Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
TypeScript while Loop
The while
loop repeatedly executes a block of code as long as the specified condition is true
.
The syntax of the while
loop is:
while (condition) {
// Body of loop
}
Here,
- The
while
loop first evaluates thecondition
inside( )
. - If
condition
evaluates totrue
, the code inside{ }
is executed. - Then,
condition
is evaluated again. - This process continues as long as
condition
evaluates totrue
. - If
condition
evaluates tofalse
, the loop stops.
Flowchart of while Loop
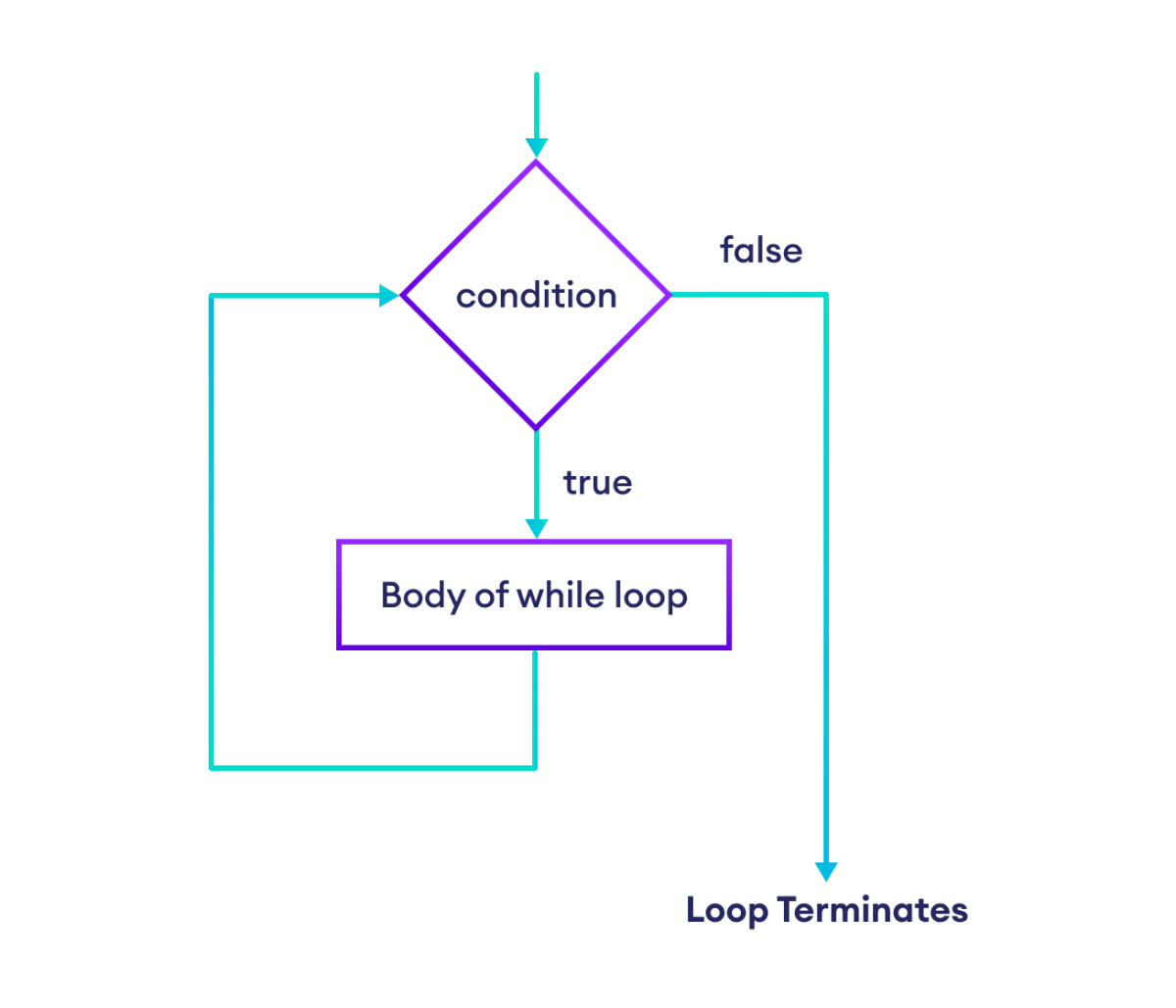
Example 1: Display Numbers From 1 to 3
// Initialize variable i
let i: number = 1;
// Loop runs until i is less than 4
while (i < 4) {
console.log(i);
// Increase value of i by 1
i += 1;
}
Output
1 2 3
Here is how the program works in each iteration of the loop:
Variable | Condition: i | Action |
---|---|---|
i = 1 |
true |
1 is printed. i is increased to 2. |
i = 2 |
true |
2 is printed. i is increased to 3. |
i = 3 |
true |
3 is printed. i is increased to 4. |
i = 4 |
false |
The loop is terminated. |
To learn more about loop conditions, visit TypeScript Comparison and Logical Operators.
