The match…case
statement allows us to execute different actions based on the value of an expression.
The syntax of the match...case
statement in Python is:
match expression:
case value1:
# code block 1
case value2:
# code block 2
...
Here, expression
is a value or a condition to be evaluated.
If expression
is equal to
value1
, thecode block 1
is executed.value2
, thecode block 2
is executed.
In a match
statement, only one of the options will be executed. Once a match is found, the corresponding block of code runs, and the rest are skipped.
Note: The match..case
statement was introduced in Python 3.10 and doesn't work on older versions. It is similar to the switch…case
statement in other programming languages such as C++ and Java.
Now, let's look at a few examples of the match..case
statement.
Example 1: Python match...case Statement
operator = input("Enter an operator: ")
x = 5
y = 10
match operator:
case '+':
result = x + y
case '-':
result = x - y
case '*':
result = x * y
case '/':
result = x / y
print(result)
Output 1
Enter an operator: * 50
The operator
variable stores the input from the user, which represents a mathematical operator. The match
statement evaluates the value of operator
and runs the corresponding code block.
If operator
is:
+
:result = x + y
is executed.-
:result = x - y
is executed.*
:result = x * y
is executed./
:result = x / y
is executed.
While this program works for inputs +, -, *, and /, it will result in an error if we enter any other character as an operator.
Output 2
Enter an operator: % ERROR! NameError: name 'result' is not defined
We are getting this error because the input value (%
) doesn't match with any cases.
To solve this problem, we can use the default case.
The default case
We use the default case, which is executed if none of the cases are matched. Here's how we use a default case:
match expression:
case value1:
....
case value2:
...
case _: # default case
....
Here, _
represents the default case.
Let's solve the above error using the default case.
Example 2: The default case
operator = input("Enter an operator: ")
x = 5
y = 10
match operator:
case '+':
result = x + y
case '-':
result = x - y
case '*':
result = x * y
case '/':
result = x / y
case _:
result = "Unsupported operator"
print(result)
Output
