TypeScript (TS) is a programming language built on top of JavaScript (JS). It essentially adds a concept of type safety that helps you catch mistakes in your code before running it.
Since TS is tightly coupled with JS, before diving into TS, it's highly recommended to have a basic understanding of JS.
Note: If you're new to JavaScript or want to refresh your skills, you can go through our JavaScript Basics course on Programiz PRO.
How TypeScript Works
Unlike JavaScript, TypeScript doesn't run directly in browsers. Instead, it's first compiled into regular JavaScript code using the TypeScript compiler. This generated JS code can then run anywhere JS works.
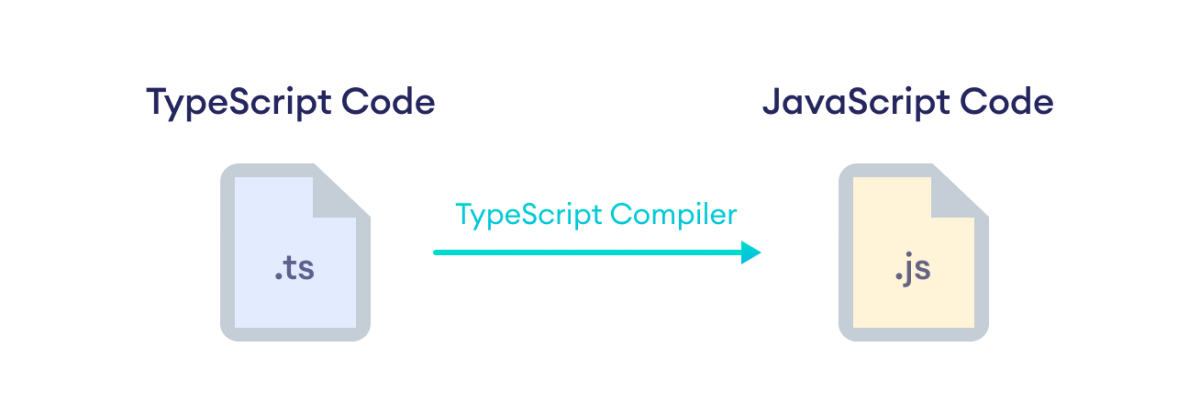
Running TypeScript
You can run TypeScript in two main ways:
- Run TypeScript Online
- Run TypeScript on Your Computer
In this tutorial, you will learn both methods of running TypeScript.
Run TypeScript Online
You can try our free online TypeScript compiler to run your TypeScript code directly in your browser, without any installation required.
If you're a beginner, we recommend you to use this approach for learning.
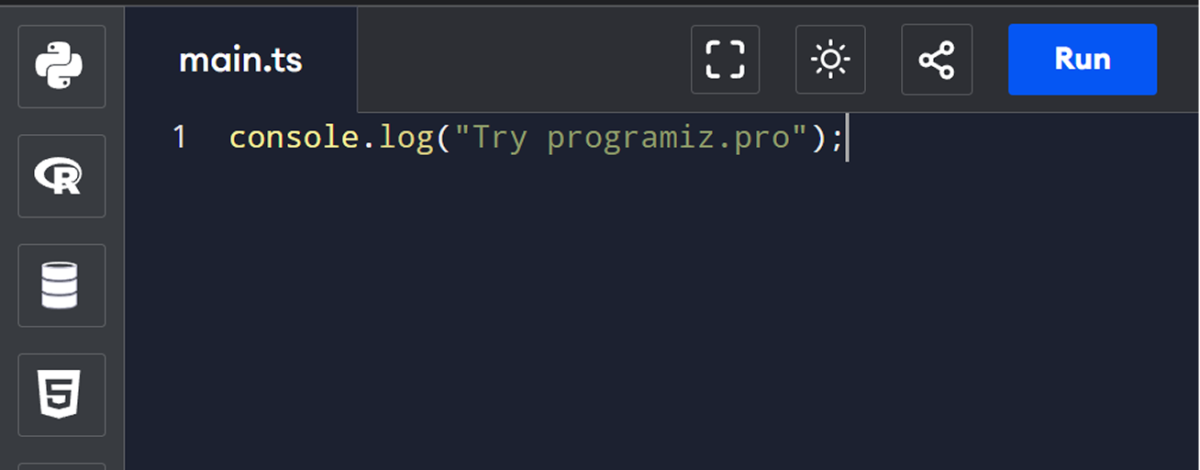
Run TypeScript on Your Computer
If you prefer to run TypeScript locally on your computer, this guide will walk you through the steps needed for Windows, macOS, or Linux (Ubuntu).
You can switch the tabs below for specific OS implementation.
Install TypeScript on Windows
To run TypeScript locally on your computer, follow these steps:
1. Install Visual Studio Code
2. Download and Install Node.js
3. Install TypeScript
4. Verify Your Installation
Step 1: Install Visual Studio Code
Go to the VS Code Official website and download the Windows installer. Once the download is complete, run the installer and follow the installation process.
Click Finish to complete the installation process.
Step 2: Download and Install Node.js
First, go to the official Node.js website and download the LTS version (which is the recommended version for most users).
