Kotlin is a modern programming language mainly used for Android application development.
You can run Kotlin on your computer using the following two methods:
- Run Kotlin online
- Install Kotlin on your computer
In this tutorial, you will learn both methods.
Run Kotlin Online
To run Kotlin code, you must install the Kotlin Compiler on your system. However, you can use a free online Kotlin compiler if you want to start immediately.
The online editor enables you to run Kotlin code directly in your browser—no installation is required.
Install Kotlin on Your Computer
For those who prefer to install Kotlin on your computer, this guide will walk you through the installation process on Windows, macOS, or Linux (Ubuntu).
To install Kotlin on your Windows, just follow these steps:
- Install VS Code
- Download the Kotlin Compiler Zip File
- Extract the Downloaded File
- Add the Compiler to Your PATH
- Verify the Installation
Here is a detailed explanation of each of the steps:
Step 1: Install VS Code
Go to the VS Code official website and download the Windows installer. Once the download is complete, run the installer and follow the installation process.
Click Finish to complete the installation process.
Step 2: Download the Kotlin Compiler Zip File
Navigate to the Kotlin Command Line documentation page and follow the link to the Kotlin Releases page on GitHub.
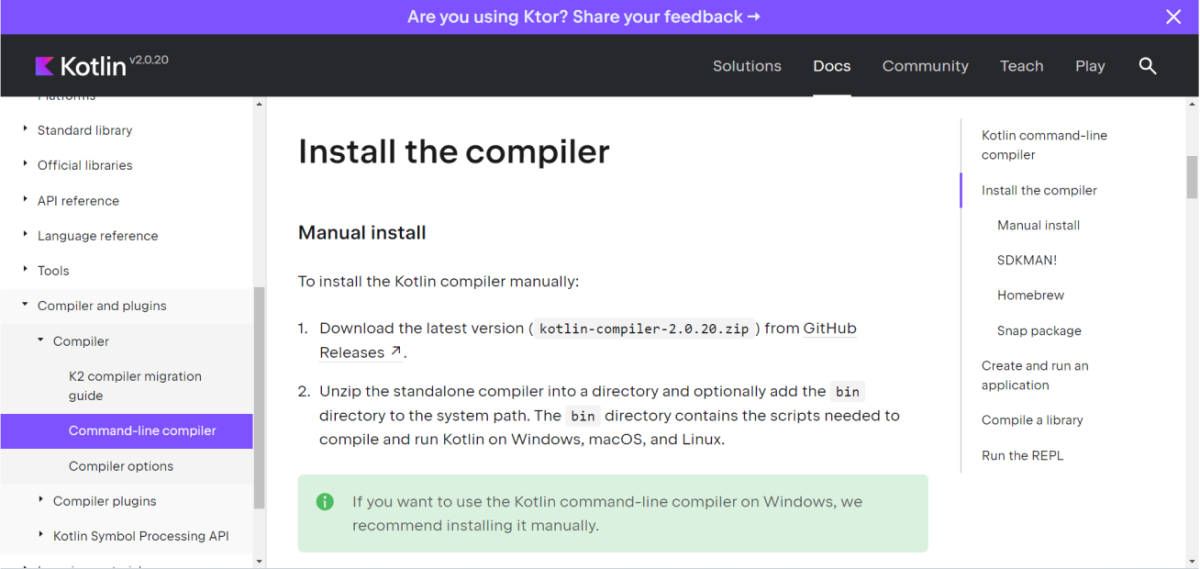
The most recent release is under the "Assets" section. To download it, download a file similar to
kotlin-compiler-x.x.x.zip
, where
x.x.x
represents the version number.
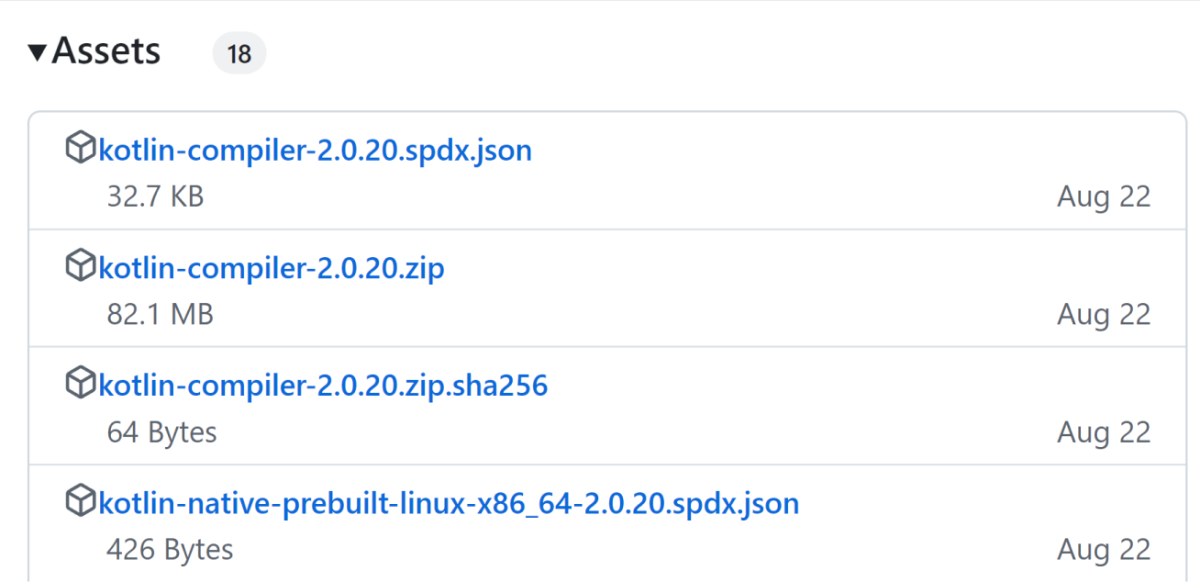
Step 3: Extract the Downloaded File
Once the download is complete, extract the zip file to your preferred location. The zip file contains the Kotlin compiler executables.
