In computer programming, we use the if
statement to run a block code only when a certain condition is met.
For example, assigning grades (A, B, C) based on marks obtained by a student.
- if the percentage is above 90, assign grade A
- if the percentage is above 75, assign grade B
- if the percentage is above 65, assign grade C
In Swift, there are three forms of the if...else
statement.
if
statementif...else
statementif...else if...else
statement
1. Swift if Statement
The syntax of if
statement in Swift is:
if (condition) {
// body of if statement
}
The if
statement evaluates condition
inside the parenthesis ()
.
- If
condition
is evaluated totrue
, the code inside the body ofif
is executed. - If
condition
is evaluated tofalse
, the code inside the body ofif
is skipped.
Note: The code inside { }
is the body of the if
statement.
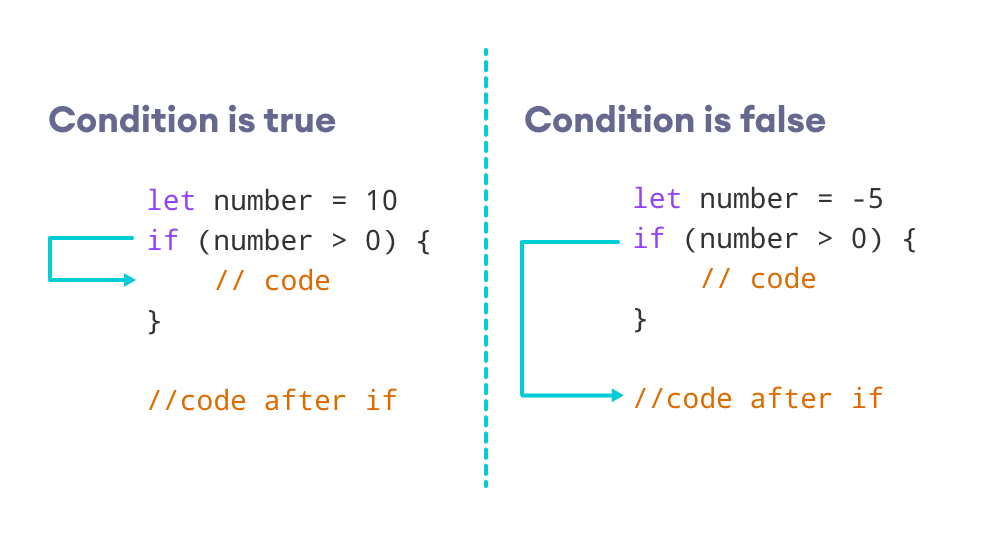
Example 1: if Statement
let number = 10
// check if number is greater than 0
if (number > 0) {
print("Number is positive.")
}
print("The if statement is easy")
Output
Number is positive. The if statement is easy
In the above example, we have created a variable named number
. Notice the test condition,
number > 0
Here, since number is greater than 0, the condition evaluates true
.
If we change the variable to a negative integer. Let's say -5.
let number = -5
Now, when we run the program, the output will be:
The if statement is easy
This is because the value of number
is less than 0. Hence, the condition evaluates to false
. And, the body of if
block is skipped.
2. Swift if...else Statement
An if
statement can have an optional else
clause.
The syntax of if-else
statement is:
if (condition) {
// block of code if condition is true
}
else {
// block of code if condition is false
}
The if...else
statement evaluates the condition
inside the parenthesis.
If the condition evaluates to true,
- the code inside
if
is executed - the code inside
else
is skipped
If the condition evaluates to false,
- the code inside
else
is executed - the code inside
if
is skipped
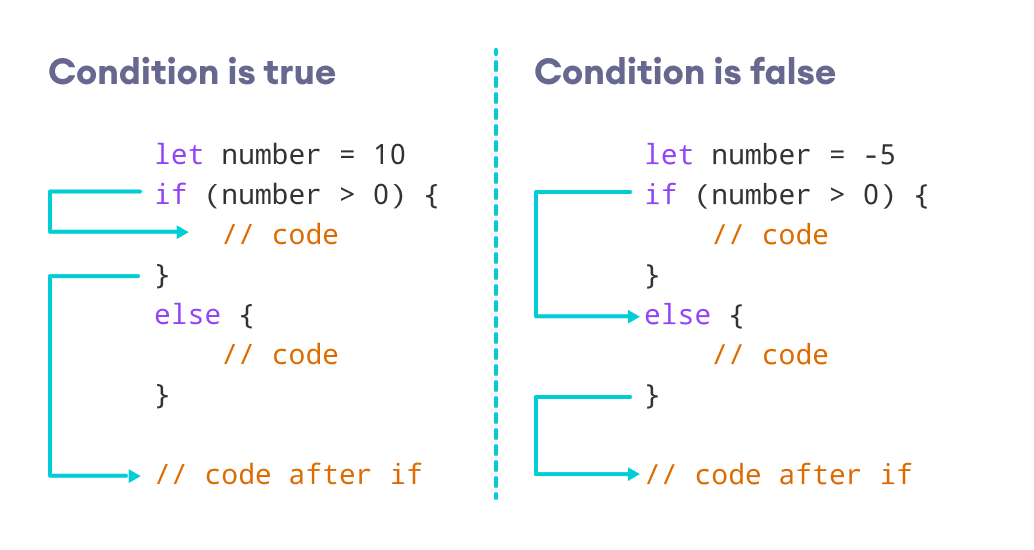
Example 2: Swift if...else Statement
let number = 10
if (number > 0) {
print("Number is positive.")