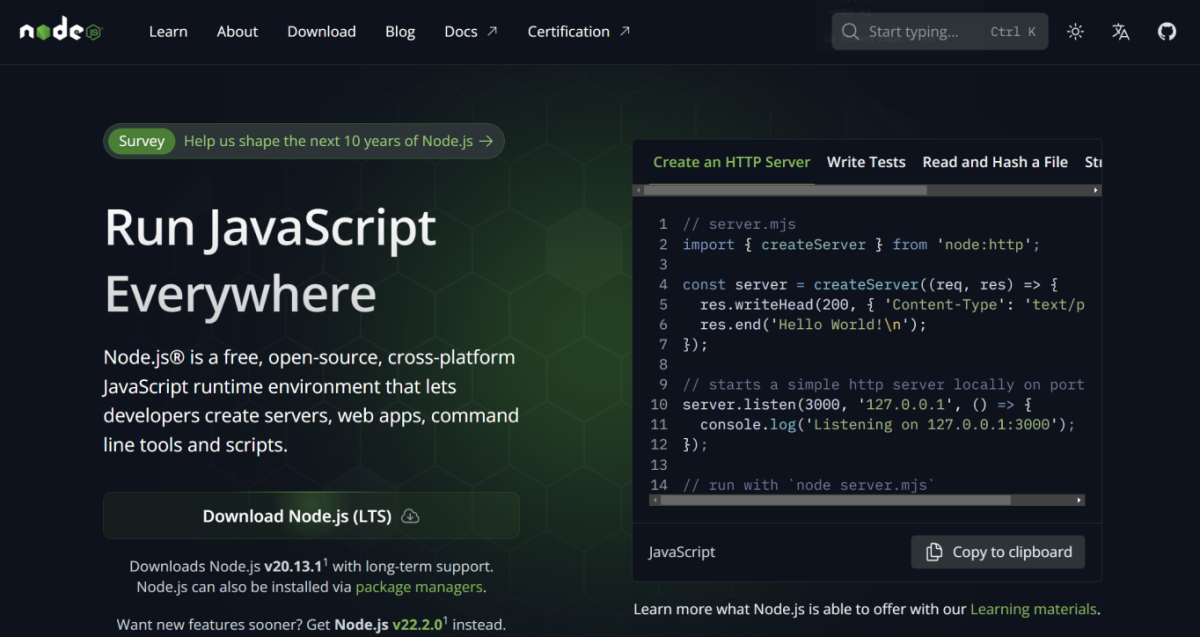
After the download is complete, go to your Downloads folder and double-click the .msi file you just downloaded to start the installation. You may be prompted to allow changes. Click Next to proceed.
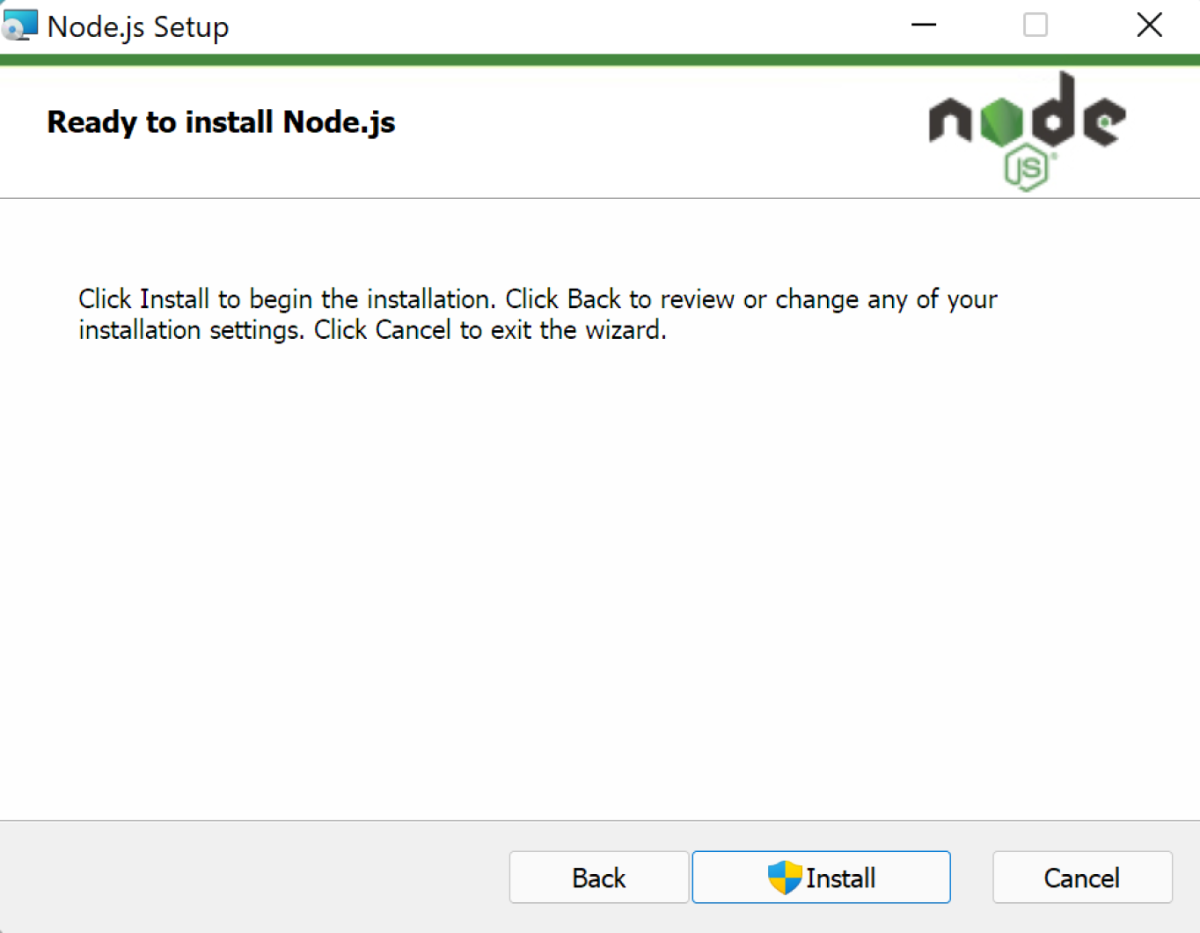
Now, just click the Install button to begin installing. After a few moments, Node.js will be installed and ready to use on your device.
