In programming, a loop is used to repeat a block of code. For example,
If we want to print a statement 100 times, instead of writing the same print statement 100 times, we can use a loop to execute the same code 100 times.
This is just a simple example; we use for
loops to clean and simplify complex programs.
Golang for loop
In Golang, we use the for
loop to repeat a block of code until the specified condition is met.
Here's the syntax of the for
loop in Golang.
for initialization; condition; update {
statement(s)
}
Here,
- The initialization initializes and/or declares variables and is executed only once.
- Then, the condition is evaluated. If the condition is
true
, the body of the for loop is executed. - The update updates the value of initialization.
- The condition is evaluated again. The process continues until the condition is
false
. - If the condition is
false
, the for loop ends.
Working of for loop
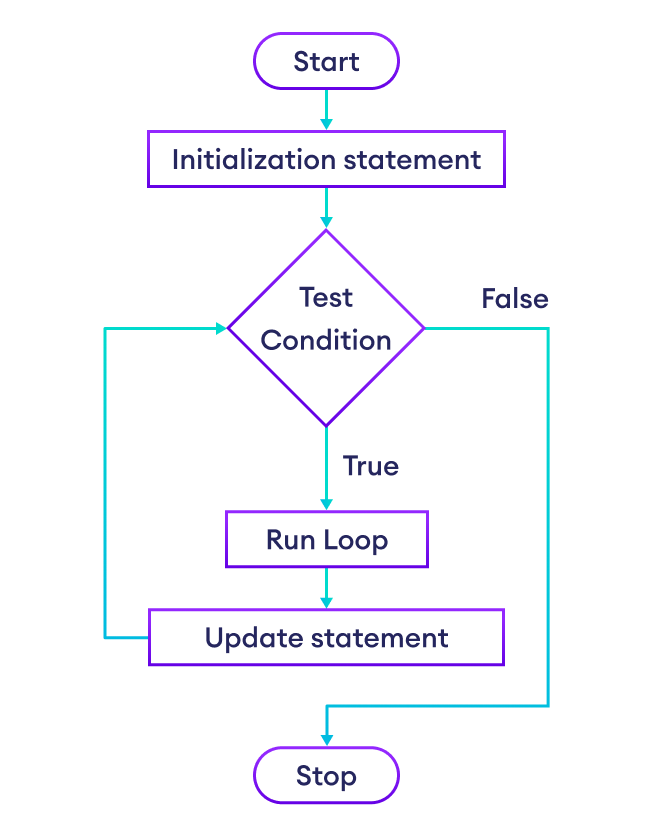
Example 1: Golang for loop
// Program to print the first 5 natural numbers
package main
import "fmt"
func main() {
// for loop terminates when i becomes 6
for i := 1; i <= 5; i++ {
fmt.Println(i)
}
}
Output
1 2 3 4 5
Here is how this program works.
Iteration | Variable | Condition: i | Action |
---|---|---|---|
1st | i = 1 |
true |
1 is printed.i is increased to 2. |
2nd | i = 2 |
true |
2 is printed.i is increased to 3. |
3rd | i = 3 |
true |
3 is printed.i is increased to 4. |
4th | i = 4 |
true |
4 is printed.i is increased to 5. |
5th | i = 5 |
true |
5 is printed.i is increased to 6. |
6th | i = 6 |
false |
The loop is terminated. |
Example 2: Golang for loop
// Program to print numbers for natural numbers 1 + 2 + 3 + ... +n
package main
import "fmt"
func main() {
var n, sum = 10, 0
for i := 1 ; i <= n; i++ {
sum += i // sum = sum + i
}
fmt.Println("sum =", sum)
}
Output
sum = 55
Here, we have used a for
loop to iterate from i equal to 1 to 10.
In each iteration of the loop, we have added the value of i to the sum
variable.
Related Golang for Loop Topics
In Go, we can use range with for
loop to iterate over an array. For example,
package main
import "fmt"
func main() {
// create an array
numbers := [5] int {11, 22, 33, 44, 55}
// for loop with range
for item := range numbers {
fmt.Println(numbers[item])
}
}
Output
11 22 33 44 55
Here, we have used range
to iterate 5 times (the size of the array).
The value of the item is 11 in the first iteration, 22 in the second iteration, and so on.
To learn more about arrays, visit Golang Arrays
In Golang, for
loop can also be used as a while
loop (like in other languages). For example,
for condition {
statement(s)
}
Here, the for loop only contains the test condition. And, the loop gets executed until the condition is true
. When the condition is false
, the loop terminates.
A loop that never terminates is called an infinite loop.
If the test condition of a loop never evaluates to true, the loop continues forever. For example,
// Program to infinitely iterate through the loop
package main
import "fmt"
func main() {
for i := 0; i <= 10; i-- {
fmt.Println("Welcome to Programiz")
}
}
Here, the condition i <= 10
never evaluates to false
resulting in an infinite loop.
Output
Welcome to Programiz Welcome to Programiz Welcome to Programiz …
If we want to create an infinite loop intentionally, Golang has a relatively simpler syntax for it. Let's take the same example above.
// Program to infinitely iterate through the loop
package main
import "fmt"
func main() {
// for loop without initialization/condition/update
// infinite loop
for {
fmt.Println("Welcome to Programiz")
}
}
In Golang, we have to use every variable that we declare inside the body of the for
loop. If not used, it throws an error. We use a blank identifier _
to avoid this error.
Let's understand it with the following scenario.
numbers := [5] int {11, 22, 33, 44, 55}
// index variable is declared but not used
for index, item := range numbers {
// throws an error
fmt.Println(item)
}
}
Here, we get an error message index declared but not used.
To avoid this, we put _
in the first place to indicate that we don't need the first component of the array( index ). Let's correct the above example with a blank identifier.
// Program to print the elements of the array
package main
import "fmt"
func main() {
numbers := [5] int {11, 22, 33, 44, 55}
for _, item := range numbers {
fmt.Println(item)
}
}
Output
11 22 33 44 55
Here, the program knows that the item indicates the second part of the array.