {
// declare slice variable of type integer
numbers := []int{1, 2, 3, 4, 5}
// print the slice
fmt.Println("Numbers: ", numbers)
}
Output
Numbers: [1 2 3 4 5]
In the above example, we have created a slice named numbers. Here, int
specifies that the slice numbers can only store integers.
Note: We can also create a slice using the var
keyword. For example,
var numbers = []int{1, 2, 3, 4}
Create slice from an array in Golang
In Go programming, we can also create a slice from an existing array. For example,
Suppose we have an array of numbers
numbers := [8]int{10, 20, 30, 40, 50, 60, 70, 80}
Now, we can slice the specified elements from this array to create a new slice.
sliceNumbers = numbers[4, 7]
Here,
- 4 is the start index from where we are slicing the array element
- 7 is the end index up to which we want to get array elements
While creating a slice from an array, the start index is inclusive and the end index is exclusive. Hence, our slice will include elements [50, 60, 70]
.
Example: Create Slice from Array in Go
// Program to create a slice from an array
package main
import "fmt"
func main() {
// an integer array
numbers := [8]int{10, 20, 30, 40, 50, 60, 70, 80}
// create slice from an array
sliceNumbers := numbers[4 : 7]
fmt.Println(sliceNumbers)
}
Output
[50, 60, 70]
Slice Functions
In Go, slice provides various built-in functions that allow us to perform different operations on a slice.
Functions | Descriptions |
---|---|
append() |
adds element to a slice |
copy() |
copy elements of one slice to another |
Equal() |
compares two slices |
len() |
find the length of a slice |
Adds Element to a Slice
We use the append()
function to add elements to a slice. For example,
// Program to add elements to a slice
package main
import "fmt"
func main() {
primeNumbers := []int{2, 3}
// add elements 5, 7 to the slice
primeNumbers = append(primeNumbers, 5, 7)
fmt.Println("Prime Numbers:", primeNumbers)
}
Output
Prime Numbers: [2 3 5 7]
Here, the code
primeNumbers = append(primeNumbers, 5, 7)
adds elements 5 and 7 to the primeNumbers.
We can also add all elements of one slice to another slice using the append()
function. For example,
// Program to add elements of one slice to another
package main
import "fmt"
func main() {
// create two slices
evenNumbers := []int{2, 4}
oddNumbers := []int{1, 3}
// add elements of oddNumbers to evenNumbers
evenNumbers = append(evenNumbers, oddNumbers...)
fmt.Println("Numbers:", evenNumbers)
}
Output
Numbers: [2 4 1 3]
Here, we have used append()
to add the elements of oddNumbers to the list of evenNumbers elements.
Copy Golang Slice
We can use the copy()
function to copy elements of one slice to another. For example,
// Program to copy one slice to another
package main
import "fmt"
func main() {
// create two slices
primeNumbers := []int{2, 3, 5, 7}
numbers := []int{1, 2, 3}
// copy elements of primeNumbers to numbers
copy(numbers, primeNumbers)
// print numbers
fmt.Println("Numbers:", numbers)
}
Output
Numbers: [2 3 5]
In the above example, we are copying elements of primeNumbers to numbers.
copy(numbers, primeNumbers)
Here, only the first 3 elements of primeNumbers are copied to numbers. This is because the size of numbers is 3 and it can only hold 3 elements.
The copy()
function replaces all the elements of the destination array with the elements.
Find the Length of a Slice
We use the len()
function to find the number of elements present inside the slice. For example,
// Program to find the length of a slice
package main
import "fmt"
func main() {
// create a slice of numbers
numbers := []int{1, 5, 8, 0, 3}
// find the length of the slice
length := len(numbers)
fmt.Println("Length:", numbers)
}
Output
Length: 5
Looping through Go Slice
In Go, we can use a for
loop to iterate through a slice. For example,
// Program that loops over a slice using for loop
package main
import "fmt"
func main() {
numbers := []int{2, 4, 6, 8, 10}
// for loop that iterates through the slice
for i := 0; i < len(numbers); i++ {
fmt.Println(numbers[i])
}
}
Output
2 4 6 8 10
Here, len()
function returns the size of a slice. The size of a slice numbers is 5, so the for
loop iterates 5 times.
Frequently Asked Questions
In Go, each element in a slice is associated with a number. The number is known as a slice index.
We can access elements of a slice using the index number (0, 1, 2 …). For example,
// Program to access the slice elements
package main
import "fmt"
func main() {
languages := []string{"Go", "Java", "C++"}
// access element at index 0
fmt.Println(languages[0])
// access element at index 2
fmt.Println(languages[2])
// access elements from index 0 to index 2
fmt.Println(languages[0:3])
}
Output
Go C++ [Go Java C++]
In the above example, we have created a slice named languages.
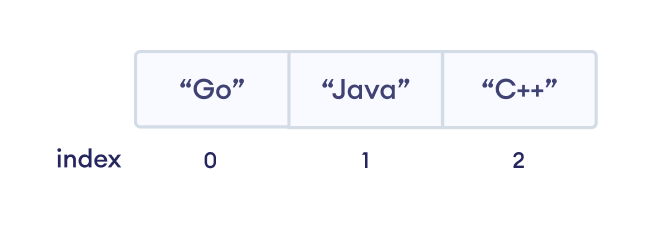
Here, we can see each slice element is associated with the index number. And, we have used the index number to access the elements.
Note: The slice index always starts with 0. Hence, the first element of a slice is present at index 0, not 1
We can use the same index number to change the value of a slice element. For example,
package main
import "fmt"
func main() {
weather := []string{"Rainy", "Sunny", "Cloudy"}
//change the element of index 2
weather[2] = "Stromy"
fmt.Println(weather)
}
Output
[Rainy Sunny Stromy]
Here, the value of index 2 changed from "Cloudy" to "Stromy".
In Go, we can also create a slice using the make()
function. For example,
numbers := make([]int, 5, 7)
Here, we have created a slice of integer type.
- 5 is the length of the slice (number of elements present in the slice)
- 7 is the capacity of the slice (maximum size up to which a slice can be extended)
Let's see an example,
package main
import "fmt"
func main() {
// create a slice using make()
numbers := make([]int, 5, 7)
// add elements to numbers
numbers[0] = 13
numbers[1] = 23
numbers[2] = 33
fmt.Println("Numbers:", numbers)
}
Output
Numbers: [13 23 33 0 0]
Here, we have created the slice with length 5. However, we have only initialized 3 values to the slice.
In this case, the remaining value is initialized with the default value 0.
Apart from using for loop, we can also use for range
to loop through a slice in Golang. For example,
// Program that loops over a slice using for range loop
package main
import "fmt"
func main() {
numbers := []int{2, 4, 6, 8, 10}
// for range loop that iterates through a slice
for _, value := range numbers {
fmt.Println(value)
}
}
Output
2 4 6 8 10
Here, the for range
loop iterates through the first element of the slice (2) to the last element(10). To learn about range, visit Go range.