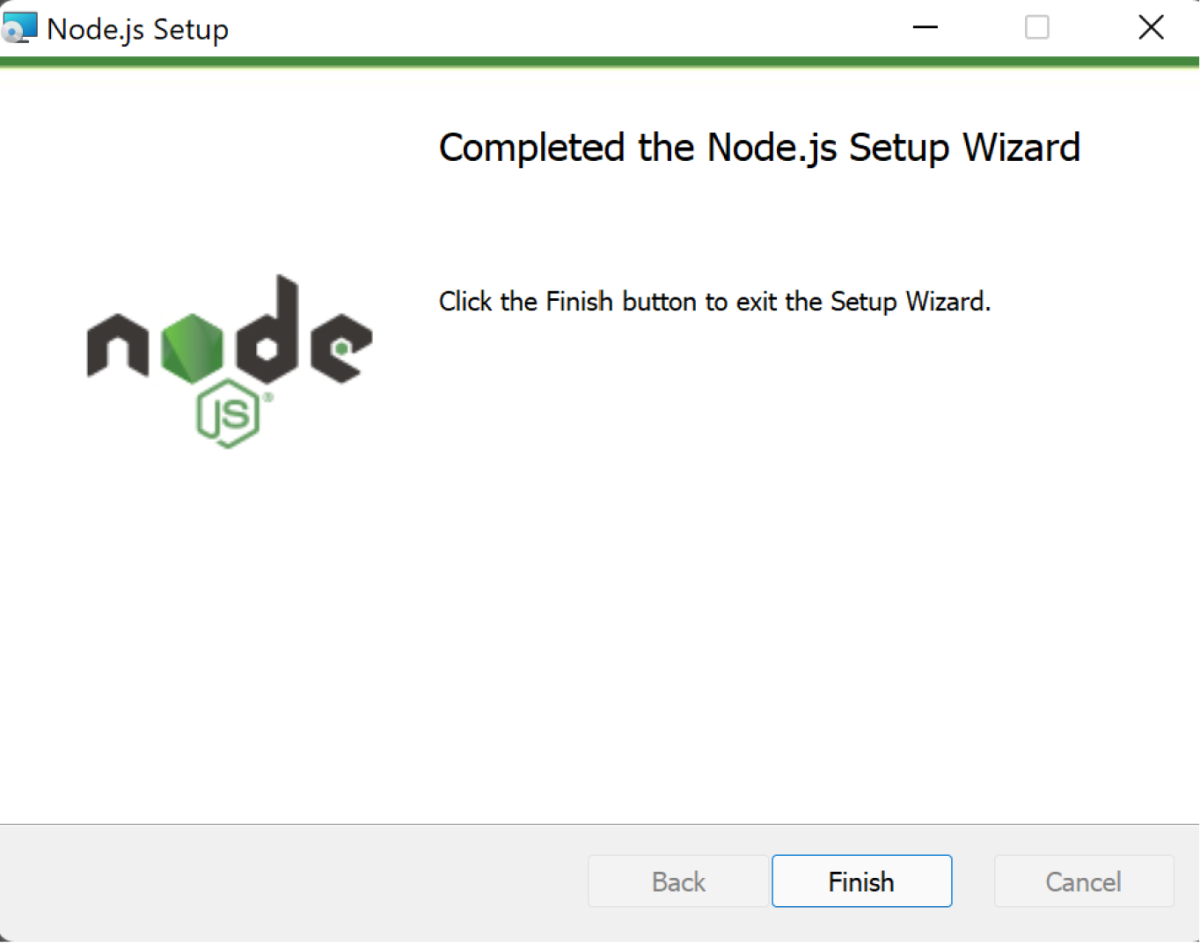
Step 3: Install TypeScript
Once Node.js is installed, open your terminal or command prompt and run the following command to install TypeScript globally:
npm install -g typescript
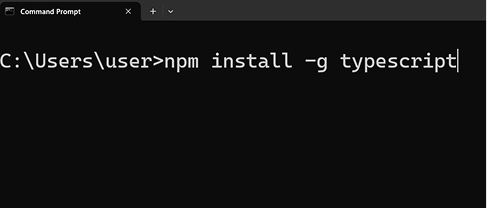
The -g
flag installs TypeScript globally so it's accessible from anywhere.
Step 4: Verify Your Installation
After installation, you can verify that TypeScript is installed correctly by running the following command:
tsc --version
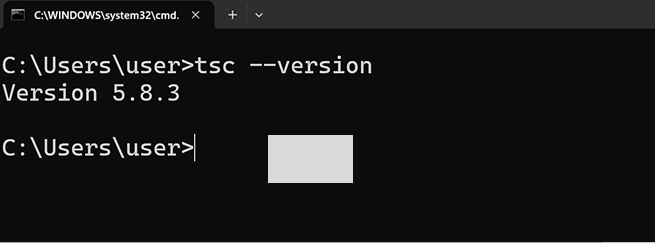
Note: The version number may vary depending on the version you have installed.
Now, you're all set to start running TypeScript programs on your device.
Install TypeScript on Mac
To run TypeScript on your Mac OS, follow these steps:
- Install VS Code
- Download and install Node.js
- Install TypeScript
- Verify your Installation
Step 1: Install Visual Studio Code
Go to the VS Code Official website and download the Mac installer. Once the download is complete, open the .dmg file and drag Visual Studio Code to your Applications folder.
Step 2: Download the Node.js File
First, go to the official Node JS website and download the LTS version, which is the recommended version for the majority of the users.
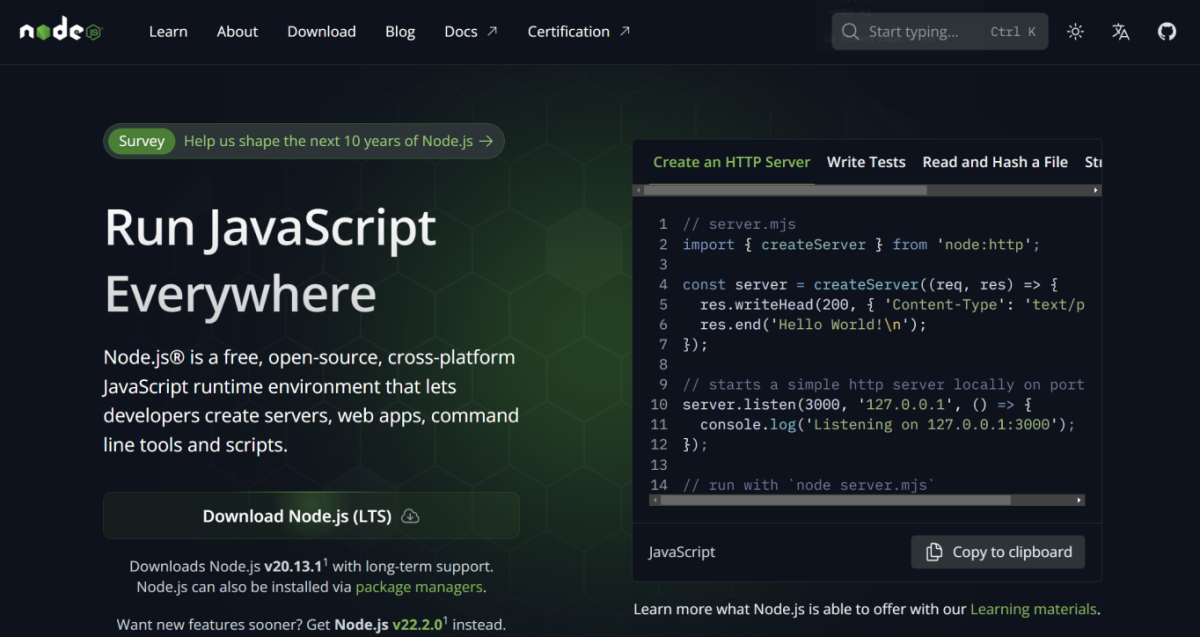
After the download is complete, open the .pkg file and follow the on-screen instructions to install Node.js. The installation process will guide you through each step; just follow the prompts and accept the license agreement.