Enter an operator: % Unsupported operator
Understanding Python match...case with Flowchart
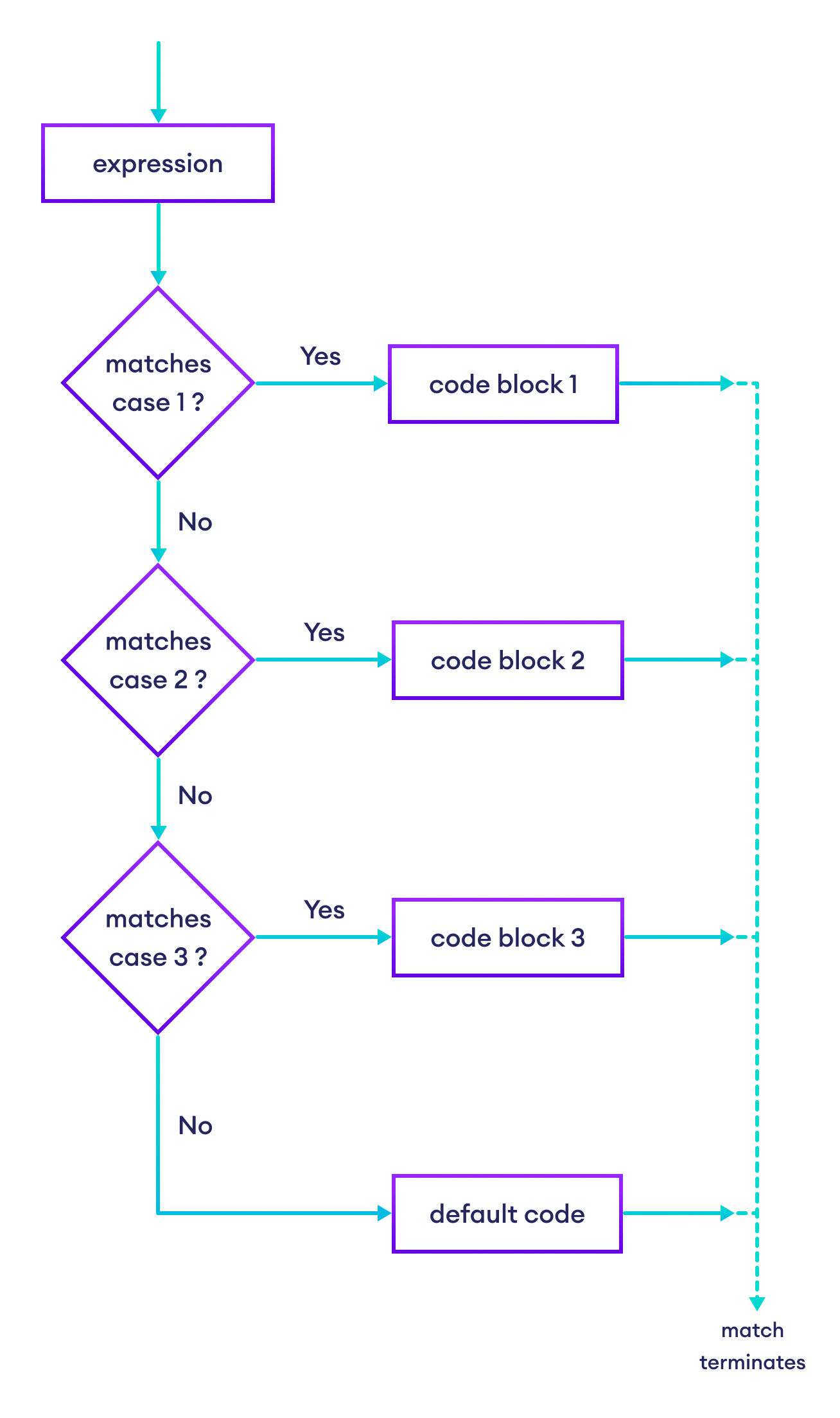
Using match...case with | Operator
The match...case
statement in Python is very flexible. For an instance, it is possible to match an expression against multiple values in case clauses using the |
operator.
For example,
status = int(input("Enter the status code: "))
match status:
case 200 | 201 | 202:
print("Success")
case 400 | 401 | 403:
print("Client error")
case 500 | 501 | 502:
print("Server error")
case _:
print("Unknown status")
Output 1
Enter the status code: 500 Server error
Output 2
Enter the status code: 700 Unknown status
In each case, the |
operator is used to match against multiple values. If the status
value matches any of the values in a certain case, the code within that case will execute:
200 | 201 | 202
: Prints"Success"
if status is 200, 201, or 202.400 | 401 | 403
: Prints"Client error"
if status is 400, 401, or 403.500 | 501 | 502
: Prints"Server error"
if status is 500, 501, or 502.
Using Python if statement in Cases
In Python, we can also use the if
statement in the case clauses. This is called guard, which adds an additional condition to an expression.
If the guard condition (the if
statement) evaluates to False
, the match
statement skips that case and continues to the next one.
For example,
subject = input("Enter a subject: ")
score = int(input("Enter a score: "))
match subject:
# if score is 80 or higher in Physics or Chemistry
case 'Physics' | 'Chemistry' if score >= 80:
print("Excellent in Science!")
# if score is 80 or higher in English or Grammar
case 'English' | 'Grammar' if score >= 80:
print("Excellent in English!")
# if score is 80 or higher in Maths
case 'Maths' if score >= 80:
print("Excellent in Maths!")
case _:
print(f"Needs improvement in {subject}!")
Output1
Enter a subject: Chemistry Enter a score: 95 Excellent in Science!
Here, case 'Physics' | 'Chemistry' if score >= 80:
is executed because the subject
matches "Chemistry"
and the score
95 satisfies the if
condition (score >= 80
).
In case clauses, we used if
statement to add an additional condition. Here, it checks whether the score
is 80 or higher.
Note: When we use an if
statement in cases, the if
condition is evaluated only after a case match is found.