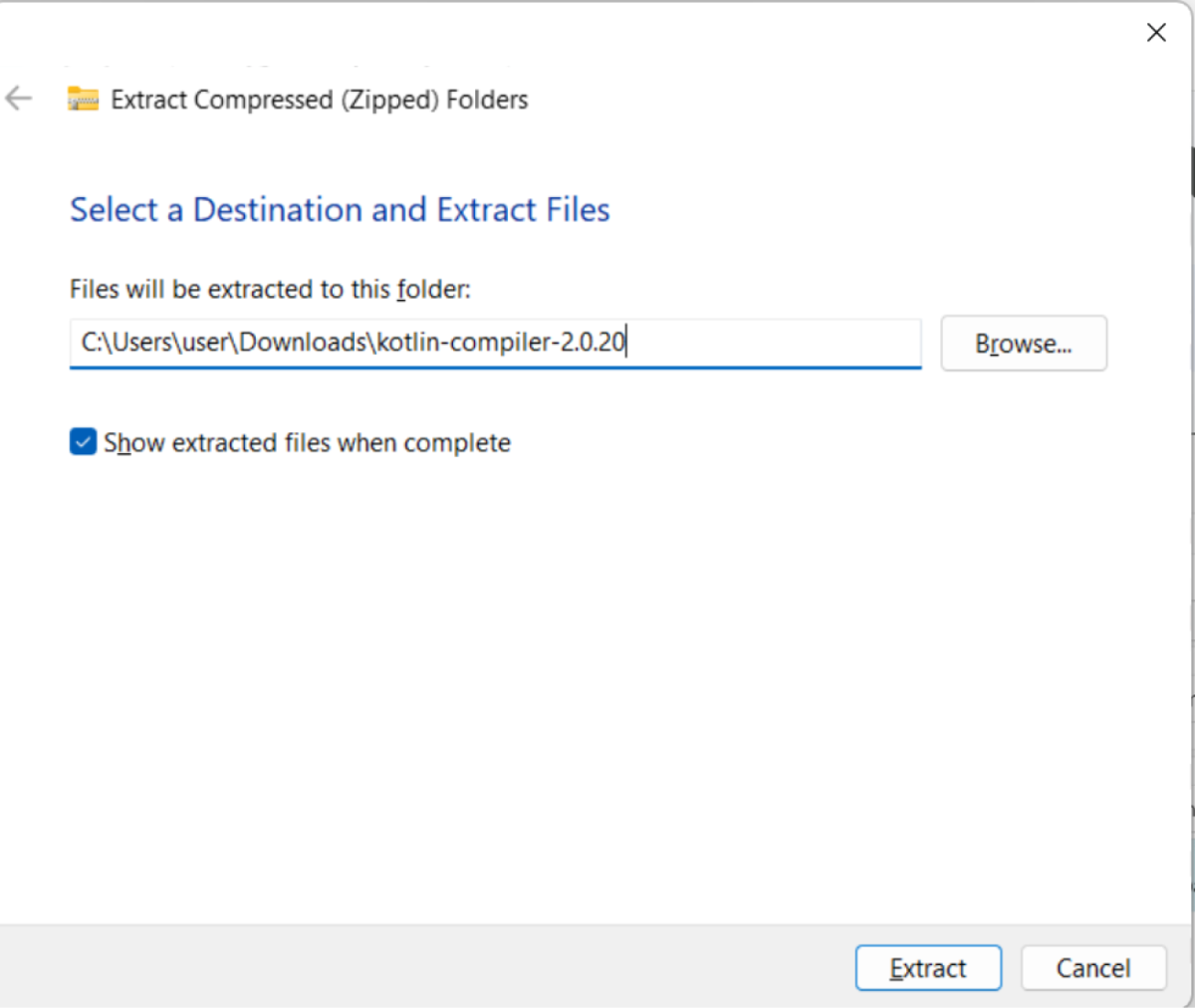
Once the downloaded file is extracted, you will see the following folder.
Step 4: Add the Compiler to Your PATH
To make Kotlin compiler commands available from any command prompt in Windows, add the compiler's directory to the system's PATH environment variable.
Follow these steps to add the PATH environment variable.
1. Copy the path in the Kotlin compiler's bin folder. This is typically found where you extract the
Kotlin compiler. (Here, it is
C:\Users\user\Downloads\kotlin-compiler-2.0.20\kotlinc\bin
)