Simply allow the installation and continue.
Then you will come across the installation part.
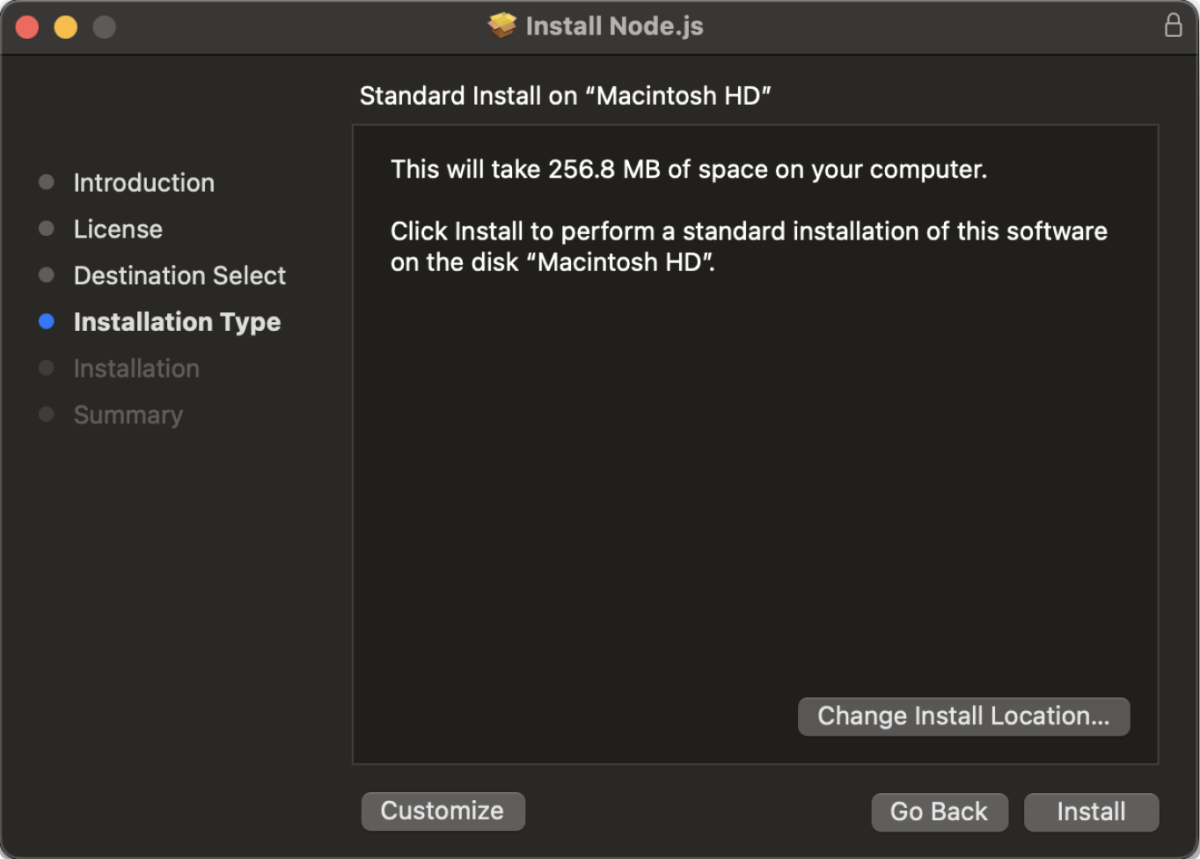
Now, just click the Install button to begin installing. After a few moments, Node will be successfully installed on your device.
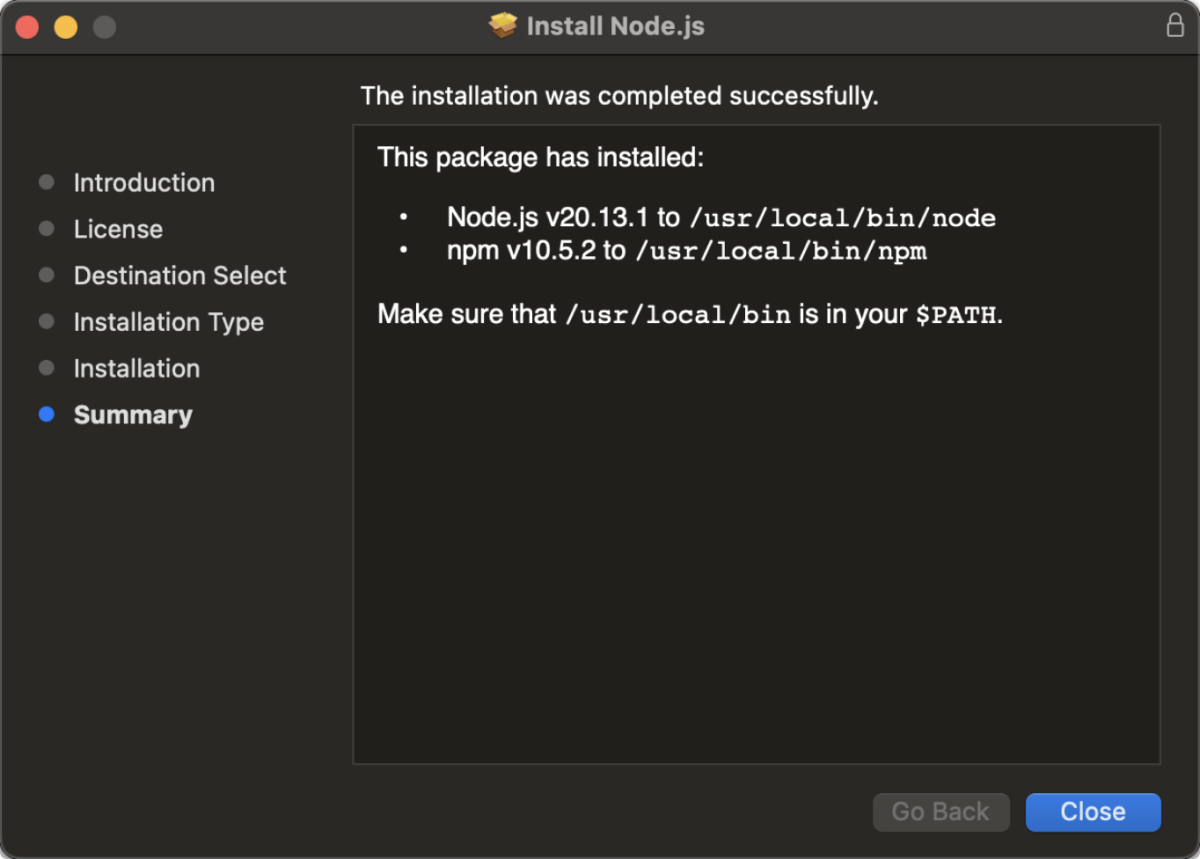
Step 3: Install TypeScript
Once Node.js is installed, open your terminal or command prompt and run the following command to install TypeScript globally:
npm install -g typescript

Here, -g
flag ensures that TypeScript is installed globally, making it accessible from
anywhere on your system.