}
else {
print("Number is negative.")
}
print("This statement is always executed.")
Output
Number is positive. This statement is always executed.
In the above example, we have created a variable named number
. Here, the test expression
number > 0
Since the value of number
is 10
, the test expression evaluates to true
. Hence code inside the body of if
is executed.
If we change the variable to a negative integer. Let's say -5.
let number = -5
Now if we run the program, the output will be:
Number is negative. This statement is always executed.
Here, the test expression evaluates to false
. Hence code inside the body of else
is executed.
3. Swift if...else if...else Statement
The if...else
statement is used to execute a block of code among two alternatives.
However, if you need to make a choice between more than two alternatives, then we use the if...else if...else
statement.
The syntax of the if...else if...else
statement is:
if (condition1) {
// code block 1
}
else if (condition2){
// code block 2
}
else {
// code block 3
}
Here,
- If condition1 evaluates to
true
, code block 1 is executed. - If condition1 evaluates to
false
, then condition2 is evaluated.- If condition2 is
true
, code block 2 is executed. - If condition2 is
false
, code block 3 is executed.
- If condition2 is
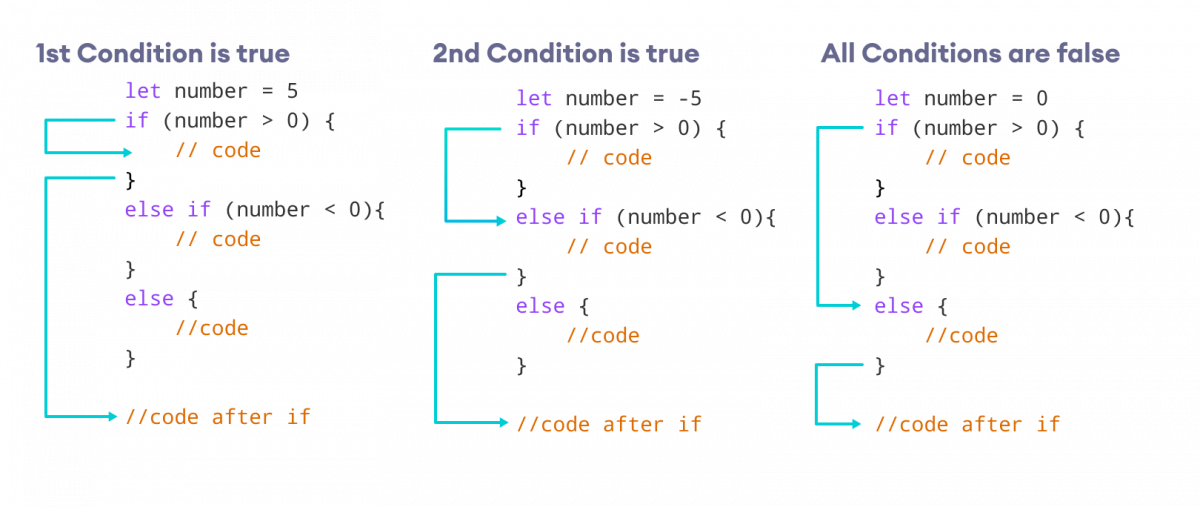
Example 3: Swift if..else if Statement
// check whether a number is positive, negative, or 0.
let number = 0
if (number > 0) {
print("Number is positive.")
}
else if (number < 0) {
print("Number is negative")
}
else {
print("Number is 0.")
}
print("This statement is always executed")
Output
Number is 0.
In the above example, we have created a variable named number with the value 0. Here, we have two condition expressions:
if (number > 0)
- checks ifnumber
is greater than0
else if (number < 0)
- checks ifnumber
is less than0
Here, both the conditions evaluate to false
. Hence the statement inside the body of else
is executed.
Swift nested if Statement
You can also use an if
statement inside of an if
statement. This is known as a nested if statement.
The syntax of nested if statement is:
// outer if statement
if (condition1) {
// statements
// inner if statement
if (condition2) {
// statements
}
}
Notes:
- We can add
else
andelse if
statements to the innerif
statement as required. - We can also insert inner
if
statement inside the outerelse
orelse if
statements(if they exist) - We can nest multiple layers of
if
statements.
Example 4: Nested if...else Statement
var number = 5
// outer if statement
if (number >= 0) {
// inner if statement
if (number == 0) {
print("Number is 0")
}
// inner else statement
else {
print("Number is positive");
}
}
// outer else statement
else {
print("Number is negative");
}
Output
Number is positive
In the above example, we have used a nested if statement to check whether the given number is positive, negative, or 0.