2. Right-click on This PC and select Properties.
3. Click on Advanced System Settings and then Environment Variables.
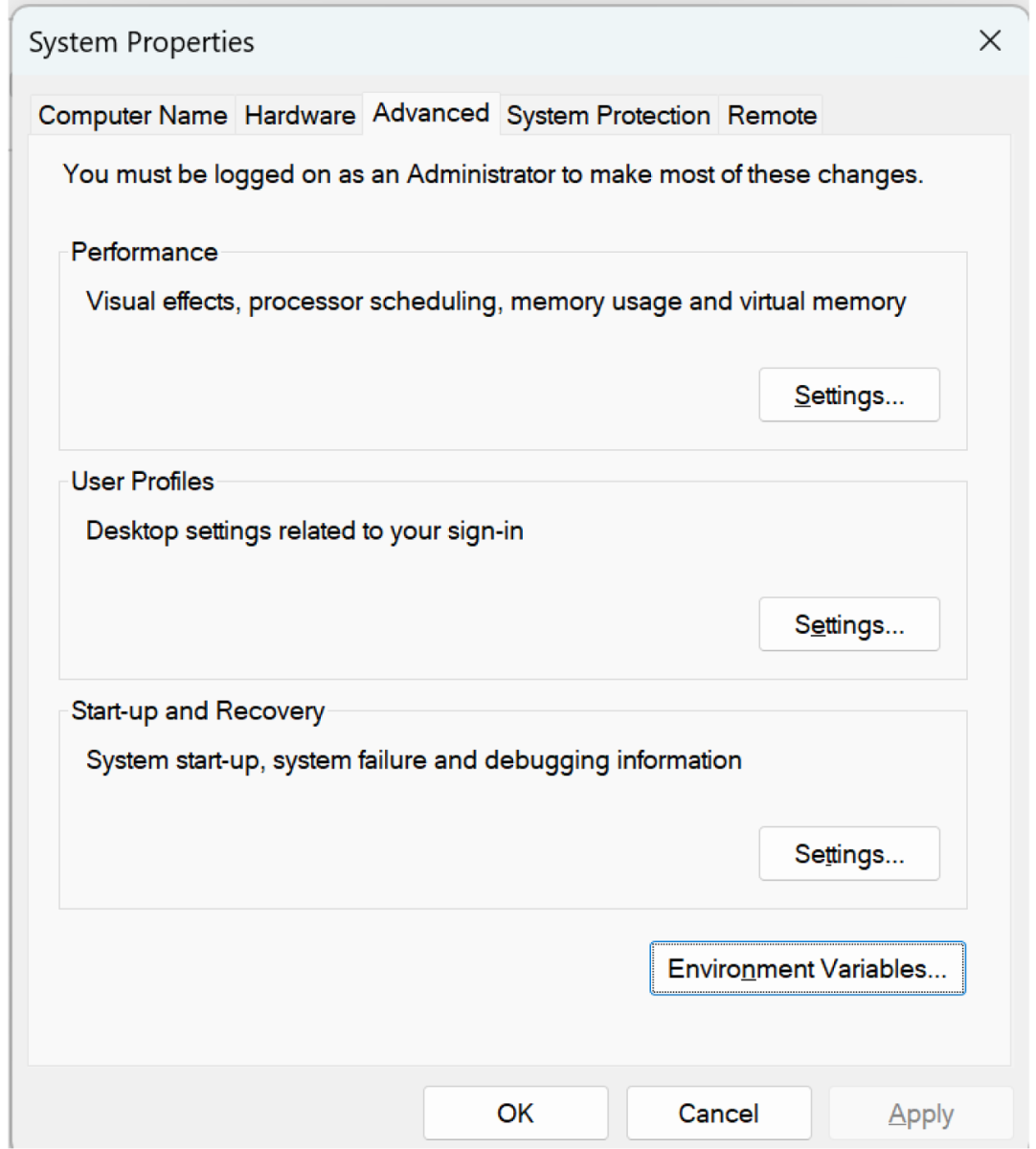
4. Under System Variables, find and select Path, then click Edit.
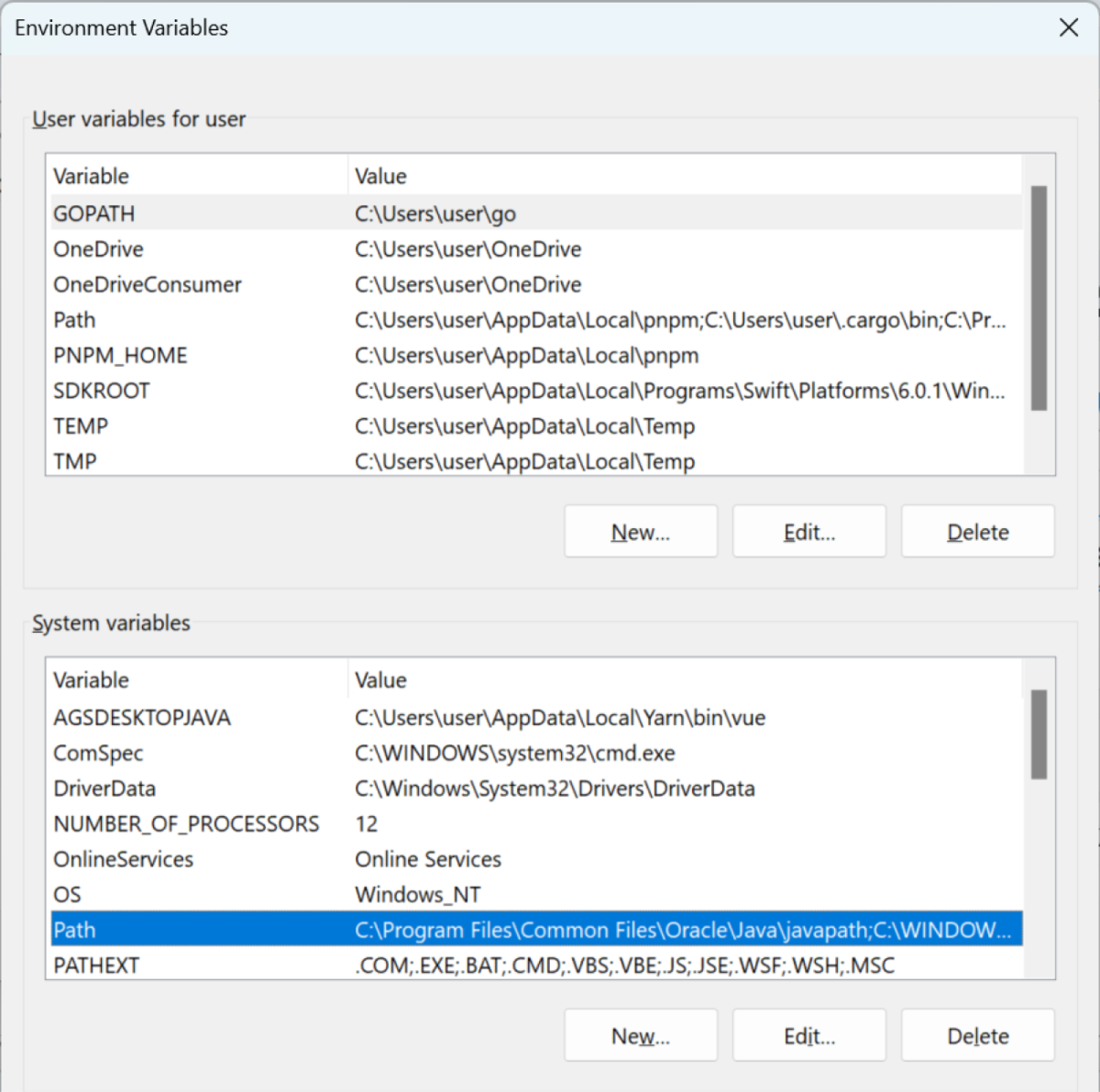
5. Click New and paste the path.
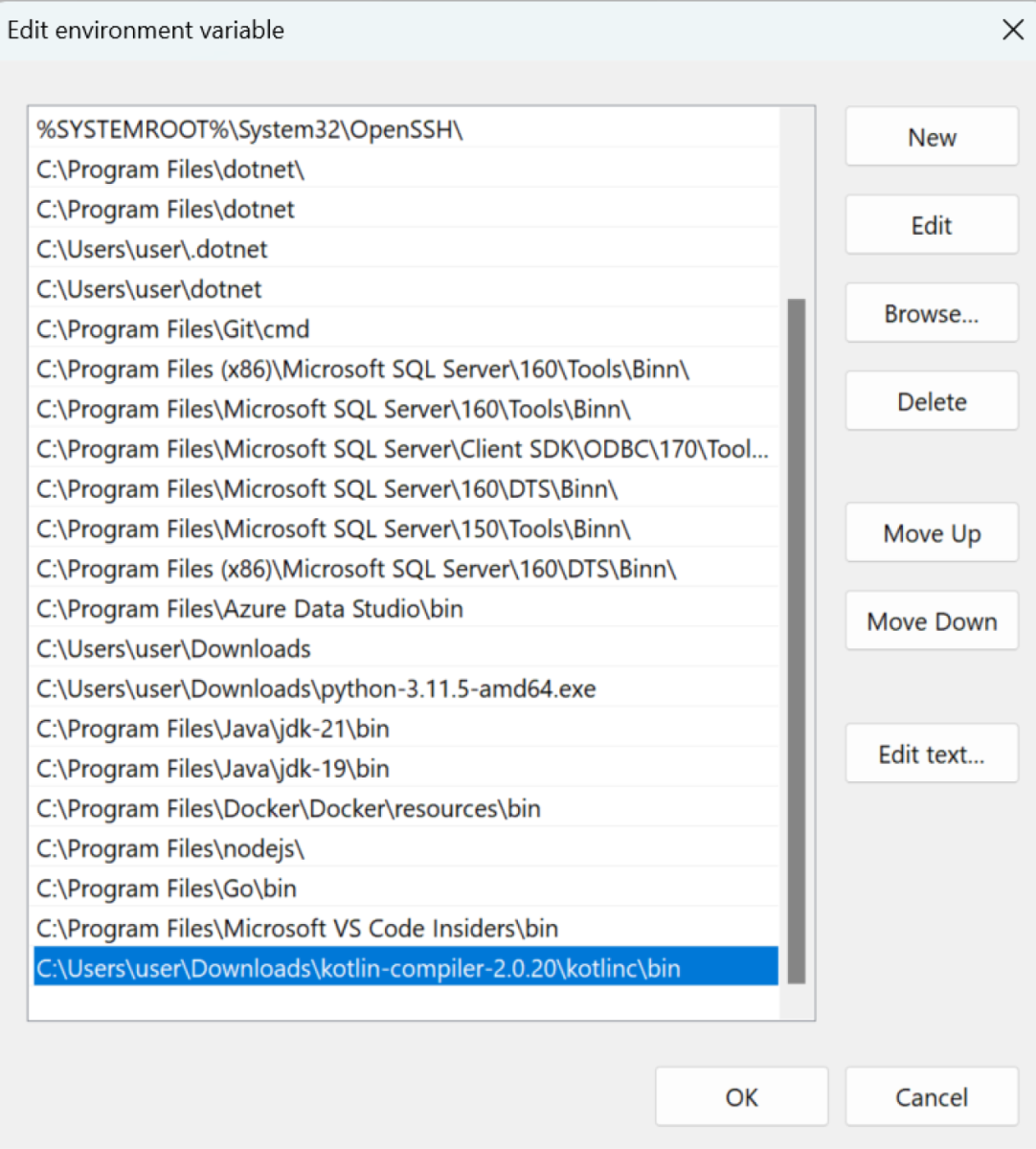
6. Click OK to close all dialogs and apply the changes.
Step 5: Verify the Installation
After installation, open a new Command Prompt window to ensure everything's set up correctly.
Run the following command.
kotlin -version
And it should now display the version you've installed.
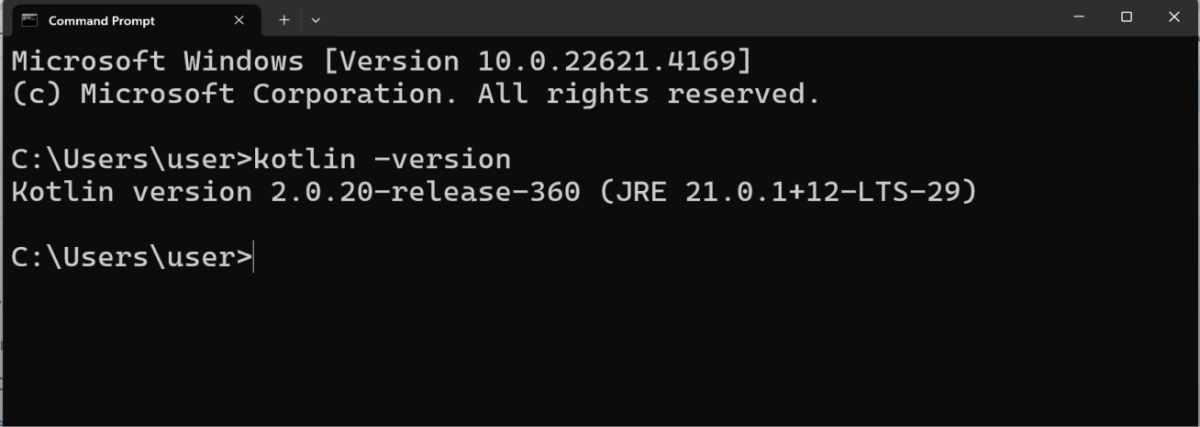
Note: The version number might differ from the one above, depending on your installed version.
Now, you are all set to run Kotlin programs on your device.
To install Kotlin on your Mac, just follow these steps:
- Install VS Code
- Install Homebrew (if not installed)
- Install Kotlin
- Verify the installation.
Here is a detailed explanation of each of the steps:
Step 1: Install VS Code
Go to the VS Code official website and download the zipped file. Once the download is complete, open the zipped file.
In Finder, open a new window and navigate to the Applications folder. To install the VS Code application from the zip file, drag it into the Applications folder.
You can now launch VS Code directly from the Applications folder.
Step 2: Install Homebrew
Homebrew is a package manager that simplifies the installation of software on macOS. Open the Terminal app and type the following command to install Homebrew (if it's not already installed):
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
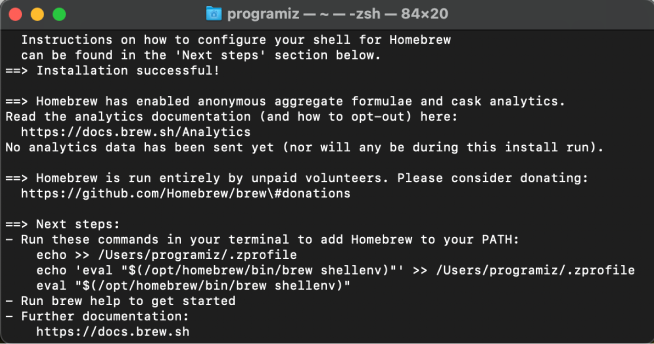
When this installation is complete, we will use homebrew to install Kotlin.
Step 3: Install Kotlin
Now that Homebrew is installed, you can easily install Kotlin. In the Terminal, enter the following command:
brew install kotlin
This command installs the Kotlin compiler and all necessary tools. Once the installation is complete, you can run Kotlin code from the command line.
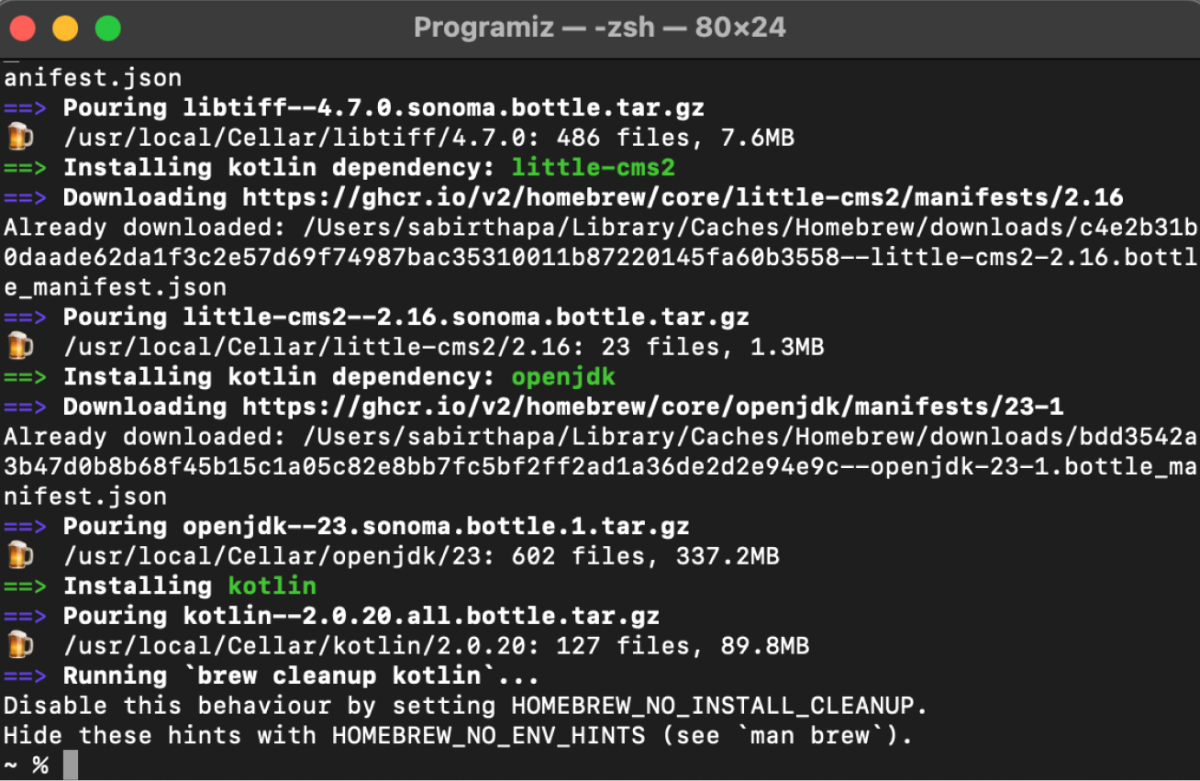
Step 4: Verify the installation
Once all the above processes are complete, exit the current terminal session and open a new one to verify installation.
To verify successful installation, run the following command:
kotlin -version
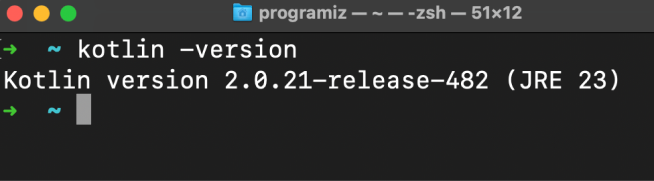
Note: The version number might differ from the one above, depending on your installed version.
Linux has various distributions, and the installation process differs slightly from each other. For now, we will focus on Ubuntu.
To install Kotlin, follow these steps:
- Install VS Code
- Install Curl
- Install SDKMAN
- Install Kotlin
- Verify Installation
Here is a detailed explanation of each of the steps:
Step 1: Install VS Code
Open the Terminal and type:
sudo apt update
This command updates your package lists to ensure you get the latest versions of your software.
Proceed to install VS Code with:
sudo snap install code --classic
Step 2: Install Curl
To download Kotlin, we'd need SDKMAN, and to install SDKMAN, we need Curl.
Download Curl using the following command:
sudo apt install curl

Step 3: Install SDKMAN
To install SDKMAN, run the following command on your terminal.
curl -s "https://get.sdkman.io" | bash
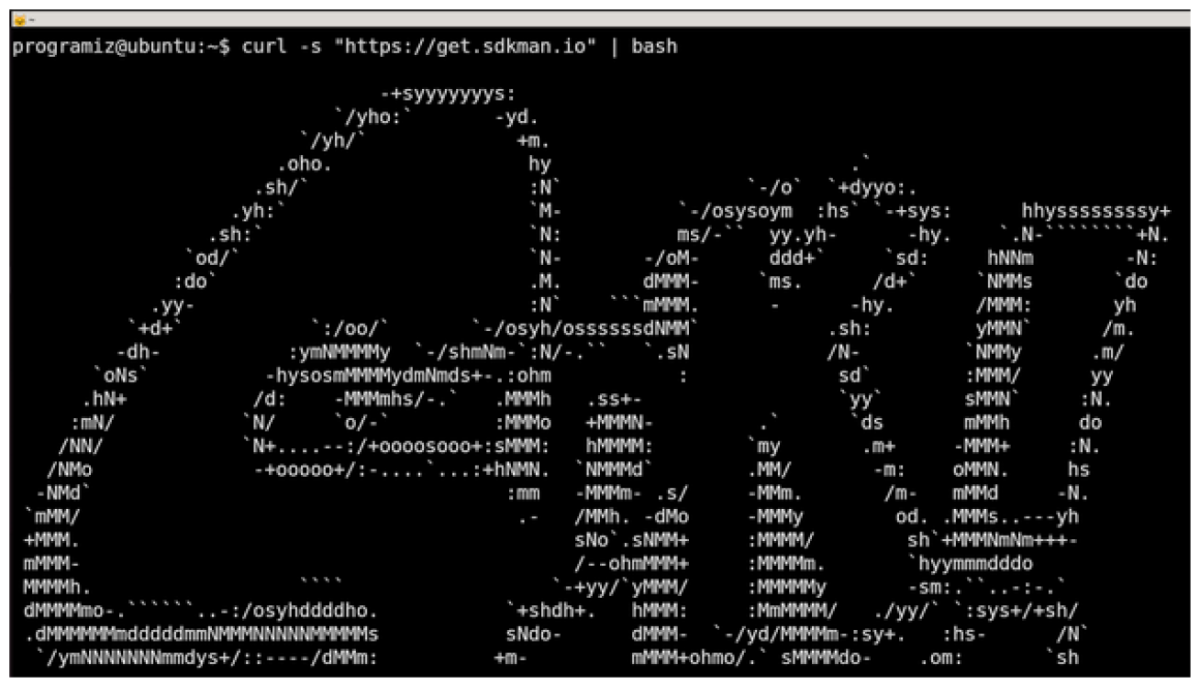
Follow the on-screen instructions to complete the installation. Afterward, open a new terminal and run the following command.
source "$HOME/.sdkman/bin/sdkman-init.sh"

Step 4: Install Kotlin
To install the Kotlin compiler, run the following command in the terminal:
sdk install kotlin
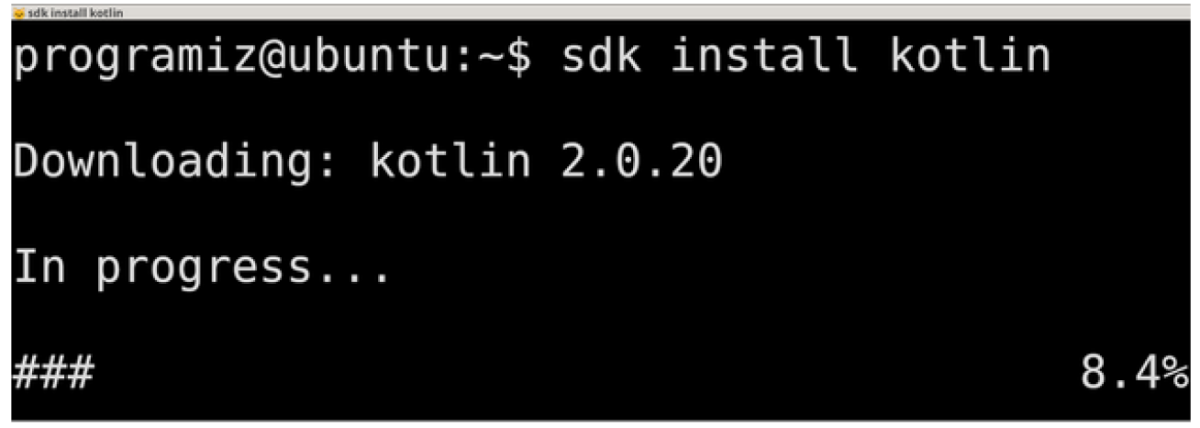
Step 5: Verify Installation
Verify the installation of Kotlin by running the following command on your terminal.
Kotlin
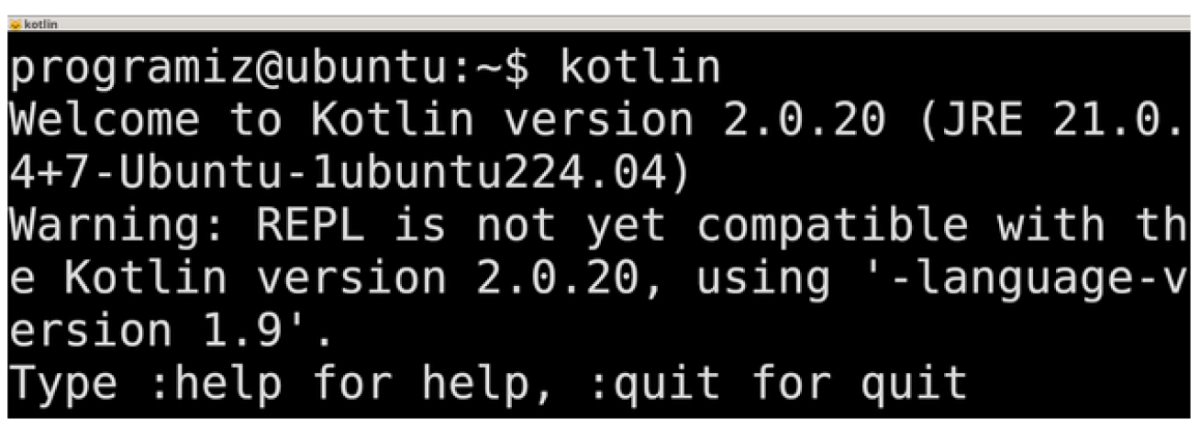
Now, you can run the Kotlin program on your device.
Run Your First Kotlin Program
You must set up a few things to run your first Kotlin program.
- Install the Code Runner and Kotlin extension in VS Code.
- Create a new file
- Write Your Kotlin Program
- Run Your Program
First, open VS Code, click on the File in the top menu and then select New File.
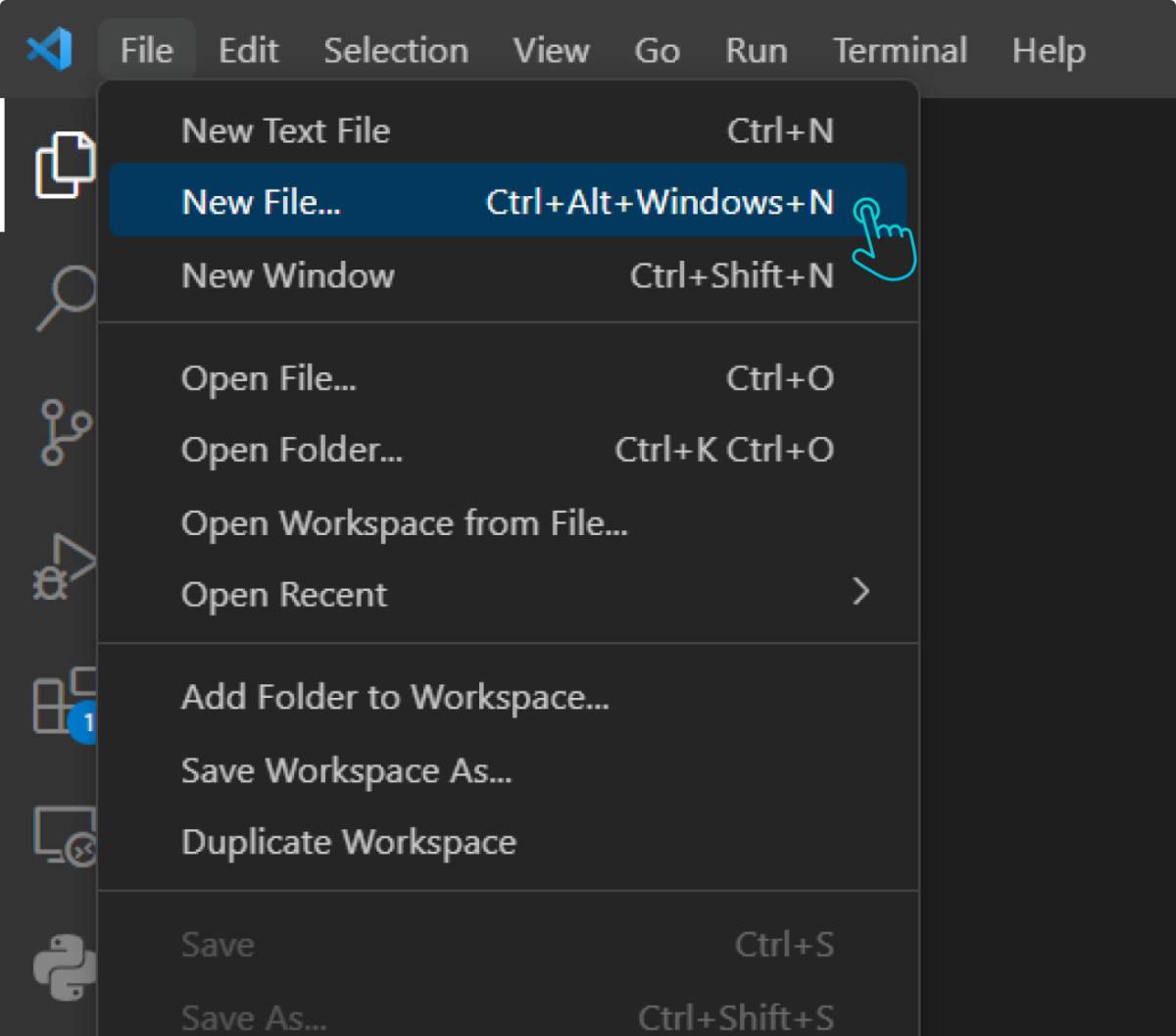
Then, save this file with a
.kt
extension by clicking on
File
again, then
Save As, and type your filename ending in
.kt
. (Here, we are saving it as
hello.kt
).
Install the Required Extension in VS Code
Before coding, ensure the Kotlin extension is installed in VS Code. Open VS Code and click on Extensions on the left sidebar. Search for the Kotlin extension and click on Install.
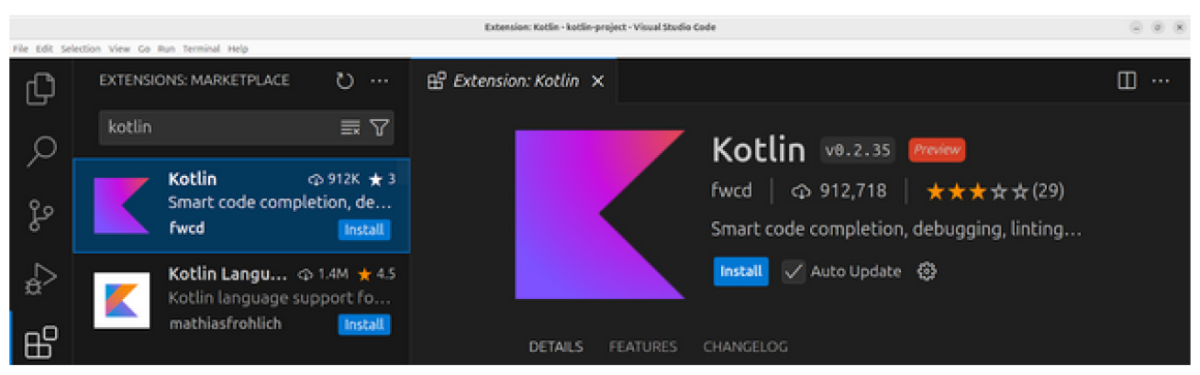
This extension provides Kotlin language support, debugging, and more, helping you develop Kotlin applications efficiently.
To run the Kotlin code directly from VS Code, we'll need the Code Runner Extension. So, follow similar steps to install the Code Runner Extension.
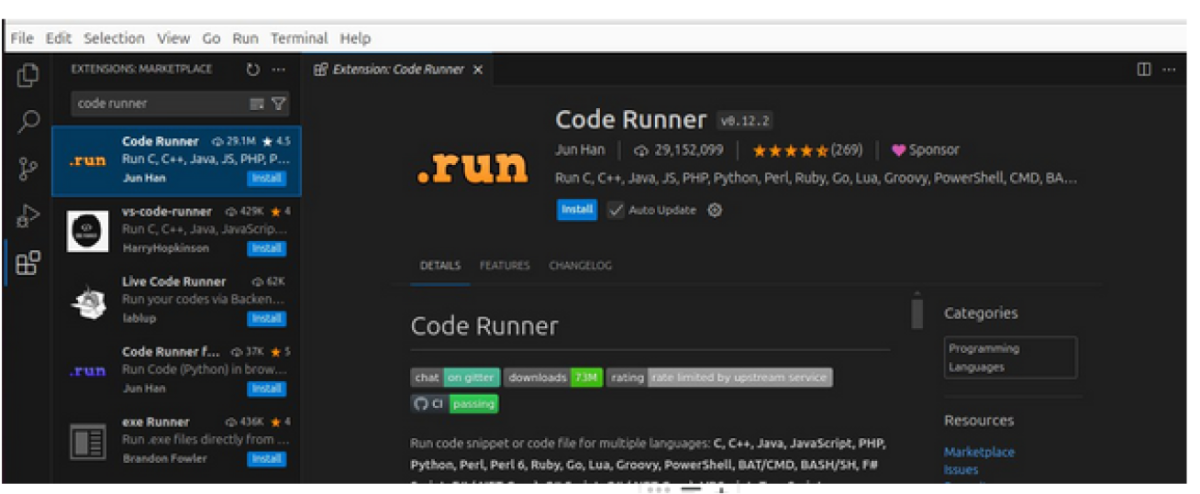
Now, write the following code into your file:
fun main() {
println("Hello, World");
}
Then click the run button on the top right side of your screen.
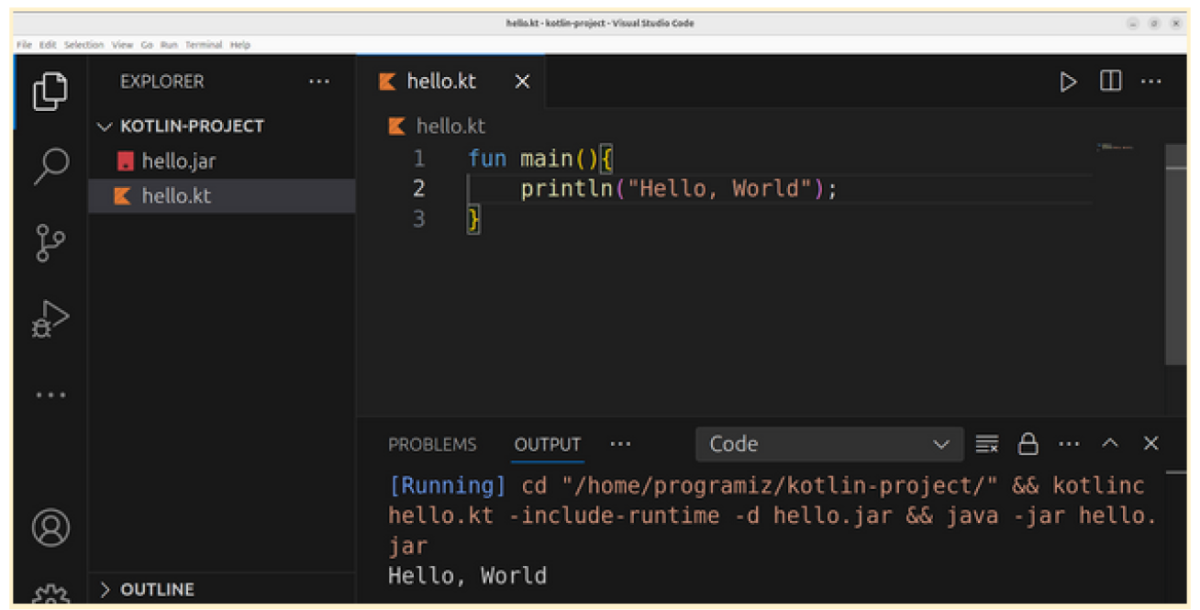
You should see
Hello, World
written on the console.
Now that you have set everything up to run a Kotlin program on your computer, you'll learn how the basic program works in Kotlin in the next tutorial.