Step 4: Verify Your Installation
After installation, you can verify that TypeScript is installed correctly by running the following command:
tsc --version
or
tsc -v
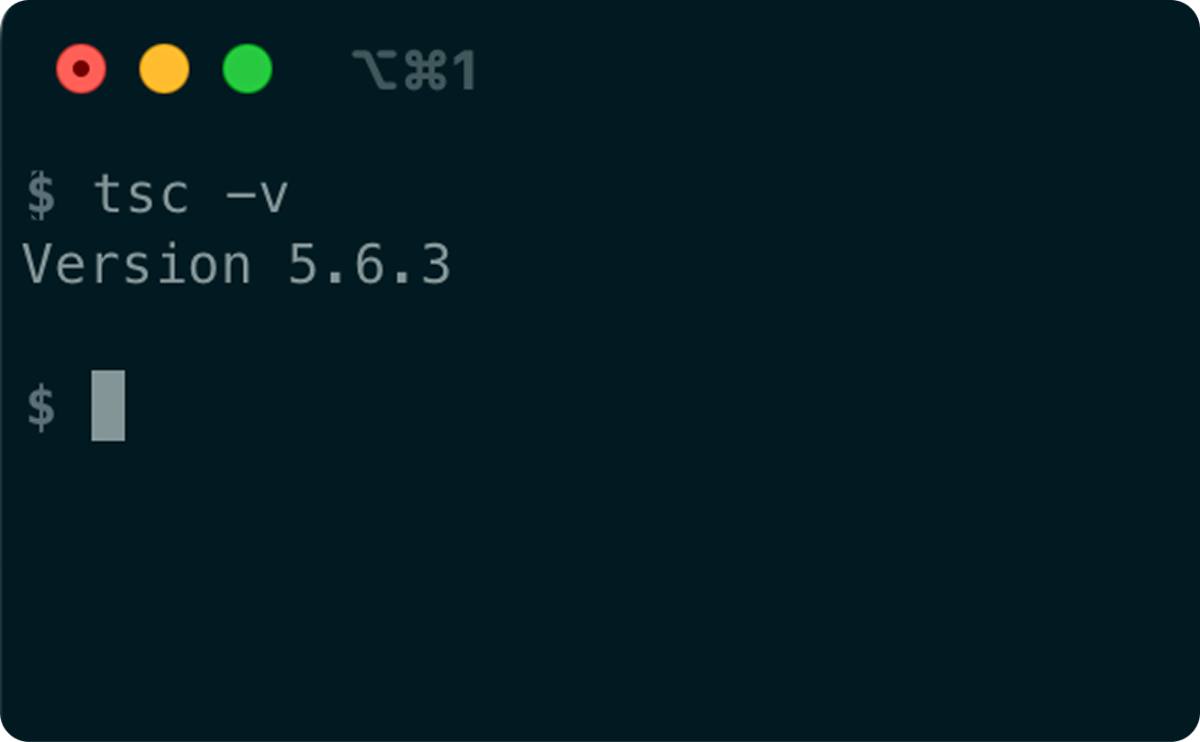
Note: The version number may vary depending on the version you have installed.
Now, you're all set to start running TypeScript programs on your device.
Install TypeScript on Linux
Linux has several distributions, each with slightly different installation processes. The following steps are specifically for the Ubuntu distribution.
- Install VS Code
- Install Node
- Install TypeScript
- Verify Your Installation
Here is a detailed explanation of each of the steps:
Step 1: Install VS Code
Open the Terminal and type
sudo apt update
This command updates your package lists to ensure you get the latest versions of your software.
Proceed to install VS Code with
sudo snap install code --classic
Step 2: Install Node
Go to snapcraft and click install.