Example 2: Sum of Only Positive Numbers
let inputNumber: number = 0;
let sum: number = 0;
// Create a variable to take user input
// The variable can be either string or null
let userInput: string | null;
// Loop as long as inputNumber is not negative
while (inputNumber >= 0) {
// Add all positive numbers
sum += inputNumber;
// Take input from the user
// prompt() returns either string or null
userInput = prompt("Enter a number: ");
// Convert userInput to number
inputNumber = Number(userInput);
}
// Display the sum outside the loop
console.log(`The sum is ${sum}`);
Output
Enter a number: 2 Enter a number: 4 Enter a number: -3 The sum is 6
The above program prompts the user to enter a number.
Since TypeScript prompt()
only takes inputs as string, we use the Number()
function to convert the input into a number.
Note: prompt()
returns null
if the user doesn't give any input (by pressing the Cancel button). In this case, pressing Cancel will exit the loop as null
will not be converted to a valid number.
As long as we enter positive numbers, the while
loop adds them up and prompts us to enter more numbers.
So when we enter a negative number, the loop terminates.
Finally, we display the total sum of positive numbers outside the loop.
Note: When we add two or more numeric strings, TypeScript treats them as strings. For example, "2" + "3" = "23"
. So, we should always convert numeric strings to numbers to avoid unexpected behaviors.
TypeScript do...while Loop
The do...while
loop executes a block of code once, then repeatedly executes it as long as the specified condition is true
.
The syntax of the do...while
loop is:
do {
// Body of loop
} while(condition);
Here,
- The
do…while
loop executes the code inside{ }
. - Then, it evaluates the
condition
inside( )
. - If
condition
evaluates totrue
, the code inside{ }
is executed again. - This process continues as long as
condition
evaluates totrue
. - If
condition
evaluates tofalse
, the loop terminates.
Flowchart of do...while Loop
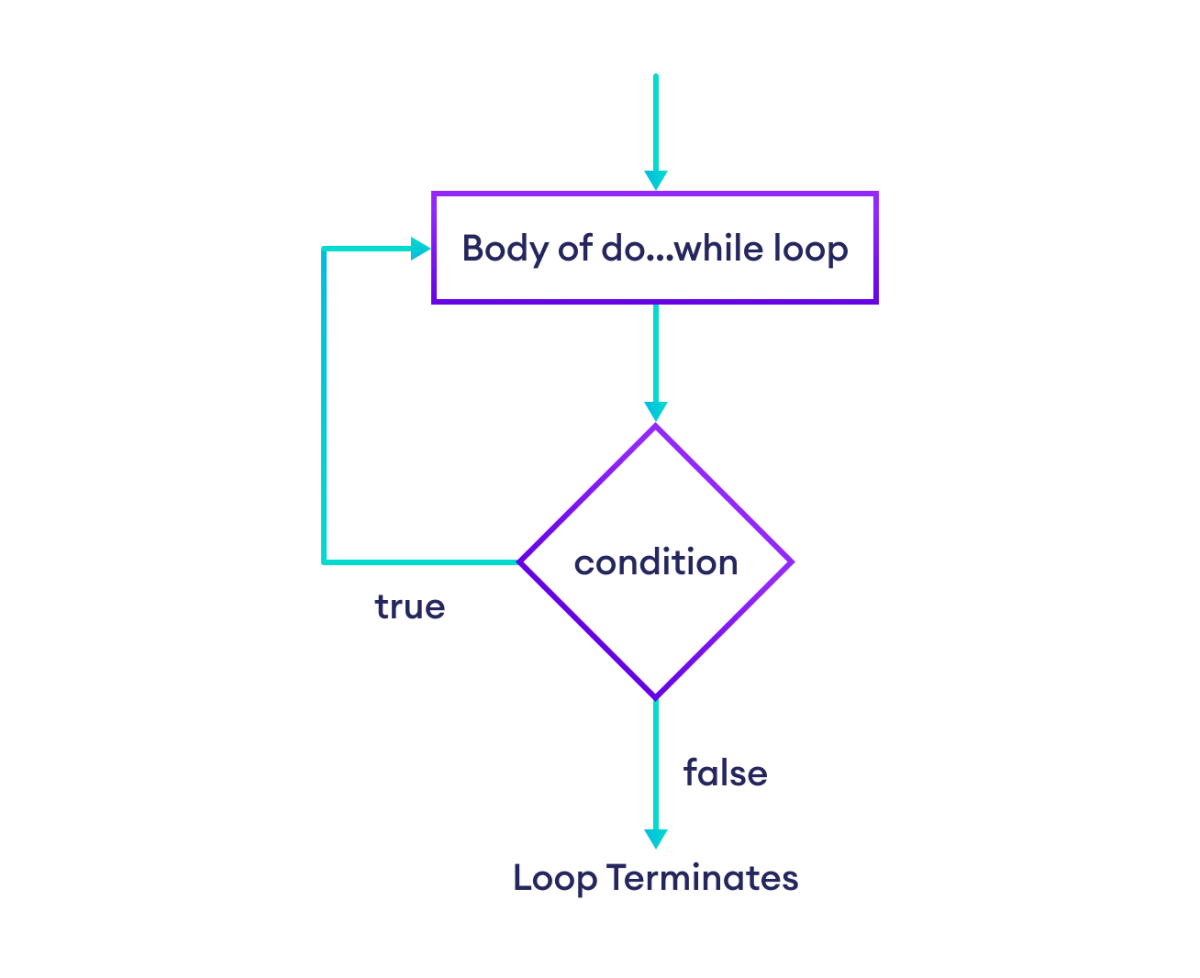
Example 3: Display Numbers from 3 to 1
let i: number = 3;
// Loop body is executed first
// Then, loop continues as long as i is positive
do {
console.log(i);
// Decrement value of i by 1
i--;
} while (i > 0);
Output
3 2 1
Here, the initial value of i is 3. Then, we used a do...while
loop to iterate over the values of i. Here's how the loop works in each iteration:
Action | Variable | Condition: i > 0 |
---|---|---|
3 is printed. i is decreased to 2. | i = 2 |
true |
2 is printed. i is decreased to 1. | i = 1 |
true |
1 is printed. i is decreased to 0. | i = 0 |
false |
The loop is terminated. | - | - |
Difference between while and do...while Loops
The difference between while
and do...while
is that the do...while
loop executes its body at least once. For example,
let i: number = 5;
// False condition
// Body executes once
do {
console.log(i);
