Web design refers to the process of creating and styling the appearance of a website. There are 3 fundamental technologies to build the modern website and web applications. They are:
- HTML
- CSS
- JS
These technologies work together to provide the structure, style, and interactivity of a web page.
HTML
HTML (HyperText Markup Language) is a markup language used to structure and organize the content on a web page. It uses various tags to define the different elements on a page, such as headings, paragraphs, and links. Let's see an example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Programiz</h1>
<p>We create easy to understand programming tutorials.</p>
</body>
</html>
Browser output
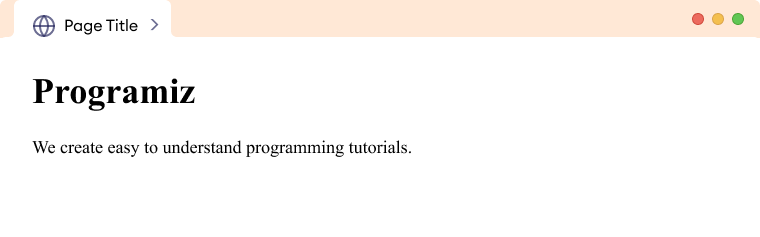
Here, we have an HTML document where:
<h1>
- heading of the document<p>
- paragraph of the document
The heading and paragraph tag in the above code help create a webpage structure.
CSS
CSS (Cascading Style Sheets) is a stylesheet language. It is used to style HTML documents.
It specifies how the elements of HTML look including their layout, colors, and fonts. We use <style>
tag to add CSS to HTML documents. Let's add some CSS to our previous HTML code.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<style>
h1 {
color: blue;
}
p {
color: red;
}
</style>
</head>
<body>
<h1>Programiz</h1>
<p>We create easy to understand programming tutorial.</p>
</body>
</html>
Browser output
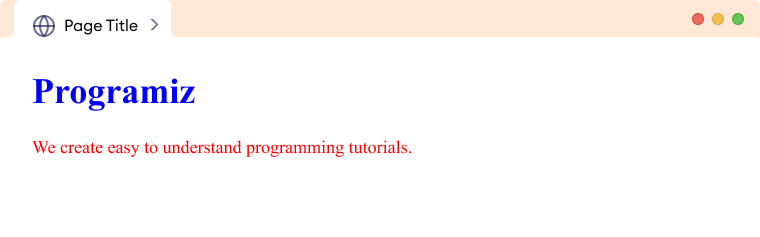
In the above code, we've applied CSS to <h1>
and <p>
tags to change their text color. Notice the code,
h1 {
color: blue;
}
This CSS code turns the text color of every <h1>
element into blue.
Similarly,
p {
color: red;
}
This CSS code turns the text color of every <p>
element into red.
JavaScript
JavaScript (JS) is a programming language that makes websites interactive and dynamic. It is primarily used in web browsers to create dropdown menus, form validation, interactive maps, and other elements on a website.
We use JavaScript with HTML and CSS to create websites that are more dynamic and user-friendly. We use <script>
tag to add JS to HTML. For example,
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<button onclick="displayAlert()">Click me!</button>
<script>
function displayAlert() {
alert("Learn coding from Programiz Pro");
}
</script>
</body>
</html>
Browser output (before button click)
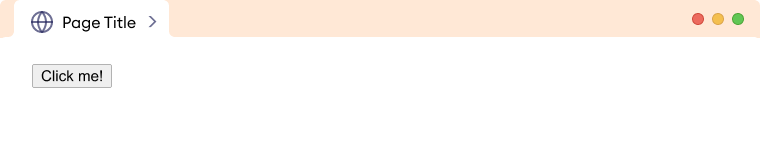
Browser output (after button click)
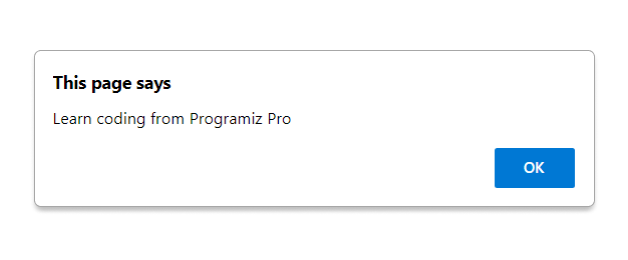
In the above example we have created a button that calls displayAlert()
when clicked.
Notice the code,
<script>
function displayAlert() {
alert("Learn coding from Programiz Pro");
}
</script>
This function simply displays an alert box with the message Learn coding from Programiz Pro
.
How do HTML, CSS, and JavaScript work?
Let us see an example of how HTML, CSS, and JS work together for a user-friendly web experience. Let's see an example,
<html>
<head>
<title>My Webpage</title>
<style>
body {
text-align: center;
}
h1 {
color: #333;
}
</style>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a simple HTML, CSS, and JavaScript webpage.</p>
<button onclick="displayAlert()">Click me!</button>
<script>
function displayAlert() {
alert('Hello World!');
}
</script>
</body>
</html>
Browser output(before button clicked)
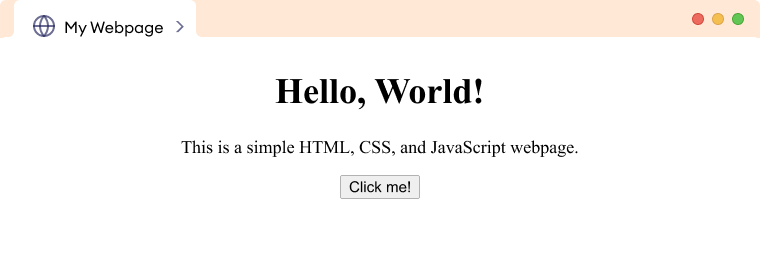
Browser output(after button clicked)
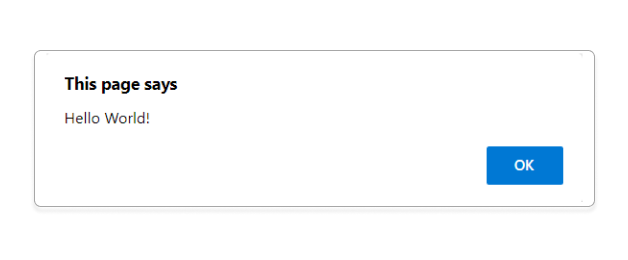
In the above example, we created a simple webpage. We can now learn more HTML, CSS, and JS to create even better websites.