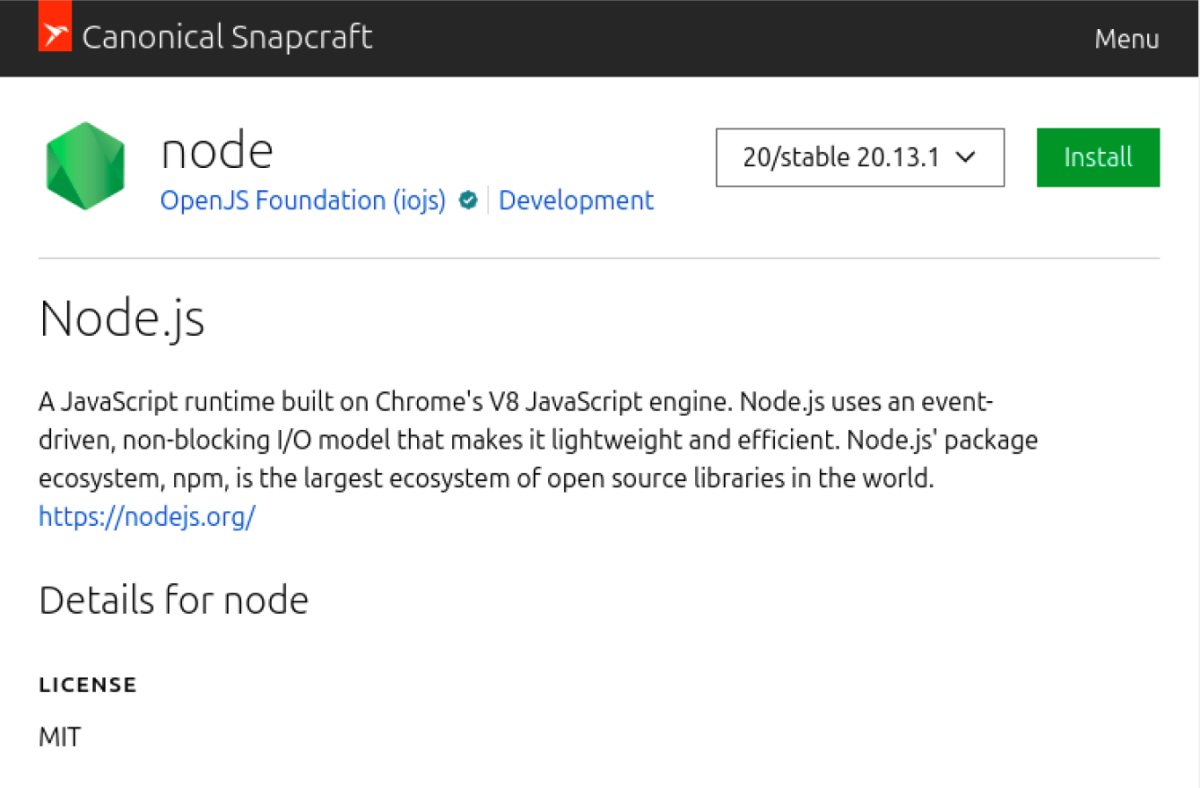
It will give you the command to install Node.
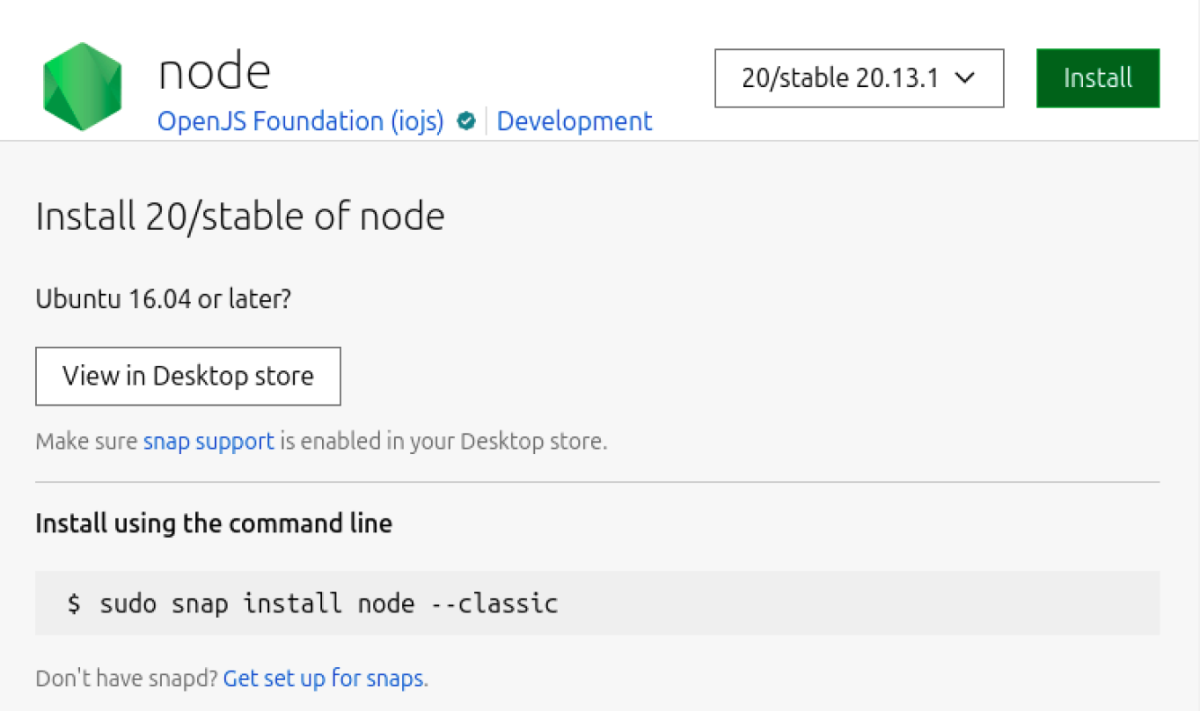
Open your Terminal and paste the command to install Node on your device.
sudo snap install node --classic

Wait until the installation finishes to start using Node.
Step 3: Install TypeScript
Once Node.js and npm are installed, you can install TypeScript globally by running the following command in your terminal:
sudo npm install -g typescript

The -g
flag ensures that TypeScript is installed globally, allowing you to use it from
anywhere on your system.
Step 4: Verify Your Installation
To verify that TypeScript has been installed correctly, run the following command:
tsc --version
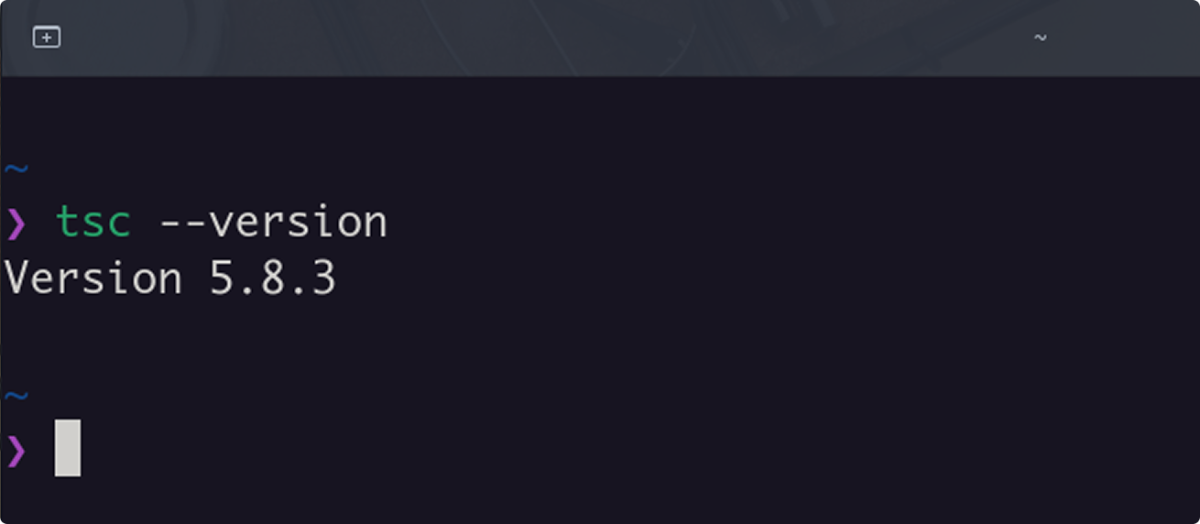
This will display the installed version of TypeScript. Note that the version number may vary depending on the version you installed.
Now, you're all set to start running TypeScript programs on your Ubuntu device.
Run Your First Program
1. Open VS Code
First, open Visual Studio Code. Click on File in the top menu and then select New File.
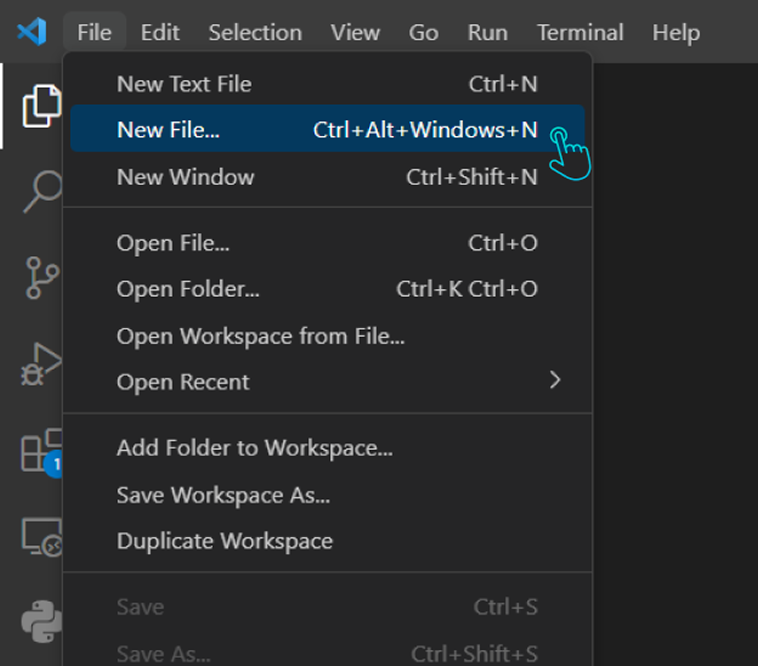
2. Save the File
Next, save the file with a .ts
extension. Click on File, then Save
As, and save the file as helloWorld.ts
.
3. Write Your Code
Now, write the following code in your helloWorld.ts
file:
console.log("Hello, TypeScript!");
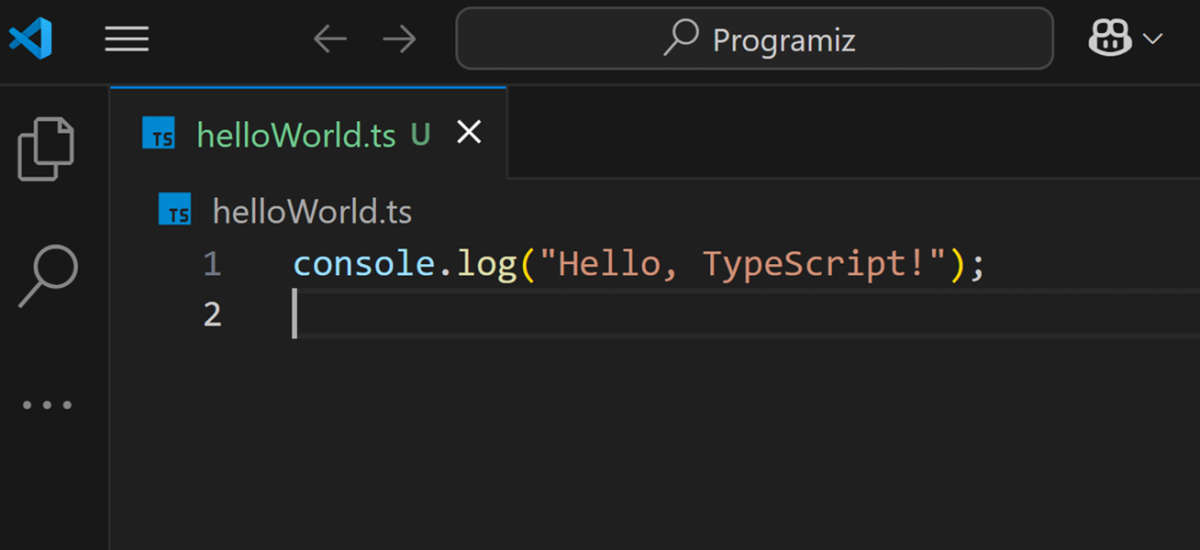
5. Compile and Run Your Code
To compile your TypeScript code into JavaScript, open the VS Code terminal by selecting Terminal > New Terminal from the top menu. Then, run the following command:
tsc helloWorld.ts
This will compile your helloWorld.ts
file into a helloWorld.js
file.
Once compiled, you can see the helloWorld.js
file has been created. To run it, type the following
command in the same terminal:
node helloWorld.js
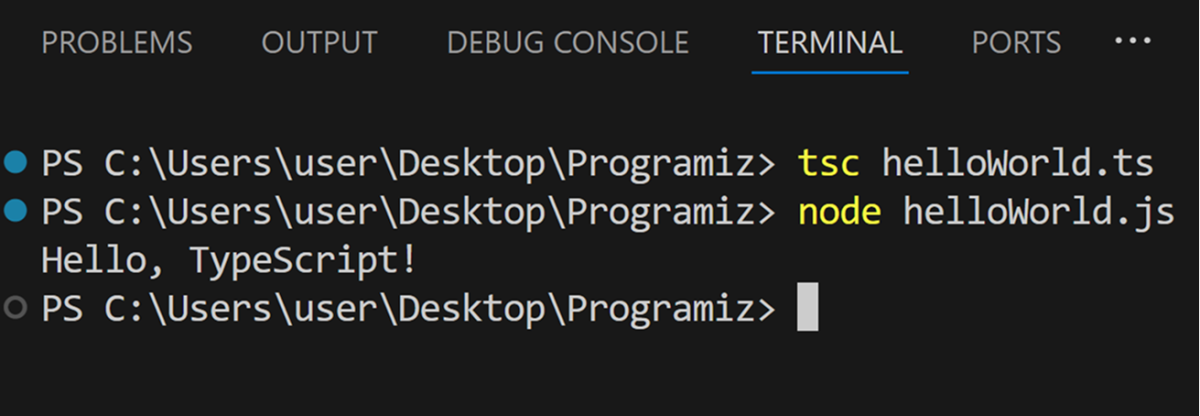
You should now see "Hello, TypeScript!"
printed in the output console.
Alternative Way to Run Your Program
To make the development process faster and run TypeScript files directly without manually compiling them to JavaScript every time, you can install Code Runner in VS Code.
1. Install Code Runner Extension
To install the Code Runner extension, open VS Code, click on the Extensions icon in the left sidebar, then search for Code Runner and click Install.
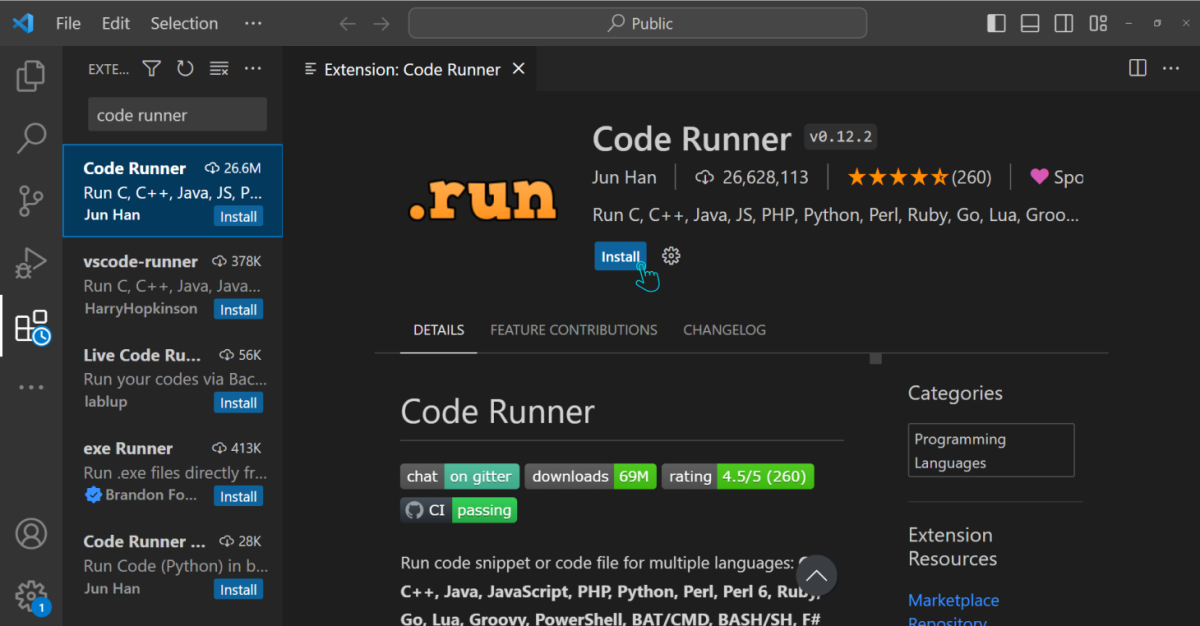
2. Install TypeScript dependency for Code Runner
For TS to work on VS code, you need to install ts-node
package. Similarly to installing
typescript
, to install ts-node
, open the VS Code terminal and run the following
command:
npm install -g ts-node
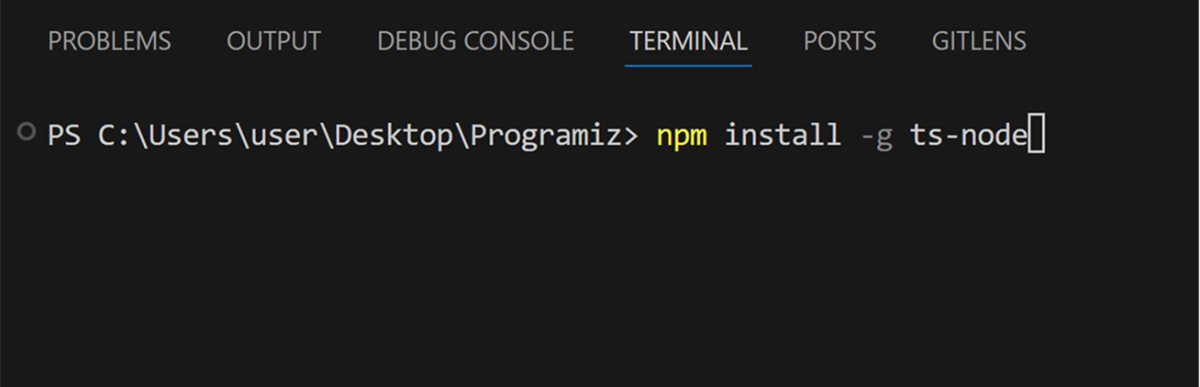
You can now directly run your TypeScript file. To do this, open the TS file, you should see a Run (Play) button to execute it in VS Code.
Now that you've set up everything to run TypeScript programs on your computer, you'll learn how to work with basic TypeScript features in the next tutorial.