} while (i > 10);
// Output: 5
On the other hand, the while
loop doesn't execute its body if the loop condition is false
. For example,
let i: number = 5;
// False condition
// Body not executed
while (i > 10) {
console.log(i);
};
Example 4: Sum of Positive Numbers
let inputNumber: number = 0;
let sum: number = 0;
// Create a variable to take user input
// The variable can be either string or null
let userInput: string | null;
do {
// Add all positive numbers
sum += inputNumber;
// Take input from the user
userInput = prompt("Enter a number: ");
// Convert userInput to number
inputNumber = Number(userInput);
// Loop terminates if inputNumber is negative
} while (inputNumber >= 0);
// Finally, display the sum
console.log(`The sum is ${sum}`);
Output
Enter a number: 2 Enter a number: 4 Enter a number: -3 The sum is 6
In the above program, the do...while
loop prompts the user to enter a number.
As long as we enter positive numbers, the loop adds them up and prompts us to enter more numbers.
If we enter a negative number, the loop terminates without adding the negative number.
More on TypeScript while and do...while Loops
An infinite while
loop is a condition where the loop runs infinitely, as its condition is always true
. For example,
let i: number = 1;
// Condition is always true
while(i < 5) {
console.log(i);
}
Also, here is an example of an infinite do...while
loop:
let i: number = 1;
// Condition is always true
do {
console.log(i);
} while (i < 5);
In the above programs, the condition i < 5
is always true
, which causes the loop body to run forever.
Note: Infinite loops can cause your program to crash. So, avoid creating them unintentionally.
1. for Loop
We use a for
loop when we know the exact number of iterations required. For example,
// Display "hi" 3 times
for (let i: number = 1; i <= 3; i++) {
console.log("hi");
}
console.log("bye");
Output
hi hi hi bye
Here, we know that we need to print "hi"
three times. So, we've used a for
loop instead of a while
loop.
2. while Loop
Meanwhile, we use a while
loop when the termination condition can vary. For example,
// Boolean variable to use as condition
let isDisplay: boolean = true;
// Variable to take user input from prompt()
// Can be either string or null
let userChoice: string | null;
// Display "hi" as long as user wants
// Basically, run the loop as long as isDisplay is true
while (isDisplay) {
console.log("hi");
// Get user input
userChoice = prompt("print hi again? y for yes: ");
// Set isDisplay to false if userChoice is not "y" or if it's null
if (userChoice != "y" || userChoice === null)
isDisplay = false;
}
console.log("bye");
Output
hi print hi again? y for yes: y hi print hi again? y for yes: y hi print hi again? y for yes: n bye
In the above program, we let the user print "hi"
as much as they desire.
Since we don't know the user's decision, we use a while
loop instead of a for
loop.
Also Read: